CSS variables, also called CSS custom properties, are a useful functionality in contemporary CSS that permits you to establish a value once and reuse it multiple times in your stylesheet.
This can aid in decreasing redundancy in your code, enhancing consistency, and simplifying maintenance and updating of your styles over time. CSS variables can be employed to define various values, including colors, font sizes, gradients, and box shadows.
Despite CSS variables providing numerous benefits, it is necessary to consider their constraints and potential performance concerns, particularly when combined with JavaScript.
- What are CSS Variables
- How to Get the Value of a CSS Variable using JavaScript
- How to Set the Value of a CSS Variable using JavaScript
- CSS Variable Best Practices
What are CSS Variables
CSS variables, also referred to as CSS custom properties, are variables in Cascading Style Sheets (CSS) that enable you to define and reuse values throughout your CSS stylesheets.
Let's say you want to define a variable for the background color of your website. Instead of hard-coding a color value into your CSS, you can define a CSS variable like this:
:root {
--bg-color: #84d7f1;
}
Then, you can use the var()
function to reference this variable wherever you need to set a background color:
body {
background-color: var(--bg-color);
}
.header {
background-color: var(--bg-color);
}
.footer {
background-color: var(--bg-color);
}
Now, if you want to change the background color of your entire website, you only need to update the value of the --bg-color
variable in one place, rather than updating each individual element's background-color property. This makes it easier to maintain consistency allround your website and update the design as needed.
Why CSS variables are useful?
CSS variables offer several advantages, including flexibility and readability. By defining a value once and using it multiple times throughout your stylesheet, you can make changes to your design in one place and create more consistent styles across your website.
Additionally, using descriptive variable names can improve the readability and maintainability of your code, especially in larger projects. CSS variables also allow for dynamic styling using JavaScript, making it possible to create interactive and responsive designs.
Furthermore, CSS variables are useful for creating themes or styles that can be easily switched out, enabling you to define variables for colors, fonts, spacing, and other elements to quickly apply different themes or styles to your website.
How to Get the Value of a CSS Variable using JavaScript?
If you want to retrieve the value of a CSS variable using JavaScript, you can use the getComputedStyle()
method. This method returns the computed style of an element, which includes the value of any CSS variable specified in its styles.
Here's an example of how to use getComputedStyle()
to retrieve the value of a CSS variable named --primary-color
:
// Define the CSS variable
:root {
--primary-color: green;
}
// Get the value of the CSS variable using JavaScript
const rootStyles = getComputedStyle(document.documentElement);
const primaryColor = rootStyles.getPropertyValue('--primary-color');
// Log the value of the CSS variable
console.log(primaryColor); // Outputs 'green'
In the example, we first define the CSS variable --primary-color
within the :root
pseudo-class. Then, we use getComputedStyle()
to obtain the computed style of the documentElement
(i.e., the html
element) and store it in a variable called rootStyles
. We can then utilize the getPropertyValue()
method on rootStyles to extract the value of the --primary-color
variable and assign it to a variable called primaryColor
.
Finally, we print the value of the --primary-color
variable to the console, which outputs 'green'
.
It's important to note that the value returned by getPropertyValue()
is a string that contains any units specified in the CSS variable value. For example, if the value of --primary-color
is 10px
, getPropertyValue('--primary-color')
would return the string '10px'
. To use the value of a CSS variable for calculations, you may need to extract the unit using a JavaScript function like parseInt()
or parseFloat()
.
Getting CSS Variable Values in Different Scenarios
#1 Text
CSS variables can also be helpful for making websites more accessible by allowing the definition of text size and color values that meet accessibility standards. By accessing these variable values, the text style can be dynamically adjusted based on the user's needs or preferences.
Example:
:root {
--font-size-small: 11px;
--font-size-medium: 15px;
--font-size-large: 21px;
--text-color-primary: #f47eb6;
--text-color-secondary: #ef3f92;
--text-color-tertiary: #d8126f;
}
body {
font-size: var(--font-size-small);
color: var(--text-color-primary);
}
h1 {
font-size: var(--font-size-large);
color: var(--text-color-secondary);
}
h2 {
font-size: var(--font-size-medium);
color: var(--text-color-tertiary);
}
The usage of CSS variables to set font sizes and colors for various page elements like body
, h1
, and h2
. A media query is also applied to adjust the font size for body on smaller screens.
The benefit of using CSS variables is that they make it easy to modify the font sizes and colors without altering the code, enabling developers to adhere to accessibility standards or user preferences effortlessly.
#2 Dynamic theme
Dynamic theming can be achieved by defining CSS variables to store values for various styles, such as color, font-size, padding, etc., for multiple themes on a website. Accessing the values of these variables through JavaScript enables dynamic switching between themes without needing to modify style values in the code.
:root {
--primary-color: #ff407f;
--secondary-color: #ff95b8;
--background-color: #fff;
}
body {
background-color: var(--background-color);
color: var(--primary-color);
}
button {
background-color: var(--secondary-color);
color: var(--background-color);
}
.dark-mode {
--primary-color: #fff;
--secondary-color: #ff407f;
--background-color: #0e1011;
}
The definition of three CSS variables to set the primary, secondary, and background colors of elements on a webpage.
A .dark-mode
class is also defined to change the values of these CSS variables, thus creating a dark theme. By adding or removing the .dark-mode
class on the HTML element through JavaScript, dynamic switching between themes is possible without changing style values in the code.
#3 Conditional Styles
To apply conditional styles to elements on a webpage, CSS variables can be combined with JavaScript. By retrieving the value of a CSS variable, you can compare it to a condition and apply a specific style to the element.
:root {
--color-primary: grey;
--color-secondary: pink;
}
.conditional-styles {
background-color: var(--color-primary);
padding: 5px;
color: white;
}
const element = document.querySelector('.conditional-styles');
const color = getComputedStyle(document.documentElement).getPropertyValue('--color-primary');
if (color === 'blue') {
element.style.backgroundColor = 'var(--color-secondary)';
}
The two CSS variables for primary and secondary colors and applies the primary color to a class named .conditional-styles
. Using JavaScript, the value of the variable --color-primary
is retrieved and checked against a condition. If the color is blue, the background color of the element is set to the secondary color.
#4 Media Queries and Breakpoints
To create a responsive website, CSS variables can be utilized to specify media queries and breakpoints. By obtaining the values of these variables, the website's layout can be adjusted dynamically according to the screen size.
:root {
--breakpoint-small: 750px;
--breakpoint-medium: 900px;
--breakpoint-large: 1200px;
}
/* Styles for small screens */
@media (max-width: var(--breakpoint-small))
/* Styles for medium screens */
@media (min-width: var(--breakpoint-medium))
/* Styles for large screens */
@media (min-width: var(--breakpoint-large))
This code demonstrates the use of CSS variables to set breakpoints for responsive web design. The variables are utilized in media queries to apply styles based on screen sizes. By adjusting the values of these variables, the website's layout can be easily optimized for various devices and screen sizes.
#5 Animation Effects
CSS variables provide the flexibility to define animation properties such as duration, delay, and timing functions. With JavaScript, the values of these variables can be obtained to dynamically adjust the animation effects of an element.
:root {
--animation-duration: 2s;
--animation-delay: 0.5s;
--animation-timing-function: ease;
}
.box {
animation-duration: var(--animation-duration);
animation-delay: var(--animation-delay);
animation-timing-function: var(--animation-timing-function);
animation-name: slide-in;
}
@keyframes slide-in {
from {
transform: translateX(-100%);
}
to {
transform: translateX(0);
}
}
In this example, we define three CSS variables for animation duration, delay, and timing function, which are used to set the values for the animation properties of a .box
element. We also define a keyframe animation that fades in the element. By accessing the values of these variables through JavaScript, we can dynamically adjust the animation effects of the element.
Common CSS Variable Values Issues and Solution with JavaScript
-
Browser compatibility is a potential issue as CSS variables may not be supported by all browsers, especially older versions. This could lead to difficulties in obtaining the value of a CSS variable using JavaScript since the browser may not recognize the variable name. To mitigate this issue, fallback values may be provided, or a polyfill can be used to ensure that CSS variables are supported across all browsers.
Solution: To address this potential issue, fallback values can be provided so that if the browser does not recognize the variable, a default value can be used instead. Another option is to use a polyfill, which is a JavaScript script that emulates the behavior of CSS variables, ensuring that they are supported across all browsers.
-
Scope limitations due to their scoping rules, CSS variables can only be accessed within the element where they are defined. If a variable is defined in a child element, it cannot be accessed in the parent element or other sibling elements. This can create challenges when attempting to retrieve the value of a CSS variable using JavaScript, particularly if the variable's scope is not properly understood. To overcome this challenge, you may need to define the variable in a higher-level element or use alternative methods like custom data attributes.
Solution: To address this issue, you could define the variable in a higher-level element or use alternative methods like custom data attributes. Another possible solution is to use CSS preprocessors such as Sass, which provide greater flexibility in variable scoping.
-
Timing challenges is crucial to make sure that CSS styles have been applied to an element before attempting to access the value of a CSS variable using JavaScript. This can be challenging when styles are being applied dynamically or asynchronously. To overcome this challenge, you may need to use a callback or event listener to ensure that styles have been applied before trying to access the variable.
Solution: One possible solution is to use a callback or event listener to ensure that the styles have been applied before accessing the variable. By doing so, errors can be prevented, and the code can run smoothly.
-
Errors in syntax defining or using CSS variables with incorrect syntax can lead to issues, where the variable may not be recognized or used properly. These issues can be challenging, especially if the error is difficult to diagnose or identify. To overcome this challenge, it is essential to thoroughly review your code and leverage debugging tools to identify and fix any syntax errors.
Solution: To tackle this challenge, it is crucial to review your code thoroughly and use debugging tools to catch and fix any syntax errors. By doing so, you can make sure that your CSS variables are used correctly, preventing any potential issues with your code.
How to Set the Value of a CSS Variable using JavaScript?
You can use the setProperty()
method on the style
object of an element in JavaScript to assign a value to a CSS variable. This method requires two arguments: the name of the CSS variable that you want to set and the value that you want to assign to it.
// Select the element you want to set the CSS variable on
const element = document.querySelector('.my-element');
// Set the value of the CSS variable using setProperty()
element.style.setProperty('--my-variable', 'pink');
We have targeted an element with the class name "my-element"
and assign the value of the CSS variable "--my-variable"
to the color pink
. The notation of double dashes (--) specifies that "--my-variable"
is a CSS variable.
Upon setting the value of a CSS variable, it will apply to all the elements that incorporate that variable in their styles. Furthermore, with JavaScript, you can modify the value of a CSS variable dynamically. This enables you to create dynamic theming or adjust other styles based on user interactions or other events.
CSS Variable Best Practices
- Avoid using CSS variables for styles that are unlikely to change, as this can add unnecessary complexity to your code.
- Test your code thoroughly across different browsers and devices to ensure compatibility and avoid potential issues with browser support or syntax errors.
- Use fallback values to ensure that your code works on older browsers or in case the variable is not defined, provide fallback values using CSS or JavaScript. For example, you can set a default color or size for an element if the variable is not available.
- Use descriptive variable names when defining CSS variables, use descriptive names that clearly convey their purpose. This will make it easier to understand and maintain your code in the future.
- Use clear and consistent naming conventions for your CSS variables to avoid confusion and errors when using them in JavaScript.
- Test your code thoroughly to be sure to test your code thoroughly to ensure that it works as expected on all browsers and devices. Use JavaScript error analysis tools like the ReplayBird to identify and fix any issues that arise, and consider using automated testing tools to speed up your testing process.
- Keep your code modular when using CSS variables and JavaScript together, it's important to keep your code modular and well-organized. This will make it easier to update and maintain your code over time, and will also help prevent conflicts with other code on your website or application.
- Be aware of the scope of CSS variables and how it can affect their accessibility in JavaScript. Define variables at a high enough level in the DOM to ensure they are accessible to all elements that need to use them.
- Try keeping variables modular to keep your variables modular and reusable across different parts of your project. This will help to improve consistency and reduce duplication in your code.
- Consider using a preprocessor if you're working with a large number of CSS variables or complex styles, it may be helpful to use a preprocessor like Sass or Less. Preprocessors can help you organize your code and generate CSS with more advanced features like mixins and functions.
- Use a naming convention to establish a naming convention for your variables and stick to it across your project. This will make it easier to maintain and organize your code, especially when working with a team.
- Avoid overuse of CSS variables while CSS variables can be useful for reducing repetition and improving consistency in your styles, it's important to use them judiciously. Overusing CSS variables can make your code harder to read and maintain, and can also impact performance.
- Use JavaScript to set the value of CSS variables dynamically, based on user interactions or other events. This can be a powerful tool for creating dynamic theming or adjusting styles in response to user input.
- Optimize for performance to be mindful of the performance impact of using CSS variables and JavaScript together. Avoid using too many variables or excessively querying the DOM for variables, which can slow down your application.
- Keep your code organized and well-documented to make it easier to maintain and update over time. Consider using comments or other documentation tools to explain the purpose and use of your CSS variables and how they relate to your JavaScript code.
- Consider using a CSS preprocessor like Sass or Less, which can help you manage your variables more efficiently and provide additional features like nesting and math operations.
- Use CSS variables sparingly and strategically. While they can be useful for managing styles across multiple elements, overusing them can lead to cluttered and hard-to-maintain code.
- Use tools like browser dev tools or VS Code extensions to help identify and resolve syntax errors or other issues with CSS variables and JavaScript.
- Define variables in a central location to define your CSS variables in a single location, such as a CSS file or a CSS custom property set. This makes it easier to manage and update your variables across your entire project.
- If you're using CSS variables to set styles like CSS flexbox and CSS shadows that are critical to the functionality or accessibility of your website or application, be sure to plan ahead for fallback values in case the variables are not supported by a particular browser.
- Use variable names that clearly indicate their purpose, such as --primary-color or --font-size-small. This will make it easier to understand and maintain your code, especially when working with larger projects.
Conclusion:
In conclusion, CSS variables offer a flexible solution for creating efficient and consistent stylesheets.
By defining variables at the top level, developers can easily adjust the entire design by changing the values of those variables, leading to greater consistency and the ability to switch between different themes or color schemes.
CSS variables can also be combined with other CSS features to create more dynamic and responsive designs like 3D buttons. Overall, CSS variables provide developers with a valuable tool for creating more efficient, flexible, and consistent stylesheets.
ReplayBird - Driving Revenue and Growth through Actionable Product Insights
ReplayBird is a digital experience analytics platform that offers a comprehensive real-time insights which goes beyond the limitations of traditional web analytics with features such as product analytics, session replay, error analysis, funnel, and path analysis.
With Replaybird, you can capture a complete picture of user behavior, understand their pain points, and improve the overall end-user experience. Session replay feature allows you to watch user sessions in real-time, so you can understand their actions, identify issues and quickly take corrective actions. Error analysis feature helps you identify and resolve javascript errors as they occur, minimizing the negative impact on user experience.
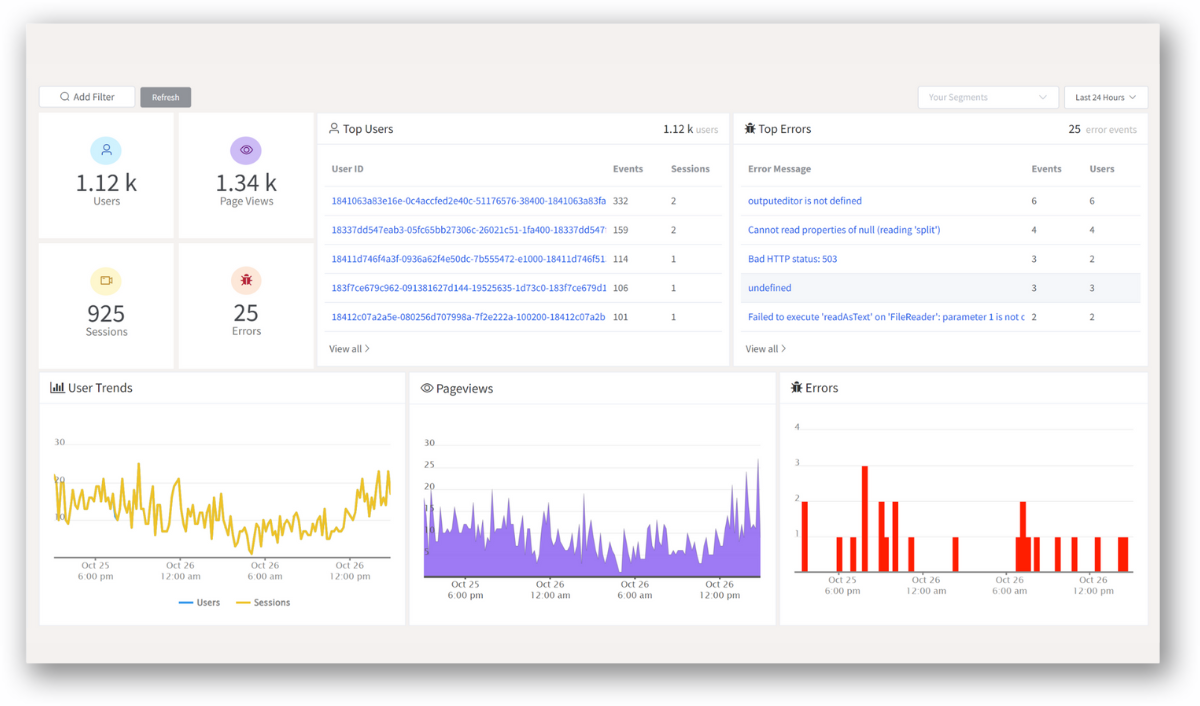
With product analytics feature, you can get deeper insights into how users are interacting with your product and identify opportunities to improve. Drive understanding, action, and trust, leading to improved customer experiences and driving business revenue growth.
Try ReplayBird 14-days free trial
Further Readings:
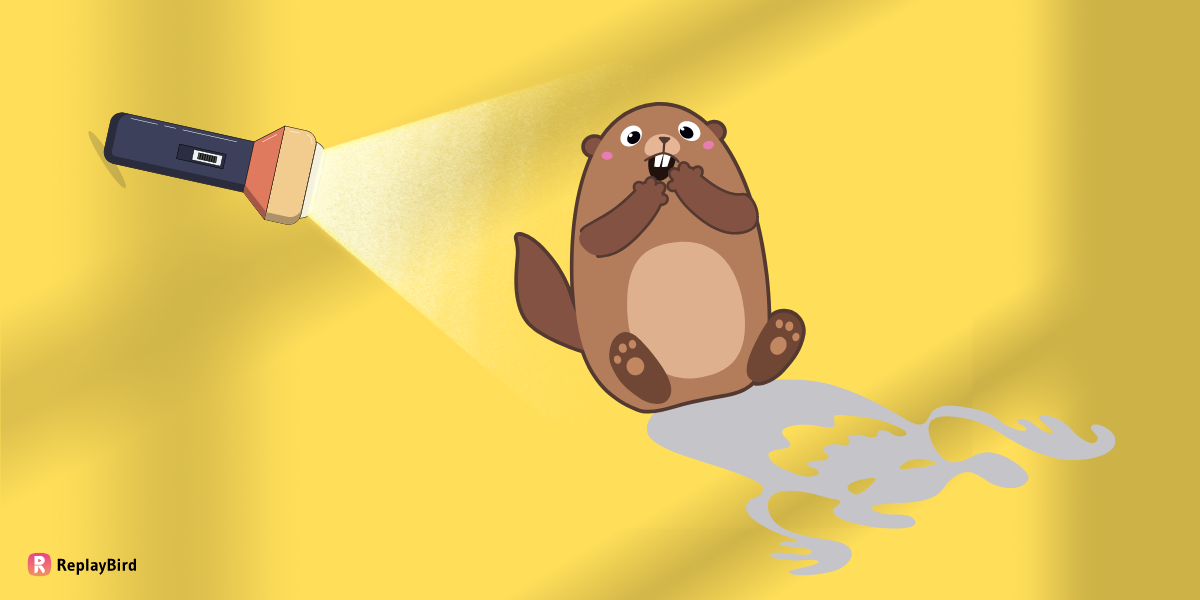
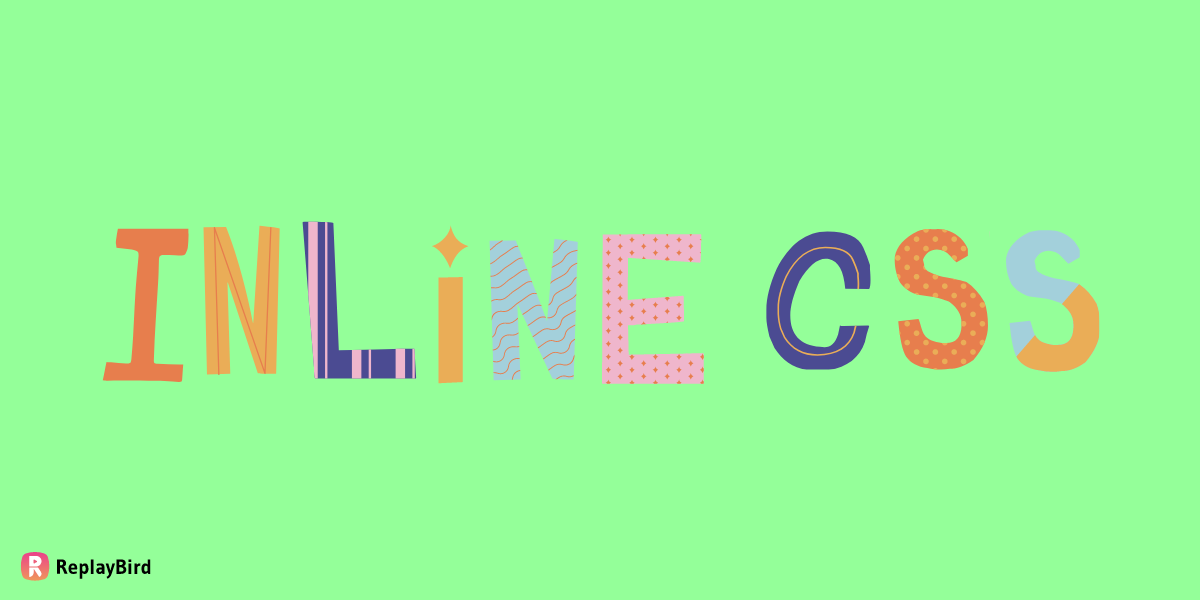
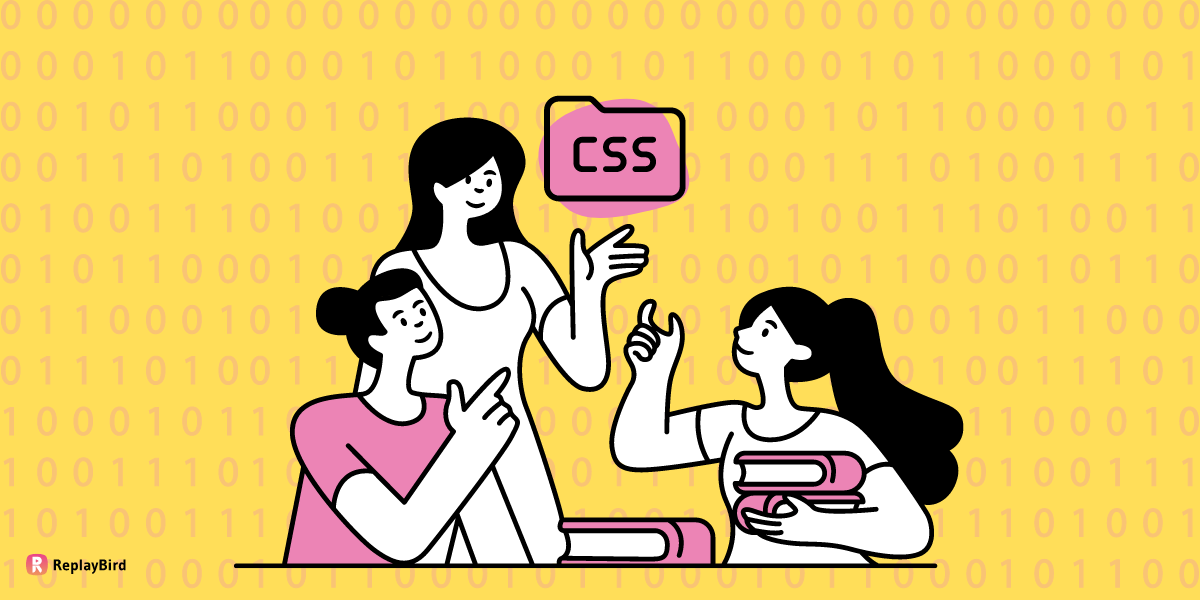