SyntaxErrors in your code can result in a poor user experience, which underscores the importance of thorough testing, robust error handling, and continuous monitoring to ensure the reliability and usability of the users.
Before jumping our syntax error guide you can bookmark these other javaScript error blogs too:
- Javascript Errors: Permission, Recursion, URI, and Warnings (Part 1)
- RangeError, ReferenceError: Javascript Errors (Part 2)
- SyntaxError: JavaScript Errors (Part 3) - Currently Reading
- TypeError: JavaScript Errors (Part 4)
Imagine an e-commerce website where users can browse products, add them to their cart, and proceed to checkout. The checkout process involves multiple steps, including entering shipping details, selecting payment methods, and reviewing the order before finalizing the purchase. The backend of the website is implemented in Python, using various programming constructs.
Impact on User Experience:
- Failure to Load Checkout Page: If there's a syntax error in the code responsible for rendering the checkout page, users may encounter errors when attempting to proceed to checkout. Instead of seeing the checkout page, they might find a blank page, an error message, or unexpected behavior, preventing them from completing their purchase.
- Incorrect Price Calculation: Suppose there's a syntax error in the code that calculates the total price of the order. As a result, users may see incorrect prices displayed during the checkout process. This can lead to confusion, frustration, and mistrust, especially if users notice discrepancies between the displayed price and what they expected to pay.
- Inability to Submit Orders: If there's a syntax error in the code handling form submissions during the checkout process, users may encounter errors when attempting to submit their orders. Instead of successfully completing their purchase, they may find error messages or experience the submission process failing silently, leaving them unsure whether their order went through.
- Loss of User Data: In some cases, syntax errors may cause unexpected crashes or failures in the backend code, leading to data loss or corruption. For example, if there's a syntax error in the code responsible for storing order information in the database, users may experience issues such as orders not being recorded properly or order details getting lost during the checkout process.
- Negative Brand Perception: Users rely on e-commerce websites to provide a seamless and reliable shopping experience. When syntax errors occur frequently, users may perceive the website as unreliable or poorly maintained, leading to a negative perception of the brand and potentially discouraging them from returning to make future purchases.
Syntax Error Table of Content
What are Syntax Errors?
Syntax errors occur in programming when code violates the rules of a programming language's syntax. These errors prevent the program from being compiled or interpreted properly.
Common examples are missing parentheses, semicolons, quotation marks, and incorrect indentation. Syntax errors are typically detected by the compiler or interpreter during the code execution process, and they must be fixed before the program can run successfully.
Most Common Syntax Errors
#1 "use strict" not allowed in function with non-simple parameters
This syntax error occurs in JavaScript when the "use strict" directive is used within a function that has non-simple parameters.
SyntaxError: "use strict" not allowed in function with non-simple parameters
In JavaScript, use strict
applies stricter parsing and error handling, but it's not permitted within functions that have non-simple parameters, such as functions with default parameters or the rest parameters (...args). How to fix SyntaxError? - Ensure that "use strict" is placed at the beginning of the script or within functions that have only simple parameters.
#2 "x" is a reserved identifier
This syntax error occurs in JavaScript when the code attempts to use a variable or identifier named x
that is reserved by the language. In JavaScript, certain identifiers like class
, let
, const
, and function
are reserved for specific language constructs and cannot be used as variable names.
SyntaxError: "x" is a reserved identifier
Similarly, x
might be reserved in certain contexts, particularly when using strict mode or within certain libraries. How to fix SyntaxError? - Choose a different variable name that is not reserved by the language or any libraries you're using.
#3 JSON.parse
The error "JSON.parse: bad parsing" typically occurs when attempting to parse JSON data that doesn't adhere to the JSON syntax rules. JSON (JavaScript Object Notation) expects well-formed data, with correct formatting such as key-value pairs enclosed in curly braces {}
and values enclosed in double quotes ""
.
SyntaxError: JSON.parse
Common causes include missing or extra commas, unescaped special characters within strings, or improper nesting of objects or arrays.
To resolve this error, review the JSON data closely, ensuring it follows the correct structure. Using tools like JSON validators can help identify and fix parsing issues efficiently, ensuring the data is correctly formatted for parsing.
#4 Unexpected '#' used outside of class body
The syntax error "Unexpected '#' used outside of class body" indicates that a '#' symbol, typically used for private class fields or methods in certain programming languages like JavaScript or Python, is encountered outside the scope of a class definition. This error suggests that the '#' symbol is being used incorrectly, perhaps in a global scope or within a function where it's not allowed.
SyntaxError: Unexpected '#' used outside of class body
To resolve this error, ensure that the '#' symbol is only used within the body of a class definition for declaring private members, as per the language's syntax rules.
#5 Unexpected token
The SyntaxError occurs in JavaScript when an unexpected token is found during parsing, such as a symbol, keyword, or operator that is not valid in the current context. This happens really often, as it happens due to a typo, a missing or misplaced character, or incorrect syntax.
SyntaxError: Unexpected token
#6 Using //@ to indicate sourceURL pragmas is deprecated. Use //# instead
The SyntaxError is deprecated. Use //# instead" occurs in JavaScript when employing the deprecated syntax for specifying source URLs in the code. In modern JavaScript, the correct way to indicate source URLs is by using the //# syntax instead of //@.
SyntaxError: Using //@ to indicate sourceURL pragmas is deprecated. Use //# instead
This deprecation warning ensures compatibility with evolving JavaScript specifications and tools. To address this error, update the code to replace all instances of //@ with //# to align with current best practices and avoid potential issues in future JavaScript environments.
#7 a declaration in the head of a for-of loop can't have an initializer
The "SyntaxError: a declaration in the head of a for-of loop can't have an initializer" arises in JavaScript when attempting to initialize a variable within the head of a for-of loop. In JavaScript, the for-of loop syntax doesn't allow for variable declarations with initializers, such as "let" or "const", within its head.
SyntaxError: a declaration in the head of a for-of loop can't have an initializer
This restriction makes sure clarity and consistency in loop constructs, as it prevents potential confusion and unintended behavior. How to fix SyntaxError? - Initialize the variable outside the loop or use a traditional for loop if initialization within the loop header is necessary. Alternatively, refactor the code to accommodate the restrictions imposed by the for-of loop syntax.
#8 await is only valid in async functions, async generators and modules
The SyntaxError await is only valid in async functions, async generators, and modules" occurs in JavaScript when using the "await" keyword outside of an asynchronous function, asynchronous generator, or module context.
SyntaxError: await is only valid in async functions, async generators and modules
This error indicates that "await" can only be used within functions marked with the "async" keyword, async generators, or modules.
#9 continue must be inside loop
The SyntaxError occurs in programming languages like JavaScript when the "continue" statement is used outside of a loop construct. In JavaScript, "continue" is a statement that skips the current iteration of a loop and proceeds to the next iteration. However, it's only valid within loop structures such as "for", "while", or "do-while".
SyntaxError: continue must be inside loop
When encountered outside of these constructs, the interpreter raises a syntax error to indicate that "continue" cannot function as intended. How to fix SyntaxError? - Ensure that "continue" statements are placed within appropriate loop structures, allowing for the expected behavior of skipping iterations during looping processes.
#10 for-in loop head declarations may not have initializers
The "SyntaxError: for-in loop head declarations may not have initializers" occurs in JavaScript when attempting to initialize a variable within the head of a for-in
loop. This error suggests that the interpreter does not allow variable initialization within the loop declaration, as it expects only a variable declaration.
SyntaxError: for-in loop head declarations may not have initializers
For example, writing for (var i = 0 in object) { ... }
will prompt this error. To resolve this issue, initialize the variable before the loop or within the loop body rather than within the loop declaration itself. This error often arises due to confusion between the for-in loop
and other loop constructs in JavaScript.
#11 function statement requires a name
The "SyntaxError: function statement requires a name" occurs in JavaScript when attempting to declare an anonymous function using the function declaration syntax.
SyntaxError: function statement requires a name
This error suggests that the interpreter expects a function name after the function
keyword. For example, writing function() { ... }
instead of function functionName() { ... }
will prompt this error. Ensure to provide a name for the function when using function declarations to resolve this issue.
#12 getter and setter for private name #x should either be both static or n-static
The "SyntaxError: getter and setter for private name #x should either be both static or non-static" occurs in JavaScript when defining a getter or setter method for a private field with the #
prefix.
SyntaxError: getter and setter for private name #x should either be both static or n-static
This error indicates that the getter and setter methods must have consistent staticness; either both static or both non-static. Mixing static and non-static definitions for getter and setter methods of the same private field is not allowed.
To resolve this error, ensure that both the getter and setter methods for the private field are either declared as static or non-static. Consistency in the declaration of getter and setter methods maintains clarity and coherence in the codebase, facilitating better code organization and readability.
#13 label not found
The "SyntaxError: label not found" occurs in JavaScript when using a break
or continue
statement with a label that doesn't exist in the code. This error suggests that the specified label in the break
or continue
statement cannot be found within the code's context.
SyntaxError: label not found
#14 unlabeled break must be inside loop or switch
The "SyntaxError: unlabeled break must be inside loop or switch" occurs in JavaScript when a break
statement is used outside the context of a loop or switch statement and lacks a label.
SyntaxError: unlabeled break must be inside loop or switch
This error indicates that the interpreter expects the break
statement to be used within the body of a loop or switch statement.
To resolve this error, ensure that the break
statement is placed within the body of a loop or switch statement, or provide a label if it needs to break out of an outer nested loop or switch statement.
Expressions and Operators
#15 cannot use ?? unparenthesized within || and && expressions
The error "SyntaxError: cannot use ?? unparenthesized within || and && expressions" arises when attempting to use the nullish coalescing operator (??) without proper parentheses within logical expressions involving || (logical OR) or && (logical AND).
SyntaxError: cannot use ?? unparenthesized within || and && expressions
JavaScript requires explicit parentheses to clarify the precedence between logical operators and the nullish coalescing operator.How to fix SyntaxError? - Enclose the nullish coalescing operation within parentheses to specify its intended evaluation order within the logical expression. This ensures clarity and avoids ambiguity in parsing. For instance, (a || b) ?? c or a || (b ?? c).
#16 applying the 'delete' operator to an unqualified name is deprecated
The error "SyntaxError: applying the 'delete' operator to an unqualified name is deprecated" indicates an attempt to use the delete operator with an unqualified name, which is no longer supported and has been deprecated.
SyntaxError: applying the 'delete' operator to an unqualified name is deprecated
In JavaScript, the delete operator is typically used to remove properties from objects. However, using it on unqualified names, such as variables declared with var, let, or const, is deprecated because it can lead to unexpected behavior and does not achieve the intended purpose.
How to fix SyntaxError? - Review the code and ensure that the delete operator is used appropriately with object properties rather than with variables or other unqualified names.
If you intended to remove a variable from the scope, consider using proper scoping techniques or reevaluating the need for dynamic variable management.
#17 test for equality (==) mistyped as assignment (=)
The error "SyntaxError: test for equality (==) mistyped as assignment (=)" occurs when a comparison operation using the equality operator == is mistakenly written as an assignment operation using the assignment operator =. This is a common error that can lead to unintended behavior in code.
SyntaxError: test for equality (==) mistyped as assignment (=)
For example, writing if (x = 10) instead of if (x == 10) will assign the value 10 to variable x and always evaluate to true, regardless of the original value of x.
How to fix SyntaxError? - Review the affected code and ensure that comparison operations use the equality operator == for comparison, while assignment operations use the assignment operator = for variable assignment.
#18 unparenthesized unary expression can't appear on the left-hand side of ''**
The error "SyntaxError: unparenthesized unary expression can't appear on the left-hand side of '**'" occurs when using the exponentiation operator (**) in combination with a unary expression on its left-hand side without parentheses.
SyntaxError: unparenthesized unary expression can't appear on the left-hand side of ''**
For example, writing -x ** 2 without parentheses around -x triggers this error. How to fix SyntaxError? - Enclose the unary expression in parentheses to explicitly define the precedence, ensuring that it's evaluated before applying the exponentiation operator.
For instance, (-x) ** 2 would correctly calculate the square of the negative value of x.
Variables and Declarations
#19 missing ) after argument list
The "SyntaxError: missing ) after argument list" occurs when a closing parenthesis is omitted after a function's arguments. This commonly happens when defining or calling a function.
SyntaxError: missing ) after argument list
For example, in Python, if you have a function call like print("Hello, world!", without the closing parenthesis ), this error will be raised. It's crucial to ensure that all opening parentheses have corresponding closing parentheses to avoid this syntax error.
#20 missing ) after condition
The "SyntaxError: missing ) after condition" typically arises in languages like Python and JavaScript when a closing parenthesis is omitted after a condition within a control structure such as an if statement or a loop.
SyntaxError: missing ) after condition
This error suggests that the interpreter encountered a situation where it expected a closing parenthesis to close off a condition expression, but it was missing.
For instance, in Python, writing if x > 5: without completing the condition with a closing parenthesis can trigger this error. It's essential to ensure proper syntax by including all necessary parentheses to close off expressions in control structures.
#21 missing
The "SyntaxError: missing )" indicates that a closing parenthesis is expected but not found. It usually occurs after conditions or function calls.
For instance, if x > 5: without a closing parenthesis will prompt this error. Always make sure you have a proper syntax by closing all parentheses where required.
SyntaxError: missing
#22 missing ; before statement
The "SyntaxError: missing ; before statement" typically appears in languages like JavaScript or C/C++ when a semicolon is expected to terminate a statement but is absent.
SyntaxError: missing ; before statement
This error suggests that the interpreter encountered a situation where it expected a semicolon to mark the end of a statement, but it was missing.
For example, in JavaScript, writing var x = 5 without terminating it with a semicolon can trigger this error. You can avoid this by adding semicolons where necessary to end statements.
#23 missing = in const declaration
The "SyntaxError: missing = in const declaration" occurs in JavaScript when declaring a constant variable (const) without assigning it a value. This error indicates that the interpreter expects an assignment operator (=) to initialize the constant with a value, but it's missing.
SyntaxError: missing = in const declaration
For instance, writing const x; instead of const x = 5; will prompt this error. Constants must be initialized upon declaration in JavaScript, so ensure proper syntax by assigning a value to the constant when declaring it.
#24 missing ] after element list
The "SyntaxError: missing ] after element list" indicates a missing closing square bracket (]) in an array declaration in JavaScript. This error suggests that the interpreter expects a closing square bracket to mark the end of the array's elements but did not find it.
SyntaxError: missing ] after element list
For example, [1, 2, 3 instead of [1, 2, 3] will prompt this error. You can avoid this syntaxerror by closing arrays with ] after the element list.
#25 missing formal parameter
The "SyntaxError: missing formal parameter" occurs in JavaScript when defining a function without specifying formal parameters. This error suggests that the interpreter expects a list of parameters within the parentheses of the function declaration, but it's empty.
SyntaxError: missing formal parameter
For instance, function myFunction() { ... } without specifying parameters like function myFunction(parameter1, parameter2) { ... } will prompt this error. You can provide formal parameters when defining functions to avoid this syntax error.
#26 missing name after . operator
The "SyntaxError: missing name after . operator" typically occurs in languages like JavaScript when a dot (.) operator is used without specifying a property or method name after it. This error suggests that the interpreter expects a valid identifier to follow the dot operator, indicating the property or method accessed on an object.
SyntaxError: missing name after . operator
For example, writing object. without specifying a property or method name like object.property or object.method() will prompt this error. Ensure proper syntax by specifying a valid property or method name after the dot operator to access object properties or methods.
#27 missing variable name
The "SyntaxError: missing variable name" arises in JavaScript when attempting to declare a variable without specifying its name. This error indicates that the interpreter expects an identifier to represent the variable being declared, but it's absent.
SyntaxError: missing variable name
For example, writing var ; instead of var x; will prompt this error. Ensure proper syntax by providing a valid variable name after the var, let, or const keyword when declaring variables in JavaScript.
#28 missing } after function body
The "SyntaxError: missing } after function body" occurs in languages like JavaScript when a closing curly brace (}) is omitted after the body of a function. This error indicates that the interpreter expects a closing curly brace to terminate the function definition, but it's missing.
SyntaxError: missing } after function body
For example, failing to include } at the end of a function definition like function myFunction() { ... will prompt this error. Ensure proper syntax by closing function bodies with } to avoid this error.
#29 missing } after property list
The "SyntaxError: missing } after property list" typically occurs in JavaScript when an object literal is not properly closed with a closing curly brace (}). This error indicates that the interpreter expects a closing curly brace to mark the end of the object literal's property list, but it's missing.
SyntaxError: missing } after property list
For instance, writing { name: "John", age: 30 without closing with } will prompt this error. You can avoid this syntax error by closing object literals with } after the property list.
#30 redeclaration of formal parameter "x"
The "SyntaxError: redeclaration of formal parameter 'x'" arises in JavaScript when attempting to declare a parameter with the same name within the parameter list of a function. This error indicates that the interpreter recognizes an attempt to declare a parameter that has already been declared for the same function.
SyntaxError: redeclaration of formal parameter "x"
For example, writing function myFunction(x, x) { ... } will prompt this error. JavaScript does not allow multiple parameters with the same name within a function's parameter list. To resolve this error, ensure that each parameter in the function declaration has a unique name, or refactor the function to use a different approach that does not require duplicate parameter names.
#31 return not in function
The "SyntaxError: return not in function" occurs in JavaScript when a return statement is encountered outside the body of a function. This error indicates that the interpreter expects the return statement to be inside a function definition.
SyntaxError: return not in function
Strings and Literals
#32 identifier starts immediately after numeric literal
The error "SyntaxError: identifier starts immediately after numeric literal" indicates a JavaScript syntax issue where an identifier (variable name) follows a numeric literal without any separation. This commonly happens when a number is followed directly by letters or underscores, violating JavaScript's syntax rules.
SyntaxError: identifier starts immediately after numeric literal
How to fix SyntaxError? - Ensure there's a valid separator (such as a space) between the numeric literal and the identifier, or consider using a different naming convention that adheres to JavaScript's syntax requirements.
#33 illegal character
The error "SyntaxError: illegal character" occurs when the JavaScript parser encounters a character that is not allowed in the code. This commonly happens due to typos, unrecognized symbols, or stray characters such as invisible control characters.
How to fix SyntaxError? - Carefully review the code to identify and remove the illegal character causing the error.
SyntaxError: illegal character
#34 invalid BigInt syntax
The error "SyntaxError: invalid BigInt syntax" occurs when attempting to use BigInt syntax that does not adhere to the correct format. BigInt literals must be followed by "n" (e.g., 123n).
Ensure that BigInt literals are properly formatted, with an integer followed by "n", to resolve this error.
SyntaxError: invalid BigInt syntax
#35 invalid assignment left-hand side
The error "SyntaxError: invalid assignment left-hand side" indicates an issue with the syntax of an assignment operation. This error typically occurs when the left-hand side of the assignment does not represent a valid target for assignment.
SyntaxError: invalid assignment left-hand side
It might happen due to attempting to assign a value to a read-only property, using an invalid variable name, or omitting the assignment target altogether.
How to fix SyntaxError? - Ensure that the left-hand side of the assignment operation is a valid target for assignment, such as a variable, property, or element of an array or object. Double-check variable declarations and property accesses for correctness.
#36 invalid regular expression flag "x"
The error "SyntaxError: invalid regular expression flag 'x'" occurs when using an unsupported or invalid flag in a regular expression literal. Regular expression flags, such as 'g', 'i', or 'm', control how the matching behavior occurs.
SyntaxError: invalid regular expression flag "x"
If 'x' or any other unsupported flag is used, it results in a SyntaxError. How to fix SyntaxError? - Review the regular expression and ensure that only valid flags are used. Check for typos or incorrect flag usage, and remove or replace the invalid flag with one that is supported by JavaScript's regular expression engine.
#37 unterminated string literal
The error "SyntaxError: unterminated string literal" indicates that a string declaration has started but has not been properly closed with a closing quotation mark. This often occurs when a string spans multiple lines or when the closing quotation mark is accidentally omitted.
SyntaxError: unterminated string literal
How to fix SyntaxError? - Check the string declaration for missing or misplaced quotation marks and ensure that all strings are properly terminated. If the string spans multiple lines, consider using string concatenation or template literals ( ) to ensure correct parsing and termination.
Keep Reading more on JavaScript Errors
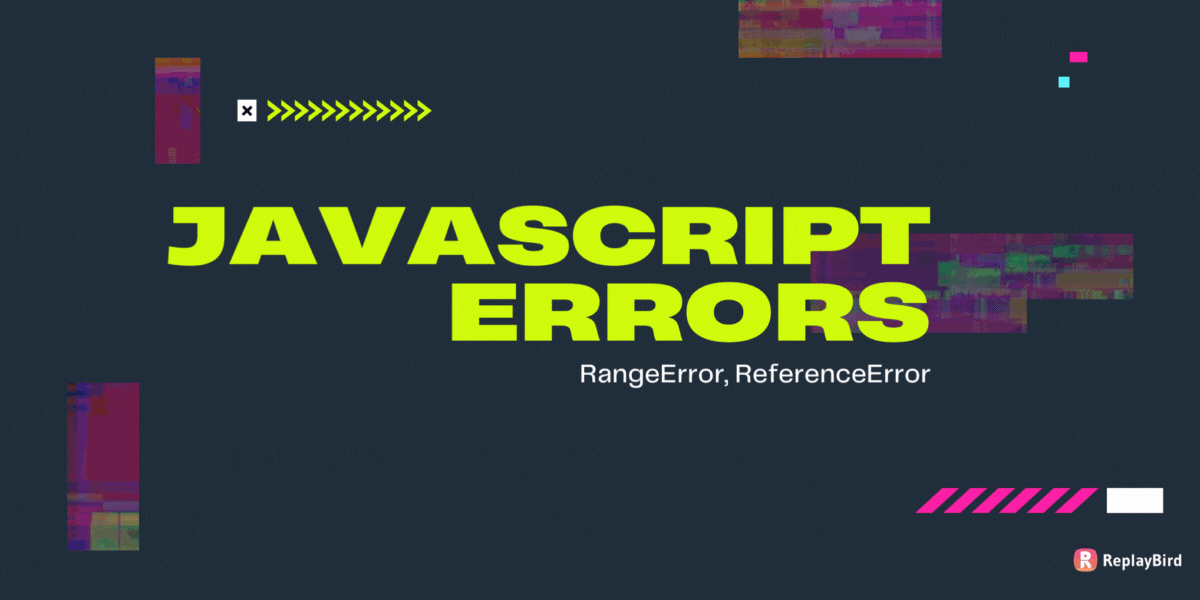
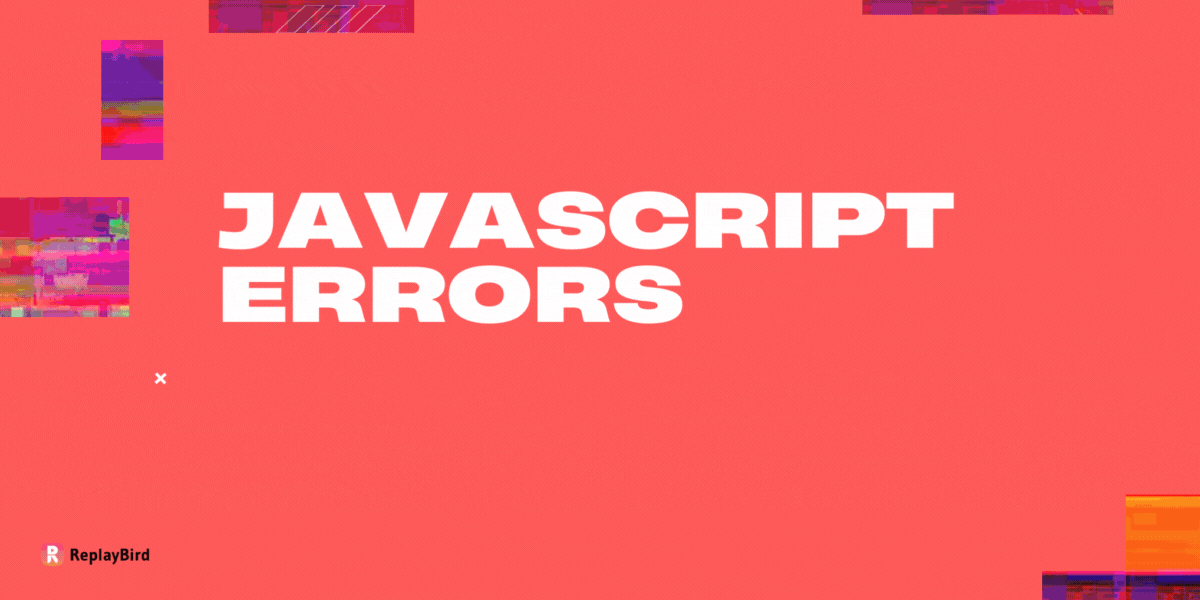
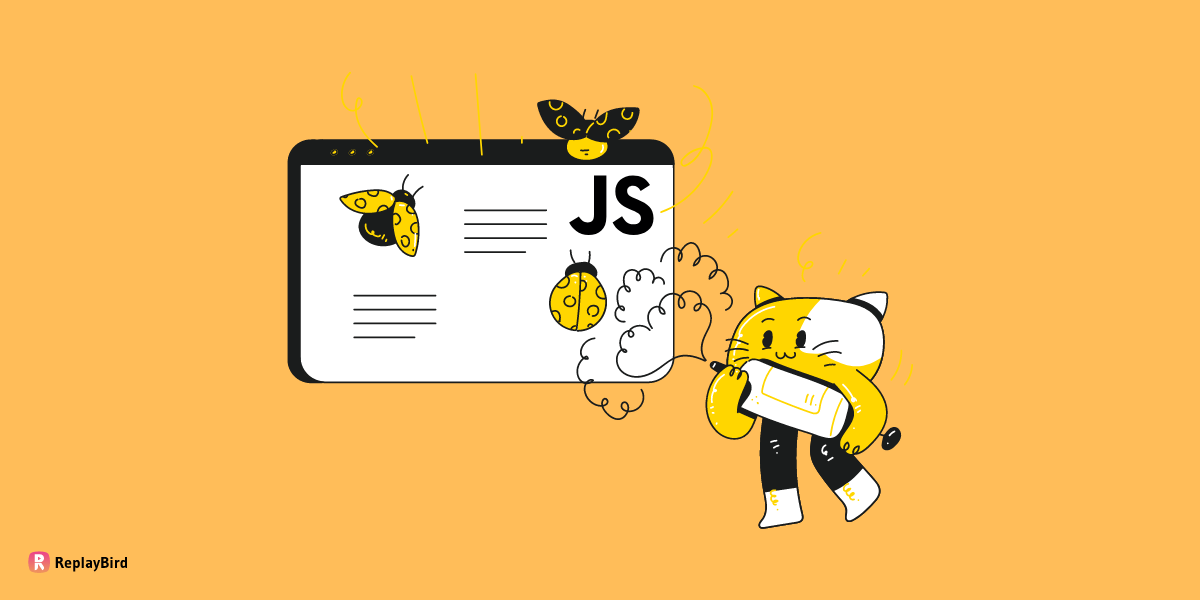