A TypeError occurs in programming when an operation is performed on a data type that is not supported or is incompatible with the operation. This error typically arises when attempting to perform actions like accessing properties or methods on null or undefined values, applying operators to mismatched data types, or calling functions on non-function values.
To resolve this, operations need to be performed on compatible data types, and handle potential edge cases like null or undefined values appropriately to prevent JavaScript TypeErrors in the code.
TypeErrors can occur in various programming scenarios where the expected type of data is not received or when incompatible data types are used.
Some specific use cases of TypeErrors include:
- Function Parameter Types: When a function expects certain data types as parameters but receives different types.
- Data Validation: During input validation, when the data type provided by the user does not match the expected type.
- Object Property Access: When attempting to access properties or methods of an object that does not exist or is not of the expected type.
- Type Conversion: Errors can occur when trying to convert data from one type to another, especially if the conversion is not possible or valid.
- Array Operations: When performing array operations such as sorting or mapping, if the elements are not of compatible types, TypeErrors can occur.
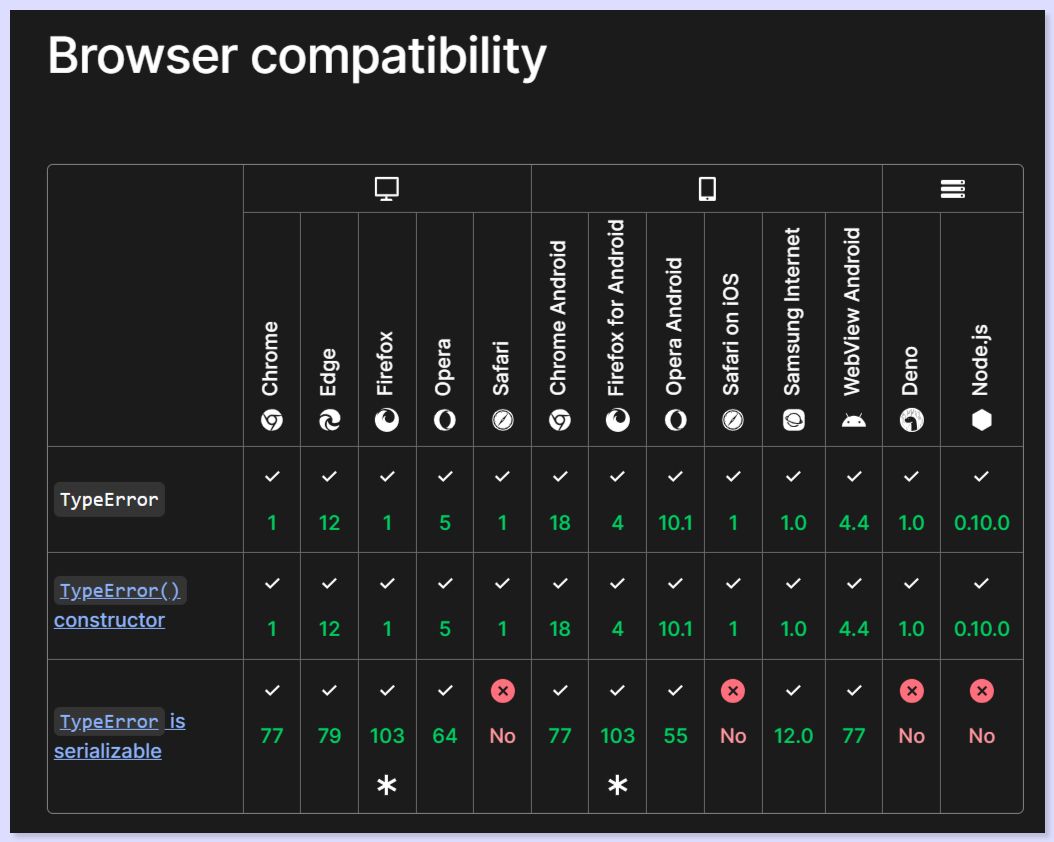
Before jumping our TypeError guide you can bookmark these other javaScript error blogs too:
- Javascript Errors: Permission, Recursion, URI, and Warnings (Part 1)
- RangeError, ReferenceError: Javascript Errors (Part 2)
- SyntaxError: JavaScript Errors (Part 3)
- TypeError: JavaScript Errors (Part 4) - Currently Reading
TypeErrors
Lets get into the list of all the TypeErrors:
#1 "x" has no properties
The error message "TypeError: 'x' has no properties" typically occurs in programming when attempting to access properties or attributes of an object that does not exist or is not defined. This could happen if the variable "x" is not initialized or if it is initialized to a value that is not an object.
TypeError: "x" has no properties
In JavaScript, for example, it could mean trying to access properties of an undefined variable or a primitive value like a number or string, which don't have properties like objects do. To resolve this error, ensure that the variable "x" is properly initialized and refers to an object with the expected properties before trying to access its properties.
#2 TypeError: "x" is (not) "y"
The JavaScript error message "TypeError: 'x' is (not) 'y'" indicates a type mismatch, suggesting that an operation is being performed between incompatible types.
TypeError: "x" is (not) "y"
For instance, attempting to compare or perform an operation between different data types, such as a string and a number, can trigger this error. To resolve it, ensure that the operands are of compatible types or convert them to the appropriate types before the operation.
#3 "x" is not a constructor
The error "TypeError: 'x' is not a constructor" arises when attempting to use "x" as a constructor function, but "x" is not a valid constructor. This typically occurs in JavaScript when trying to instantiate an object using a variable that does not reference a constructor function.
TypeError: "x" is not a constructor
To fix this error, ensure that "x" refers to a function that can be used as a constructor (i.e., it has a prototype property) or define "x" as a constructor function before using it to create new objects.
#4 "x" is not a function
The error message "TypeError: 'x' is not a function" indicates that the variable or object referenced by "x" is not callable as a function. This commonly occurs in JavaScript when attempting to invoke a method or function on a variable that does not hold a function reference.
TypeError: "x" is not a function
It might happen due to mistakenly assigning a non-function value to "x" or if "x" is expected to be a function but isn't defined as one. To resolve this error, ensure that "x" is correctly assigned to a function or an object with a callable property before attempting to call it as a function. Double-check variable assignments and function definitions for correctness.
#5 "x" is not a non-null object
The error "TypeError: 'x' is not a non-null object" suggests that the variable or object referenced by "x" does not hold a non-null object value. This commonly occurs in languages like JavaScript when attempting to access properties or methods of an object that is null or undefined.
TypeError: "x" is not a non-null object
It could result from a failure to properly initialize or assign a value to "x" before trying to access its properties or methods. To resolve this error, ensure that "x" holds a valid non-null object value by properly initializing or assigning it before attempting any operations on it. Use null/undefined checks to handle potential absence of values appropriately.
#6 "x" is read-only
The error "TypeError: 'x' is read-only" indicates an attempt to modify a variable or property that is marked as read-only, meaning it cannot be changed after its initial assignment.
TypeError: "x" is read-only
This commonly occurs when trying to modify built-in objects or constants. To resolve this error, either avoid modifying read-only variables or properties, or use a different variable or property that allows modifications.
#7 'x' is not iterable
The error "TypeError: 'x' is not iterable" suggests that the variable or object referenced by "x" cannot be iterated over using a loop or iterator because it does not support the iterable protocol.
TypeError: 'x' is not iterable
This typically occurs when trying to use a for...of loop, spread operator, or other iterable methods on a non-iterable value, such as null, undefined, or a primitive data type like a number or string. To resolve this error, ensure that "x" holds an iterable value, such as an array, object, or other data structure that supports iteration.
#8 More arguments needed
The error from JavaScript JavaScript "TypeError: More arguments needed" indicates that a function call requires additional arguments beyond those provided.
TypeError: More arguments needed
To resolve this, provide the required number of arguments expected by the function, as specified in its definition or documentation.
#9 Reduce of empty array with no initial value
The error "TypeError: Reduce of empty array with no initial value" occurs when using the reduce() method on an empty array without providing an initial value.
TypeError: Reduce of empty array with no initial value
In JavaScript, reduce() is used to apply a function to each element of an array and reduce it to a single value. However, when the array is empty and no initial value is provided, there's no starting point for the reduction process.
To fix this error, ensure that you provide an initial value as the second argument to reduce(), which will act as the initial accumulated value before iterating over the array elements.
#10 X.prototype.y called on incompatible type
The error "TypeError: X.prototype.y called on incompatible type" suggests that the method or property "y" defined on the prototype of object "X" is being invoked on a type that does not match the expected type.
TypeError: X.prototype.y called on incompatible type
This typically occurs when attempting to access a method or property of an object using prototype chaining, but the object's type does not match the expected prototype structure. Check the object's type and ensure that it aligns with the expected prototype structure to resolve this error.
#11 can't assign to property "x" on "y"
The JavaScript error "TypeError: can't assign to property 'x' on 'y': not an object" indicates an attempt to assign a value to the property "x" on object "y", which is not of type object.
TypeError: can't assign to property "x" on "y": not an object
This typically occurs when trying to access or modify a property on a non-object value, such as null or undefined. To resolve this error, ensure that the object referenced by "y" is properly initialized and holds an object type.
Double-check variable assignments and function returns to ensure that the target object is indeed an object and not null or undefined before attempting to access or modify its properties.
#12 can't convert BigInt to number
The error "TypeError: can't convert BigInt to number" occurs when attempting to convert a value of type BigInt to a number. BigInt is a numeric data type introduced in JavaScript to handle integers beyond the limit of the Number type.
TypeError: can't convert BigInt to number
However, direct conversion from BigInt to number is not allowed due to potential loss of precision. To resolve this error, either avoid converting BigInt to number directly or find an appropriate method to handle BigInt values, such as using BigInt-specific operations or converting to strings if necessary.
#13 can't convert x to BigInt
The error "TypeError: can't convert BigInt to number" indicates an attempt to convert a BigInt value to a number, which is not supported directly in JavaScript due to potential loss of precision.
TypeError: can't convert x to BigInt
To resolve this, handle BigInt values appropriately, avoiding direct conversion to numbers if precision is important. Consider using BigInt-specific operations or converting to strings for further processing where necessary.
#14 can't define property "x"
The error message from JavaScript "TypeError: can't define property 'x': 'obj' is not extensible" occurs when attempting to add a new property "x" to the object "obj" using the Object.defineProperty() method, but the object is not extensible.
TypeError: can't define property "x": "obj" is not extensible
In JavaScript, objects are extensible by default, meaning new properties can be added to them freely. However, if an object is marked as non-extensible using Object.preventExtensions() or a similar method, further additions of properties are disallowed.
To resolve this error, either ensure that the object is extensible before attempting to add properties or use a different approach to modify the object, such as directly assigning properties or creating a new object.
#15 can't delete non-configurable array element
The error "TypeError: can't delete non-configurable array element" occurs when attempting to delete an element from an array that is marked as non-configurable.
TypeError: can't delete non-configurable array element
In JavaScript, certain properties, including array elements, can be configured to prevent deletion using Object.defineProperty() with the configurable attribute set to false.
To resolve this error, either ensure that the array element is configurable before attempting deletion or use alternative methods to modify the array, such as setting the element to undefined or using array methods like splice() to remove elements.
#16 can't redefine non-configurable property "x"
The error "TypeError: can't redefine non-configurable property 'x'" occurs when attempting to redefine a property "x" that is marked as non-configurable.
TypeError: can't redefine non-configurable property "x"
In JavaScript, properties can be configured to prevent redefinition using Object.defineProperty() with the configurable attribute set to false. To resolve this error, ensure that the property is configurable before attempting redefinition or use a different approach to modify the property, such as setting its value directly if possible.
#17 cannot use 'in' operator to search for 'x' in 'y'
The error "TypeError: cannot use 'in' operator to search for 'x' in 'y'" indicates that the 'in' operator, typically used to check for the existence of a property in an object, is being applied to the non-object operand 'y'.
TypeError: cannot use 'in' operator to search for 'x' in 'y'
In JavaScript, the 'in' operator checks if a property exists in an object or its prototype chain. However, it cannot be used with non-object operands like primitive values (e.g., strings, numbers, booleans). To resolve this error, ensure that 'y' is an object or a type that can be searched for properties, or refactor the code to handle non-object values appropriately before using the 'in' operator.
#18 cyclic object value
The JavaScript error "TypeError: cyclic object value" occurs when there is a circular reference within an object, causing an infinite loop during JSON serialization. This commonly happens when an object contains a reference to itself or forms a cycle with other objects.
TypeError: cyclic object value
To resolve, refactor the object structure to eliminate circular references or use techniques like JSON.stringify() with a replacer function to handle cyclic structures.
#19 invalid 'instanceof' operand 'x'
The error "TypeError: invalid 'instanceof' operand 'x'" occurs when the 'instanceof' operator is used with an operand 'x' that is not a constructor function. In JavaScript, 'instanceof' is used to check if an object is an instance of a particular class or constructor function.
TypeError: invalid 'instanceof' operand 'x'
However, if 'x' is not a constructor function, such as a non-function value or a primitive, this error is thrown. To resolve, ensure that 'x' is a valid constructor function or refactor the code to use a different approach for type checking, such as 'typeof' or 'Object.prototype.constructor'.
#20 invalid Array.prototype.sort argument
The error "TypeError: invalid Array.prototype.sort argument" occurs when the argument provided to the sort() method of an array is not a valid comparator function. In JavaScript, sort() expects either no arguments or a comparator function that defines the sorting order.
TypeError: invalid Array.prototype.sort argument
If an invalid argument is passed, such as a non-function or undefined, this error is thrown. To resolve, ensure that the argument passed to sort() is a valid comparator function or remove it altogether if default sorting behavior is desired.
#21 invalid assignment to const "x"
The error "TypeError: invalid assignment to const 'x'" indicates an attempt to reassign a value to a constant variable 'x'. In JavaScript, variables declared with 'const' are immutable after initialization.
TypeError: invalid assignment to const "x"
Trying to change their value will result in this error. To resolve, either declare 'x' with 'let' if reassignment is needed or ensure that 'x' is only assigned a value once, at its declaration.
#22 property "x" is non-configurable and can't be deleted
The error "TypeError: property 'x' is non-configurable and can't be deleted" arises when attempting to delete a property 'x' from an object that is marked as non-configurable.
TypeError: property "x" is non-configurable and can't be deleted
In JavaScript, properties can be configured using Object.defineProperty() with the configurable attribute set to false, preventing their deletion. This ensures the stability and integrity of critical properties. To resolve this error, either remove the non-configurability constraint if it's not necessary, or refactor the code to work without the need for deleting the property.
Alternatively, consider setting the property's value to 'undefined' or 'null' if it's no longer needed, as these values are allowed in non-configurable properties.
#23 setting getter-only property "x"
The error from JavaScript "TypeError: setting getter-only property 'x'" occurs when attempting to assign a value to a property 'x' that has only a getter defined and no setter. In JavaScript, properties can have custom getter and setter functions, allowing controlled access.
TypeError: setting getter-only property "x"
However, if a property is defined with only a getter function, attempting to set its value directly results in this error. To resolve, either define a setter function for 'x' or modify the code to use a different property for assignment if direct modification is not intended.
Debug Your JavaScript Errors in Minutes
ReplayBird, a digital user experience analytics platform designed specifically to track JavaScript errors to revolutionize the debugging process by providing comprehensive insights into application errors.
ReplayBird records a comprehensive data set, including console logs, stack traces, network activity, and custom logs. With ReplayBird, diagnosing JavaScript exceptions becomes seamless. You can replay sessions, facilitating rapid debugging and resolution.
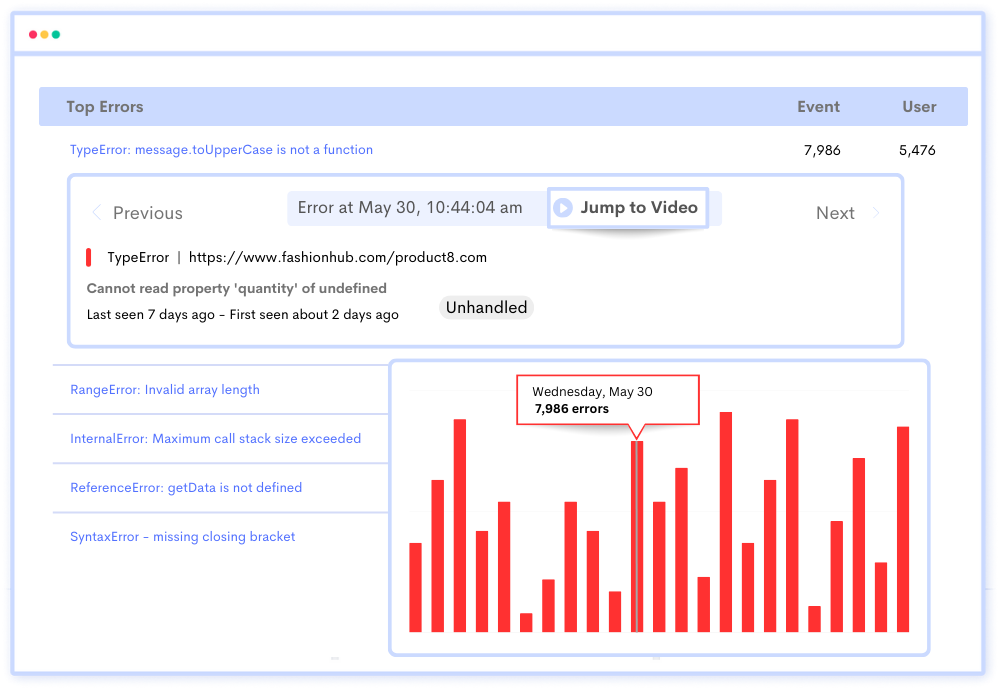
One key feature of ReplayBird is its ability to record and replay user sessions, allowing developers to recreate the exact sequence of events leading up to an error. This enables thorough debugging and facilitates understanding of the root cause of issues.
Additionally, ReplayBird's real-time error replay functionality enables developers to see errors as they occur in the user's browser, streamlining the debugging process and accelerating time to resolution.
Overall, ReplayBird's JavaScript error tracking enhances the debugging experience by providing actionable insights and facilitating efficient error resolution, ultimately leading to more robust and reliable applications.
Try ReplayBird 14-days free trial
Keep Reading More on JavaScript Errors
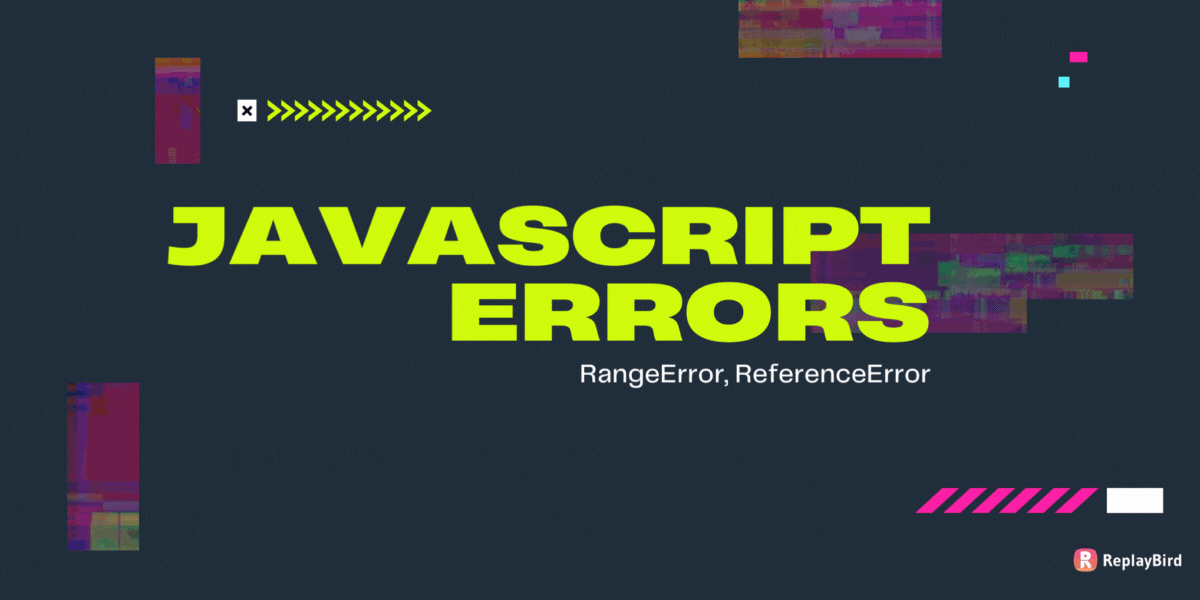
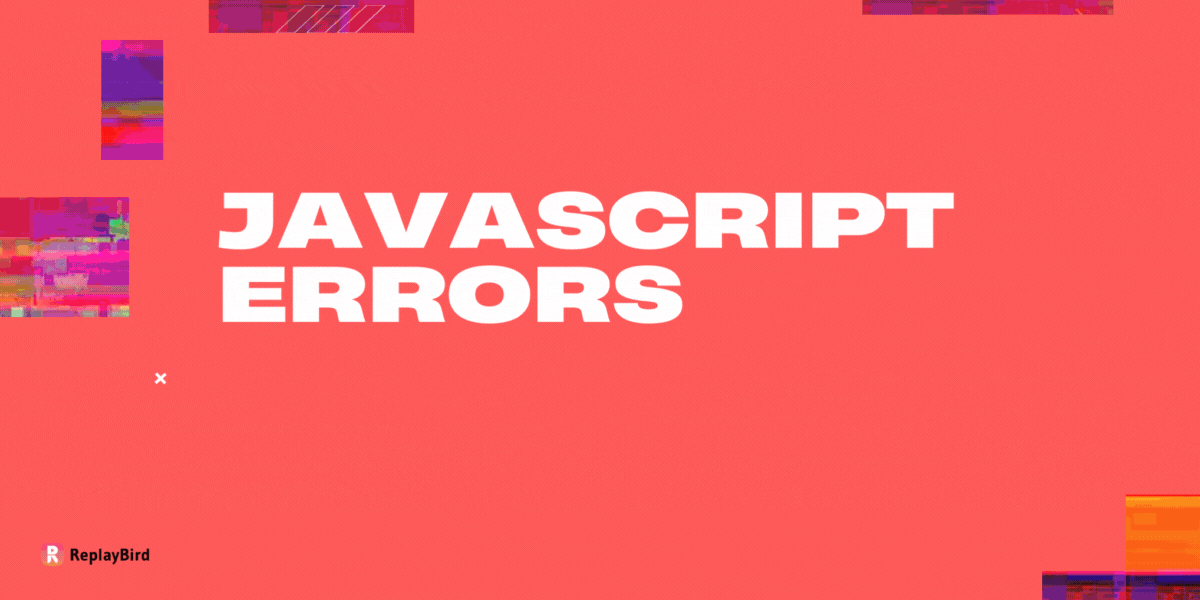
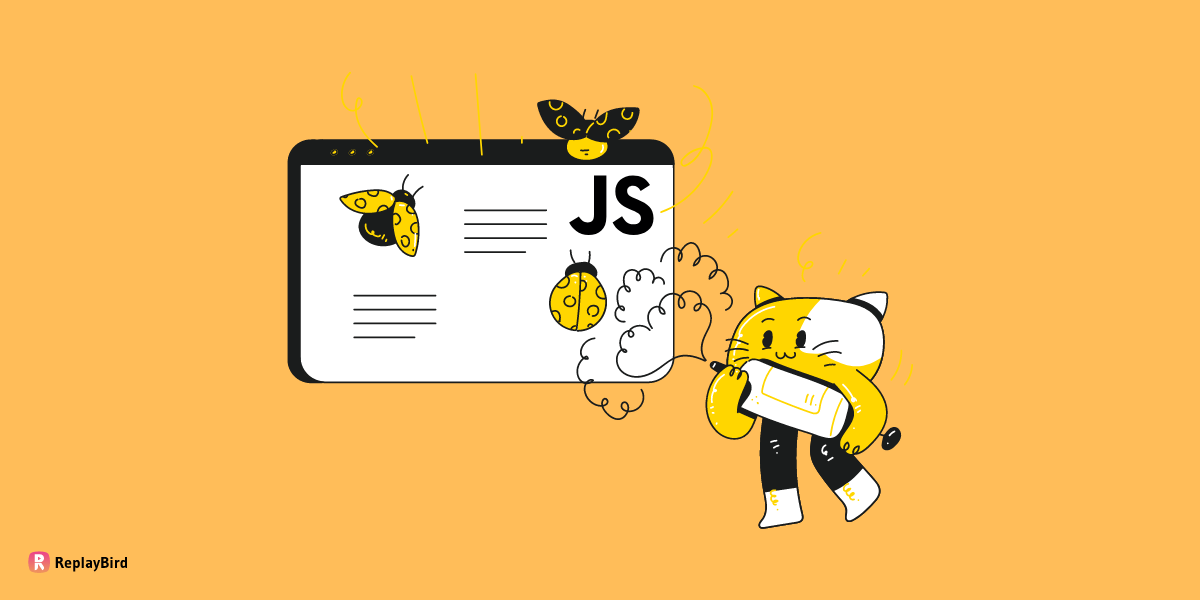