Understanding RangeError and ReferenceError in JavaScript is the best way to write robust, error-free code. RangeError occurs when a value falls outside an acceptable range, like attempting to create an array with a negative length.
By knowing RangeError, developers can operate their code within boundaries, improving code reliability. ReferenceError, on the other hand, happens when accessing an undefined variable or function due to typos or scoping issues.
Understanding ReferenceError helps in efficiently identifying and rectifying such issues, leading to cleaner, maintainable code and minimizing runtime errors. Overall, familiarity with these error types is going to let you write more resilient and dependable JavaScript applications.
Before jumping our syntax error guide you can bookmark these other javaScript error blogs too:
- Javascript Errors: Permission, Recursion, URI, and Warnings (Part 1)
- RangeError, ReferenceError: Javascript Errors (Part 2) - Currently Reading
- SyntaxError: JavaScript Errors (Part 3)
- TypeError: JavaScript Errors (Part 4)
Table of Content
- JavaScript Error: RangeError
- BigInt division by zero
- BigInt negative exponent
- argument is not a valid code point
- invalid array length
- invalid date
- precision is out of range
- radix must be an integer
- repeat count must be less than infinity
- repeat count must be non-negative
- x can't be converted to BigInt because it isn't an integer
- JavaScript Error: ReferenceError
JavaScript Error: RangeError
1. RangeError: BigInt division by zero
The RangeError: BigInt division by zero
indicates an attempt to divide a BigInt value by zero, which is mathematically invalid.
BigInts are used for large integer values, whereas this error typically occurs when attempting to perform a division operation on a BigInt where the divisor is zero, violating the mathematical rules of division.
RangeError: BigInt division by zero
Why does this JavaScript error occur?
BigInts allow for representing very large numbers, but dividing any number by zero, regardless of size, will result in this JS error. To resolve it, ensure the denominator (the number you're dividing by) is not zero.
Example:
Let's say you're working on a web application that handles large integer values using JavaScript's BigInt
data type. You have a piece of code that performs division involving BigInt
numbers. However, in one instance, the divisor is mistakenly set to zero.
let dividend = BigInt(100);
let divisor = BigInt(0);
// Attempting to perform division
let result = dividend / divisor; // This line will cause a RangeError
console.log(result);
In JavaScript, the BigInt
data type allows you to work with integers of arbitrary precision. However, when attempting to divide a BigInt
by zero, a RangeError
is thrown with the message BigInt division by zero
. This JS error occurs because dividing any number by zero is mathematically undefined, including for BigInt
values.
To avoid this JS error can implement appropriate checks in your code to prevent division by zero scenarios, such as validating user input.
2. RangeError: BigInt negative exponent
The RangeError: BigInt negative exponent
occurs when attempting to raise a BigInt to a negative exponent, which is unsupported. BigInts represent large integer values, and integers' exponentiation with negative exponents is undefined.
This JavaScript error indicates an attempt to perform an operation that violates the mathematical rules for BigInt exponentiation.
RangeError: BigInt negative exponent
Why does this JavaScript error occur?
The RangeError: BigInt negative exponent
occurs when raising a BigInt to a negative exponent. In JavaScript, BigInts support arithmetic operations like exponentiation, but raising a BigInt to a negative exponent results in a JS error because it's undefined in mathematics.
Example:
Suppose you're developing a financial application that calculates compound interest using BigInt numbers in JavaScript. You have a function that computes the future value of an investment based on the principal amount, interest rate, and the number of years. However, due to a logical JS error, the function mistakenly tries to calculate compound interest with a negative exponent.
function calculateFutureValue(principal, interestRate, years) {
// Convert interest rate to a decimal
let rate = interestRate / BigInt(100);
// Calculate future value using compound interest formula
let futureValue = principal * (BigInt(1) + rate) ** BigInt(-years); // Error occurs here
return futureValue;
}
let principalAmount = BigInt(1000);
let interestRate = BigInt(5); // 5% interest rate
let years = BigInt(10);
let futureValue = calculateFutureValue(principalAmount, interestRate, years);
console.log(futureValue);
Here, the RangeError with the message "BigInt negative exponent" occurs when calculating compound interest using a negative exponent with BigInt numbers. The negative exponent is encountered in the compound interest formula, where the future value is calculated using (1 + rate) ** (-years)
.
Attempting to raise a number to a negative exponent is mathematically invalid and results in the RangeError.
To resolve this issue, you can review the logic of your exponent calculations and handle cases where negative exponents may occur, such as validating user input or adjusting the algorithm to handle such scenarios gracefully.
3. RangeError: argument is not a valid code point
The RangeError: argument is not a valid code point
typically arises in JavaScript when a function expecting a Unicode code point receives an argument that doesn't correspond to a valid code point.
This JavaScript error indicates that the provided argument is outside the valid range of Unicode characters. It commonly occurs when working with strings or characters in JavaScript.
RangeError: argument is not a valid code point
Why does this JavaScript error occur?
The "RangeError: argument is not a valid code point" error occurs when attempting to use a method or operation that expects a valid Unicode code point as an argument, but the provided value is not a valid code point.
Unicode code points represent individual characters in the Unicode standard and must fall within a specific range to be considered valid. This JavaScript error can occur if you pass an invalid or out-of-range value to a function or method that expects a valid code point.
To resolve this JS error, you need to check if the value being passed as an argument is a valid Unicode code point according to the Unicode standard. You may need to validate or sanitize input data to prevent passing invalid code points to functions or methods.
Example:
Imagine you're developing a text processing tool in JavaScript that handles Unicode characters and performs operations on strings. You have a function that iterates over a string and processes each character individually.
However, now you have found that the function receives an invalid Unicode code point as an argument.
function processString(str) {
let result = '';
for (let i = 0; i < str.length; i++) {
// Process each character in the string
let char = str[i];
// Perform some operation on the character
// For example, convert to uppercase
result += char.toUpperCase(); // Error occurs here
}
return result;
}
let inputString = 'Hello\uD83D'; // Unicode code point U+1F600 (😀) is incomplete
let processedString = processString(inputString);
console.log(processedString);
In this scenario, the RangeError with the message argument is not a valid code point
occurs when attempting to process a string that contains an incomplete Unicode code point.
Unicode characters, specifically represented by surrogate pairs (such as emojis), require multiple code units to represent a single character. If the input string contains a truncated or incomplete code point, attempting to process it as a single character will result in the RangeError.
Your string processing functions correctly handle Unicode characters. You can use libraries or built-in functions that provide robust Unicode support, such as String.prototype.codePointAt()
and String.fromCodePoint()
, to correctly handle characters represented by multiple code units. Additionally, consider validating input strings containing valid Unicode sequences before processing them.
4. RangeError: invalid array length
The RangeError: invalid array length
in JavaScript indicates an attempt to create an array with a length that's either negative or exceeds the maximum allowed array length.
This JS error commonly occurs when creating an array with an invalid or excessively large length, typically due to a programming mistake or incorrect calculation.
RangeError: invalid array length
Why does this JavaScript error occur?
The RangeError: invalid array length
error occurs when attempting to create an array with an invalid length in JavaScript.
This typically happens when you try to create an array with a length that is either negative or exceeds the maximum allowed array length in JavaScript, which is 2^32 - 1 (approximately 4.29 billion) for most JavaScript engines.
Example:
Suppose you're working on a web application that dynamically creates arrays based on user input or other factors. You have a piece of code that attempts to create an array with an invalid length value.
let invalidLength = -1;
let newArray = new Array(invalidLength); // Error occurs here
In JavaScript, the Array constructor can be used to create arrays with a specific length. However, if the length value provided to the constructor is negative or exceeds the maximum allowed array length, a RangeError with the message invalid array length
will be thrown.
5. RangeError: invalid date
The RangeError: invalid date
occurs in JavaScript when attempting to create a Date object with invalid parameters.
This could happen when passing incorrect values for year, month, day, hour, minute, second, or milliseconds.
RangeError: invalid date
Why does this JavaScript error occur?
The "RangeError: invalid date" error occurs in JavaScript when attempting to create or parse a date object with invalid date values. This can happen for several reasons:
- Incorrect Date Format: When parsing a date string, ensure that it follows the correct format expected by the Date object constructor or parsing functions like Date.parse(). If the format is invalid or unrecognized, it can lead to this JavaScript error.
- Out-of-Range Values: The date values (year, month, day, hour, minute, second, millisecond) should fall within valid ranges. For example, months should be between 0 (January) and 11 (December), and days should be between 1 and the number of days in the given month.
- Invalid Date Values: Certain date values might be invalid, such as February 30th or April 31st. Attempting to create a Date object with such values will result in this error.
To resolve this JavaScript error, the date values you're working with must be valid and within the expected ranges. If parsing date strings, make sure they conform to the expected format. Additionally, consider using libraries like moment.js or built-in methods like Intl.DateTimeFormat for safer and more robust data handling.
Example:
Let's consider a scenario where you're building a web application that allows users to input dates for scheduling appointments or events. You have a function that attempts to create a Date object from user-provided data, but sometimes the input data is malformed or invalid.
function createDateObject(dateString) {
let date = new Date(dateString); // Error occurs here
return date;
}
let userInput = "2024-02-30"; // Invalid date with February 30th
let appointmentDate = createDateObject(userInput);
console.log(appointmentDate);
In JavaScript, the Date object constructor accepts various formats for creating date objects. However, if the provided date string represents an invalid date (such as February 30th), attempting to create a Date object with that string will result in a RangeError with the message "invalid date."
In the example scenario, the user input "2024-02-30" represents February 30th, which is not a valid date. When the function createDateObject attempts to create a Date object using this invalid date string, it results in the RangeError.
Validating user input before creating a Date object is essential to handling this error. You can implement input validation routines so that the date strings users provide adhere to a valid format and represent valid dates.
6. RangeError: precision is out of range
The RangeError: precision is out of range
typically occurs when attempting to use a method or function that requires a precision parameter that falls outside the valid range.
This might happen with methods related to numerical calculations or formatting that expect a precision value within a specific range, and the provided value exceeds that range.
RangeError: precision is out of range
Why does this JavaScript error occur?
The RangeError: precision is out of range
error typically occurs when attempting to create a Number with a precision value outside the valid range. In JavaScript, the precision of numbers is finite and falls within a specific range.
This error might arise when trying to create a Number with a precision value that is too large or too small, beyond what JavaScript can handle.
Example:
Consider a scenario where you're developing a scientific calculator application in JavaScript. You have a function that performs a mathematical operation with a specified precision level, allowing users to control the number of decimal places in the result. However, in some cases, the precision level provided by the user exceeds the valid range for the operation.
function performOperationWithPrecision(number, precision) {
let result = number.toPrecision(precision); // Error occurs here
return result;
}
let userInputNumber = 123.456789;
let userInputPrecision = 30; // Precision level provided by the user
let result = performOperationWithPrecision(userInputNumber, userInputPrecision);
console.log(result);
In JavaScript, the toPrecision()
method is used to format a number using a specified precision level. However, the precision parameter must be within a valid range to avoid JavaScript errors. If the precision level provided by the user exceeds the valid range, a RangeError with the message precision is out of range
will be thrown.
To prevent this JS error, you need to validate the precision level provided by the user before using it in operations that involve formatting numbers.
You can implement limits on the acceptable precision range based on your application's requirements and provide appropriate feedback to users when they attempt to use invalid precision values.
Additionally, consider implementing alternative approaches for handling precision formatting, such as using the toFixed()
method, which doesn't have the same precision range restrictions.
7. RangeError: radix must be an integer
The RangeError: radix must be an integer
indicates that the radix parameter provided to a function like parseInt()
is not an integer. Radix specifies the base of the numeral system to be used, typically between 2 and 36.
This JS error occurs when the radix parameter is not an integer within that range, violating the expected format.
RangeError: radix must be an integer
Why does this JavaScript error occur?
The RangeError: radix must be an integer
error occurs in JavaScript when attempting to use the parseInt()
or parseFloat()
functions with a radix argument that is not an integer.
In JavaScript, when used, the radix parameter specifies the base of the numeral system to be used. It must be an integer between 2 and 36.
Example:
Let's say you're building a web application that allows users to convert numbers between different radix systems (binary, octal, decimal, hexadecimal). You have a function that performs radix conversion, but sometimes users input non-integer radix values.
function convertToDecimal(input, radix) {
let decimalValue = parseInt(input, radix); // Error occurs here
return decimalValue;
}
let userInput = "101010"; // Binary input
let radix = 2.5; // Non-integer radix provided by the user
let decimalValue = convertToDecimal(userInput, radix);
console.log(decimalValue);
In JavaScript, the parseInt()
function is commonly used to parse strings and convert them to integers using a specified radix (base). However, the radix parameter must be an integer between 2 and 36 inclusive. If the radix value provided by the user is not an integer, a RangeError with the message radix must be an integer
will be thrown.
In the example scenario, the user provides a radix value of 2.5, which is not an integer. When the convertToDecimal function attempts to parse the input string using this non-integer radix value, it results in the RangeError.
The user's radix value must be an integer before using it in radix conversion operations. You can validate the radix input and provide appropriate feedback to users when they enter non-integer values. Additionally, consider implementing additional input validation measures to ensure that the radix value falls within the acceptable range (between 2 and 36).
8. RangeError: repeat count must be less than infinity
The RangeError: repeat count must be less than infinity
typically occurs when attempting to repeat a string a number of times that exceeds the maximum allowed value.
This JavaScript error is raised when the repeat count parameter provided to a method like String.prototype.repeat()
is greater than the maximum allowed value, which is typically the maximum safe integer in JavaScript.
RangeError: repeat count must be less than infinity
Why does this JavaScript error occur?
The RangeError: repeat count must be less than infinity
error occurs when attempting to use the String.prototype.repeat()
method with a repeat count that is not within the valid range.
In JavaScript, the repeat()
method creates and returns a new string by concatenating the specified string a certain number of times. The repeat count parameter must be a non-negative integer less than or equal to Number.MAX_SAFE_INTEGER
, which is the maximum safe integer value in JavaScript.
Example:
Consider a scenario where you're developing a text generation tool in JavaScript that allows users to repeat a given string multiple times. You have a function that performs string repetition, but sometimes, users input an excessively large repeat count that exceeds the limits.
function repeatString(str, count) {
let repeatedString = str.repeat(count); // Error occurs here
return repeatedString;
}
let userInput = "Hello!";
let repeatCount = Infinity; // Excessively large repeat count provided by the user
let repeatedString = repeatString(userInput, repeatCount);
console.log(repeatedString);
In JavaScript, the repeat()
The method is used to construct and return a new string by concatenating the specified string several times. However, to avoid JavaScript errors, the count parameter must be a non-negative integer less than or equal to the maximum safe integer value (2^53 - 1). If the repeat count provided by the user exceeds this limit, a RangeError with the message repeat count must be less than infinity
will be thrown.
In the example scenario, the user provides a repeat count of Infinity, which is beyond the acceptable range for the repeat() method. When the repeatString function attempts to repeat the input string using this excessively large repeat count, it results in the RangeError.
The user's repeat count must be within the acceptable range before being used in string repetition operations. Based on your application's requirements, you can validate the input and limit the maximum allowed repeat count.
Additionally, consider providing feedback to users when they attempt to use excessively large repeat counts to prevent such JavaScript errors and improve the user experience.
9. RangeError: repeat count must be non-negative
The RangeError: repeat count must be non-negative
indicates an attempt to repeat a string a negative number of times. This JavaScript error arises when the repeat count parameter provided to a method  String.prototype.repeat()
is negative.
The repeat count parameter must be a non-negative integer, as negative counts are not valid for string repetition.
RangeError: repeat count must be non-negative
Why does this JavaScript error occur?
The RangeError: repeat count must be non-negative
error occurs when attempting to use the String.prototype.repeat()
method with a negative repeat count parameter.
In JavaScript, the repeat() method creates and returns a new string by concatenating the specified string several times. The repeat count parameter must be a non-negative integer, meaning it cannot be negative.
For example, "abc".repeat(3) will result in "abcabcabc".
To resolve this JavaScript error, ensure that the repeat count you pass to the repeat() method is a non-negative integer. If you're encountering this error unexpectedly, double-check the value used as the repeat count to ensure it is not negative.
Example:
Let's imagine you're working on a chat application where users can send messages with custom emojis. You have a function that generates a string representation of a message, including emojis, by repeating the emoji characters based on a count provided by the user. However, sometimes, users provide negative values for the repeat count.
function generateMessageWithEmoji(emoji, count) {
if (count < 0) {
return "Invalid repeat count. Please provide a non-negative value.";
}
let message = emoji.repeat(count); // Error occurs here
return message;
}
let userInputEmoji = "😊";
let repeatCount = -3; // Negative repeat count provided by the user
let messageWithEmoji = generateMessageWithEmoji(userInputEmoji, repeatCount);
console.log(messageWithEmoji);
In JavaScript, the repeat() method constructs and returns a new string by concatenating the specified string a given number of times. However, the count parameter must be a non-negative integer to avoid JavaScript errors. If the repeat count provided by the user is negative, a RangeError with the message "repeat count must be non-negative" will be thrown.
In the example scenario, the user provides a negative repeat count of -3, which is invalid. When the generateMessageWithEmoji function attempts to repeat the emoji character using this negative repeat count, it results in the RangeError.
Additionally, consider imposing constraints on the input values or handling negative counts gracefully in your application's logic to ensure a smooth user experience.
10. RangeError: x can't be converted to BigInt because it isn't an integer
The RangeError: x can't be converted to BigInt because it isn't an integer
occurs when attempting to convert a non-integer value to a BigInt. BigInts are used to represent arbitrarily large integers in JavaScript.
This error indicates that the provided value for conversion is not an integer, violating the requirement for converting to a BigInt.
RangeError: x can't be converted to BigInt because it isn't an integer
Why does this JavaScript error occur?
The RangeError: x can't be converted to BigInt because it isn't an integer
error occurs when attempting to convert a non-integer value to a BigInt in JavaScript.
BigInts are a relatively new numeric data type in JavaScript introduced to represent arbitrary precision integers. However, BigInts can only be created from integer values.
For example, attempting to convert a decimal number or a non-numeric value like a string to a BigInt will result in this JavaScript error.
To resolve this JavaScript error, you need to attempt only once to convert integer values to BigInts. If you need to work with non-integer values, consider using other data types like Numbers or Strings as appropriate. If you're working with a string representing an integer, you can first parse it into an integer using parseInt() or parseFloat() before converting it to a BigInt.
Example:
Let's suppose you're developing a financial application in JavaScript that deals with large numbers for precise calculations, using BigInt. You have a function intended to convert numeric values to BigInt. However, sometimes users provide non-integer or non-numeric inputs, leading to conversion errors.
function convertToBigInt(x) {
if (!Number.isInteger(x)) {
return "Invalid input. BigInt conversion requires an integer.";
}
let result = BigInt(x); // Error occurs here
return result;
}
let userInput = 10.5; // Non-integer input provided by the user
let bigIntValue = convertToBigInt(userInput);
console.log(bigIntValue);
In JavaScript, BigInt is used to represent integers of arbitrary precision. However, when attempting to convert a value to BigInt, it must be an integer. If the provided value is not an integer, a RangeError with the message "x can't be converted to BigInt because it isn't an integer" will be thrown.
In the example scenario, the user provides a non-integer value of 10.5. When the convertToBigInt function attempts to convert this non-integer value to BigInt, it results in the RangeError.
To prevent this JavaScript error, it's important to validate the input before converting it to BigInt. The input value is an integer by checking its type or using appropriate validation logic.
JavaScript Error: ReferenceError
1. ReferenceError: "x" is not defined
The ReferenceError: 'x' is not defined occurs when referencing a variable or identifier that hasn't been declared or is out of scope.
This JavaScript error typically arises when trying to use a variable or identifier that hasn't been defined within the current scope or context of the program. Double-check the spelling and scope of the variable 'x'.
ReferenceError: "x" is not defined
Why does this JavaScript error occur?
The ReferenceError: 'x' is not defined
error occurs when you attempt to use a variable or identifier that has not been declared or is out of scope.
Here are some common scenarios where this JavaScript error might occur:
Using a variable before it's declared: Ensure that the variable 'x' is declared before you attempt to use it.
Example:
var x = 10;
console.log(x);
Misspelling a variable name: Double-check that the variable name 'x' is spelled correctly and matches the variable you intended to use.
Variable out of scope: If 'x' is declared within a certain scope (e.g., inside a function or block), make sure you're trying to access it from a valid scope.
Using strict mode: In strict mode, JavaScript is less forgiving about certain types of JavaScript errors. If you're using strict mode ('use strict';), ensure that variables are declared before use.
To resolve this JavaScript error, locate where 'x' should be declared and ensure it is properly defined in the correct scope before using it.
2. ReferenceError: assignment to undeclared variable "x"
The "ReferenceError: assignment to undeclared variable 'x'" indicates an attempt to assign a value to a variable 'x' that hasn't been declared using the 'var', 'let', or 'const' keywords.
This JavaScript error occurs when trying to assign a value to a variable that doesn't exist in the current scope or hasn't been properly declared.
ReferenceError: assignment to undeclared variable "x"
Why does this JavaScript error occur?
The JavaScript error message ReferenceError: assignment to undeclared variable 'x'
indicates an attempt to assign a value to a variable 'x' that hasn't been declared before.
For example:
x = 10;
To resolve this error:
Declare the variable before assigning a value to it:
var x = 10;
Use 'let' or 'const' to declare the variable if you're working within a block scope:
let x = 10;
or
const x = 10;
Before assigning a value to the variable 'x,' ensure that it is properly declared and accessible in the current scope.
Also, remember that JavaScript allows implicit declaration of variables in non-strict mode, but this practice is generally discouraged as it can lead to unexpected behavior. It's good practice to always explicitly declare variables before using them.
3. ReferenceError: can't access lexical declaration 'X' before initialization
The ReferenceError: can't access lexical declaration 'X' before initialization
occurs when attempting to access a variable declared with 'let' or 'const' before it has been initialized.
This JavaScript error is a result of attempting to access a variable in a temporal dead zone—between the start of the block scope and the actual declaration. Ensure variables are initialized before accessing them.
ReferenceError: can't access lexical declaration 'X' before initialization
Why does this JavaScript error occur?
The JavaScript error message "ReferenceError: can't access lexical declaration 'X' before initialization" occurs when attempting to access a variable declared with let or const before initialization. In JavaScript, variables declared with let and const are hoisted to the top of their containing block (or function) during the compilation phase, but they are not initialized until their actual declaration is encountered in the code.
Example:
console.log(x); // ReferenceError: can't access lexical declaration 'x' before initialization
let x = 10;
To resolve this error, ensure that you're not trying to access the variable before it's initialized. Move the usage of the variable 'x' below its declaration:
let x = 10;
console.log(x); // Now it's safe to access 'x'
This JavaScript error commonly occurs when attempting to access variables within a block of code (such as within an if statement or a loop) before they've been declared and initialized within that block. Always ensure that variables are declared and initialized before attempting to access them.
4. ReferenceError: deprecated caller or arguments usage
The ReferenceError: deprecated caller or arguments usage
indicates an attempt to use the deprecated 'caller' or 'arguments' properties within a function. These properties are deprecated and not recommended for use in modern JavaScript.
It's advised to refactor code to avoid reliance on these properties, as they can lead to confusion and unexpected behavior.
ReferenceError: deprecated caller or arguments usage
Why does this JavaScript error occur?
The JS error message "ReferenceError: deprecated caller or arguments usage" occurs when you attempt to access the caller or arguments properties of a function. These properties are deprecated in modern JavaScript and are not allowed in strict mode.
The caller property refers to the function that invoked the currently executing function, while the arguments property refers to an array-like object containing the arguments passed to the function.
For example:
function exampleFunction() {
console.log(arguments); // ReferenceError: deprecated caller or arguments usage
}
To resolve this JS error:
Avoid using caller and arguments: Instead, consider using modern JavaScript features like rest parameters (...args) or the arguments object can be converted to a regular array using Array.from(arguments) or using the spread operator.
You need to make sure that you're not using caller or arguments inadvertently: Review your code to see if you're using caller or arguments properties anywhere and replace them with appropriate alternatives.
It's important to update your code to remove usage of caller and arguments properties as they are considered deprecated and may not be supported in future versions of JavaScript.
5. ReferenceError: reference to undefined property "x"
The "ReferenceError: reference to undefined property 'x'" indicates an attempt to access a property 'x' of an object that does not exist or has not been defined.
This JavaScript error commonly occurs when trying to access properties of an object that haven't been initialized or do not exist within the object. Double-check the object's structure and ensure 'x' is properly defined.
ReferenceError: reference to undefined property "x"
Why does this JavaScript error occur?
The error message ReferenceError: reference to undefined property 'x'
indicates an attempt to access a property 'x' on an object where the property does not exist or has not been defined.
Example:
var obj = {};
console.log(obj.x); // ReferenceError: reference to undefined property 'x'
To resolve this error:
Ensure the property 'x' is defined on the object: If you intend to access the property 'x' on an object, make sure it is properly defined on that object.
var obj = { x: 10 };
console.log(obj.x); // No error
Check if the object exists: The object containing the property 'x' has initialized and exists in the scope where you're trying to access it.
Verify spelling and case sensitivity: Double-check the spelling and case of the property name ('x' in this case) to ensure it matches the property you're intending to access.
Handle potential null or undefined values: If the object might be null or undefined, consider adding checks to handle these cases gracefully.
Remember to review your code and verify that the property 'x' is properly defined and accessible in the context where you're trying to access it.
Debug Your JavaScript Errors in Minutes
ReplayBird, a digital user experience analytics platform designed specifically to track JavaScript errors to revolutionize the debugging process by providing comprehensive insights into application errors.
ReplayBird records a comprehensive data set, including console logs, stack traces, network activity, and custom logs. With ReplayBird, diagnosing JavaScript exceptions becomes seamless. You can replay sessions, facilitating rapid debugging and resolution.
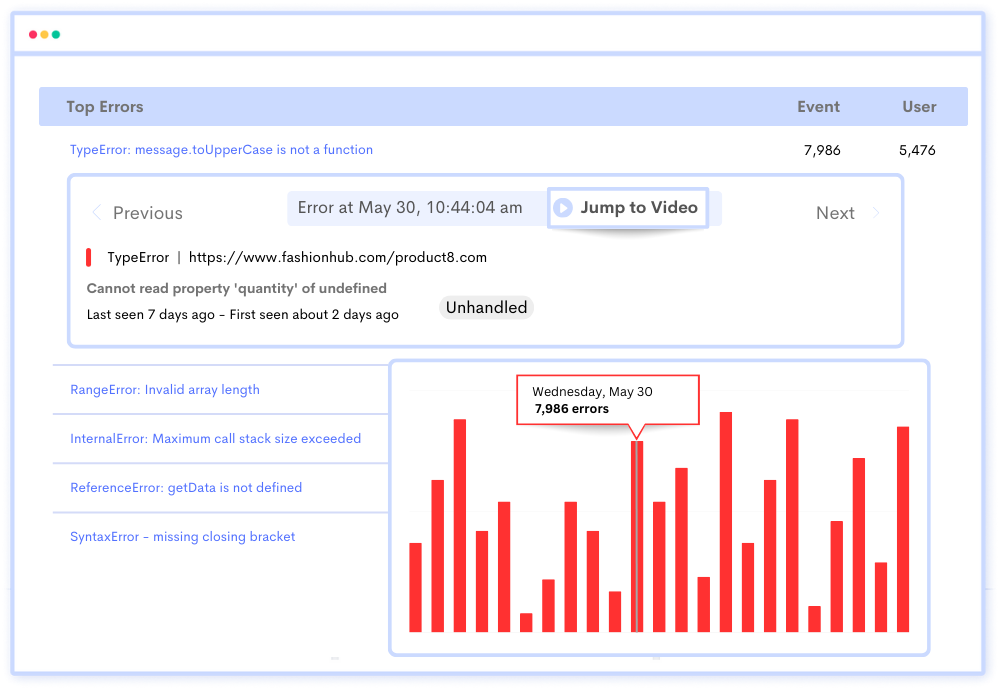
One key feature of ReplayBird is its ability to record and replay user sessions, allowing developers to recreate the exact sequence of events leading up to an error. This enables thorough debugging and facilitates understanding of the root cause of issues.
Additionally, ReplayBird's real-time error replay functionality enables developers to see errors as they occur in the user's browser, streamlining the debugging process and accelerating time to resolution.
Overall, ReplayBird's JavaScript error tracking enhances the debugging experience by providing actionable insights and facilitating efficient error resolution, ultimately leading to more robust and reliable applications.