Understand Exceptional: Javascript Errors, apart from syntax errors, type errors, range errors, and reference errors in this part 1 of javascript errors. But we will continue this blog with parts 2, part 3, and part 4, explaining all the types of javascript errors.
Before jumping our syntax error guide you can bookmark these other javaScript error blogs too:
- Javascript Errors: Permission, Recursion, URI, and Warnings (Part 1) - Currently Reading
- RangeError, ReferenceError: Javascript Errors (Part 2)
- SyntaxError: JavaScript Errors (Part 3)
- TypeError: JavaScript Errors (Part 4)
Firstly, the following exceptional types of errors help in efficient debugging by allowing programmers to identify and rectify issues swiftly. Secondly, error handling lets program robustness to manage errors rather than crashing abruptly.
This also improves user experience by maintaining smooth program execution. Moreover, addressing security concerns, particularly permission errors, is imperative to safeguard sensitive data and prevent unauthorized access.
Optimizing code to mitigate performance issues, such as recursion errors, leads to faster and more efficient applications. Furthermore, adept error handling reflects professionalism, showcasing proficiency in programming and the ability to write maintainable, high-quality code.
Table of Content:
Ultimately, mastering JavaScript error handling is indispensable for writing dependable software, enhancing problem-solving capabilities, and establishing oneself as a valuable asset in the development community.
1. Permission Errors
DOMException
is one of the exceptional events that occurs as a result of calling or accessing a property of a web API. This is a security-related JavaScript error that occurs when the same-origin policy or other security restrictions are violated.
Error: Permission denied to access property "x"
Why does this error occur?
This JavaScript error message indicates that the code is trying to access a property named "x" on an object, but the security policies in place do not allow such access.
This occurs when a script running in one context (e.g., a web page) attempts to access a property on an object that belongs to a different origin (e.g., a different domain).
Example:
<head>
<iframe
id="myframe"
src="https://www.example.com">
</iframe>
<script>
window.onload = function () {
try {
// Attempting to access the contentWindow's document property
var iframeDocument = document.getElementById('myframe').contentWindow.document;
console.log(iframeDocument);
}
catch (error) {
console.error('Error: ' + error.message);
}
};
</script>
</head>
In this example, we are trying to access the document
property of the contentWindow
of an <iframe>
. If the iframe's content is loaded from a different origin, the browser's security policies prevent this kind of access, resulting in a Permission denied to access property 'document'
JS error.
This is also a common scenario when dealing with cross-originiframes
.
We can solve this JavaScript error by checking if the content inside the iframe
is served with appropriate Cross-Origin Resource Sharing (CORS) headers or implementing other secure cross-origin communication techniques, depending on the specific requirements of our application.
2. Recursion Errors
InternalError
in Firefox, indicating that this JavaScript error has occurred internally in the JavaScript engine, whereas it is also a RangeError
in Chrome and Safari, indicating that the value is not in the set or range of allowed values.
InternalError: too much recursion
Why does this JavaScript error occur?
This JavaScript error occurs when a function in a program calls itself excessively, leading to a stack overflow. Each function call consumes space on the call stack, and when the stack limit is reached, the browser throws this error.
This situation commonly happens due to unintentional infinite loops or recursive calls that lack a proper termination condition. Review and correct the recursive function that is terminating appropriately to resolve the JavaScript error.
Example:
function countdownWithExit(x) {
if (x <= 0) {
console.log("Liftoff!");
return;
}
console.log(x);
countdownWithExit(x - 1);
}
countdownWithExit(5);
In this example, the countdownWithExit
function has an exit condition (x <= 0)
, preventing infinite recursion. It has a proper exit condition to stop recursion when x
is less than or equal to 0.
Example with a High Exit Condition:
function countdownWithHighExit(x) {
if (x >= 1000000000000) {
console.log("High Liftoff!");
return;
}
console.log(x);
countdownWithHighExit(x + 1);
}
countdownWithHighExit(0);
Here, the exit condition (x >= 1000000000000
) is set to a high value, but this won't prevent the InternalError: Too Much Recursion
because the fundamental issue is the absence of a base case. It will eventually lead to a stack overflow due to the large number of recursive calls.
Example Missing Base Case:
function countdownWithoutBaseCase(x) {
console.log(x);
countdownWithoutBaseCase(x - 1); // Missing base case
}
// This will lead to "InternalError: too much recursion"
countdownWithoutBaseCase(5);
This example lacks a base case or exit condition. As a result, the function will infinitely call itself, leading to the InternalError: too much recursion
error. To avoid this JavaScript error, recursive functions must have a base case to halt the recursion.
3. URI Errors
This javascript error belongs to the category of,URIError
which is a built-in error type that is thrown when a URI-related operation is performed on a string that contains an invalid sequence or is incorrectly encoded.
URIError: malformed URI sequence
Why does this JavaScript error occur?
The javascript error message URIError: malformed URI sequence
indicates that there is a problem with a Uniform Resource Identifier (URI) in your JavaScript code, and the URI contains an invalid or improperly formatted sequence. URIs include web addresses and can be part of functions like decodeURIComponent()
or encodeURI()
.
This error occurs when you attempt to decode or encode a URI and the string being processed is not a valid URI sequence. It can be caused due to many actions, like including special characters that are not properly encoded or decoding sequences that don't represent a valid URI.
You can resolve this JS error by checking the URIs in your code and making sure that they are correctly formatted and encoded. If you are decoding a URI, make sure it was properly encoded in the first place. If you are encoding, that the input string follows URI encoding rules.
Encoding Example:
Encoding represents special characters, non-ASCII characters, or reserved characters in a standardized and safe way for transmission over the web.
encodeURI("\uD800\uDC00");
// "URIError: malformed URI sequence"
encodeURI("\uDC00\uD800");
// "URIError: malformed URI sequence"
This attempt results in a URIError because the surrogate pairs are not properly formed.
encodeURI("\uD800\uDFFF");
// "%F0%90%8F%BF"
Here, we have demonstrated a correctly formed surrogate pair that can be encoded without errors. The use of surrogate pairs is important when dealing with characters outside the Basic Multilingual Plane (BMP) in Unicode.
Decoding Example:
Decoding refers to the process of converting encoded or formatted data back to its original form or a more human-readable form. It is also often associated with handling encoded data in Uniform Resource Identifiers (URIs).
decodeURIComponent("%E4%A%A%G");
// "URIError: malformed URI sequence"
Here, the decodeURIComponent
function attempts to decode the URI sequence %E4%A%A%G
. However, this sequence is malformed because it ends with %G
, which is an invalid hexadecimal character.
// Properly encoded URI
decodeURIComponent("Hello%20World%21");
// "Hello World!"
In this example, the decodeURIComponent
function successfully decodes the properly encoded URI sequence. The input string is "Hello%20World%21"
, where %20
represents a space and %21
represents an exclamation mark. After decoding, the result is the expected string Hello World!
. This demonstrates the correct usage of URI encoding and decoding to handle special characters in a URI.
4. Warnings
The JavaScript warning file is being assigned a
typically occurs when JavaScript encounters an assignment operation where the left-hand side operand is a dash followed by a word, such as -file-
. This warning indicates a potential error or unintended behavior in the code.
Warning: -file- is being assigned a //# sourceMappingURL, but already has one
Explanation:
JavaScript uses the dash symbol (-
) for subtraction operations. However, if the dash is not part of a valid expression and is used as a standalone identifier, it can lead to unexpected behavior or trigger warnings. The warning "file is being assigned a" suggests that the interpreter is treating -file-
as an assignment operation rather than a subtraction or any other valid operation.
Causes:
- Typographical Error: The warning often occurs due to typographical errors in the code where a dash is incorrectly used as part of an identifier.
- Misinterpretation as Assignment: JavaScript interprets
-file-
as an assignment operation because of the dash at the beginning and end, considering it as a variable or property being assigned a value.
Example:
var -file- = "example.txt"; console.log(-file-);
In this example, the variable -file-
is being assigned the value "example.txt"
. However, the dashed characters around the variable name may lead to the warning "file is being assigned a" because JavaScript interprets it as an assignment operation rather than a valid variable name.
To resolve this JavaScript warning and potential issues, it's essential to review the code and ensure that variable names follow JavaScript's identifier naming rules. Variable names should not start or end with a dash unless intended for specific purposes like private properties in certain libraries or frameworks.
If -file-
is meant to represent a variable name, consider renaming it to follow standard naming conventions, such as file
or fileName
.
var file = "example.txt"; console.log(file);
You can prevent such JavaScript warnings and maintain clean, understandable code by adhering to proper naming conventions and avoiding using dashes in variable names inappropriately.
Warning: unreachable code after return statement
The JavaScript warning unreachable code after return statement
occurs when the JavaScript interpreter detects that there are code statements or expressions after a return
statement within a function.
This JS warning also indicates that the code following the return
statement will never execute, as the function will exit immediately after encountering the return
statement. Such unreachable code is typically considered redundant and may indicate a mistake or oversight in the code.
Explanation:
In JavaScript, the return
statement is used within functions to terminate the function's execution and optionally provide a value back to the caller. Once a return
statement is encountered, the function exits immediately, and any subsequent code within the function is ignored. Therefore, placing code after a return
statement is unnecessary and serves no purpose.
What Causes this JavaScript Error:
- Logical Error: The warning may occur due to a logical error in the code where the programmer inadvertently placed code after a
return
statement, assuming it would still execute. - Copy-paste Error: Sometimes, developers may accidentally leave code after a
return
statement when copying and pasting code from elsewhere.
Example:
function sum(a, b) {
if (a < 0 || b < 0) {
return "Inputs must be positive"; // Return statement } // Unreachable code after return statement console.log("Calculating sum..."); return a + b; }
In this example, the sum
function is intended to return the sum of two positive numbers a
and b
. However, if either a
or b
is negative, the function returns a message indicating that inputs must be positive.
Any code after the return
statement, such as the console.log("Calculating sum...")
, is unreachable and will trigger the warning unreachable code after return statement.
This warning alerts developers to review the function and remove the unreachable code to improve code readability and maintainability.
To address this warning, developers should refactor the function to let all code paths leading to a return
statement terminates the function, and no code is placed after a return
statement within the function body.
function sum(a, b) {
if (a < 0 || b < 0) {
return "Inputs must be positive"; // Return statement } // Code logically placed before return statement console.log("Calculating sum..."); return a + b; }
By addressing the warning and removing unreachable code, developers can improve code quality so that functions behave as expected.
Debug Your JavaScript Errors in Minutes
ReplayBird, a digital user experience analytics platform designed specifically to track JavaScript errors to revolutionize the debugging process by providing comprehensive insights into application errors.
ReplayBird records a comprehensive data set, including console logs, stack traces, network activity, and custom logs. With ReplayBird, diagnosing JavaScript exceptions becomes seamless. You can replay sessions, facilitating rapid debugging and resolution.
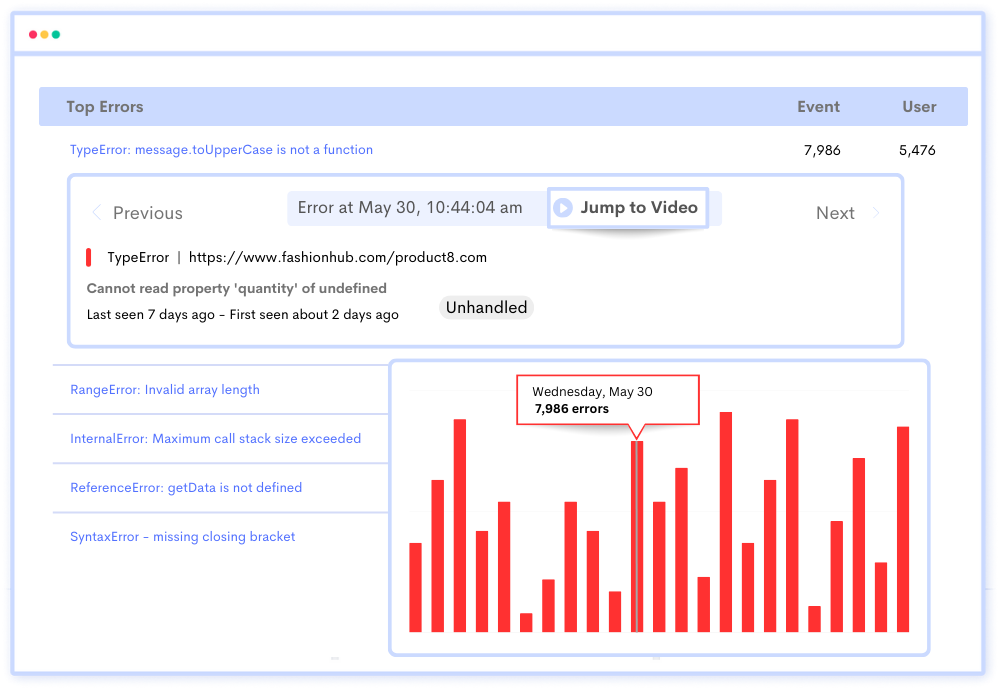
One key feature of ReplayBird is its ability to record and replay user sessions, allowing developers to recreate the exact sequence of events leading up to an error. This enables thorough debugging and facilitates understanding of the root cause of issues.
Additionally, ReplayBird's real-time error replay functionality enables developers to see errors as they occur in the user's browser, streamlining the debugging process and accelerating time to resolution.
Overall, ReplayBird's JavaScript error analysis improves the debugging experience by providing actionable insights and facilitating efficient error resolution, ultimately leading to more robust and reliable applications.