LocalStorage API in JavaScript, a game-changer for seamlessly storing and retrieving data on a user's browser. Whether you're building a dynamic web application or a snappy interactive website, control the capabilities of LocalStorage can significantly boost user experience.
Imagine the frustration of unintentionally closing a webpage while in the midst of filling out a crucial form. Suddenly, all your progress disappear, and you're left with the redoing task of starting from scratch. It's a scenario that's all too common for web users.
However, there's a solution to this exasperating problem. By using JavaScript's localStorage
, you can make sure that your data persists even beyond a single browsing session. In this blog we will go all the aspects of using localStorage API in JavaScript.
- What is localStorage in JavaScript?
- How to Store JavaScript API Data in Localstorage?
- localStorage vs sessionStorage vs cookie
- localStorage API in JavaScript - Methods
- LocalStorage API in JavaScript - Practical Example
- Advantages
- Limitations
- Browser Support
What is localStorage in JavaScript?
LocalStorage
in JS is like a small storage space right in your web browser. It allows websites to store information that stays even when you close your browser or turn off your computer. This means that the next time you visit that website, it can remember things about you, like your preferences or settings.
For example, imagine you're using a shopping website. With JavaScript localStorage
api, the website can remember what you added to your cart, so even if you leave and come back later, your items are still there waiting for you.
So, in a nutshell, localStorage
is one of those behind-the-scenes things that make the web work so well, as it remembers things about you and makes your browsing experience smoother and more personalized.
What is window.localStorage?
window.localStorage
is super handy for websites because they can use it to remember things about you.
Let's say you're customizing the look of a website or saving items in your shopping cart. window.localStorage
helps the website remember your choices, so the next time you visit, everything's just the way you left it.
In JavaScript, localStorage
is a property of the Window
interface as it allows you to store key-value pairs in a web browser's local storage, which persists even after the browser is closed.
So, when you use localStorage
in your JavaScript code, you're essentially accessing the localStorage
property of the window
object. The two terms can be used interchangeably.
Technically, localStorage
is an instance of the Web Storage API, which is part of the Document Object Model (DOM) interface. It operates as a set of methods and properties accessible through JavaScript.
Where is localStorage stored?
Now, where does this JavaScript localStorage
api actually gets stored? Well, it's right there in your browser! It's not out in the open for everyone to see; it's private, just for you.
You might want to know, "What if I close my browser or turn off my computer? Will my stuff still be there?" The answer is, yes! Even if you shut everything down and come back later, your stored data will still be there in the localStorage
.
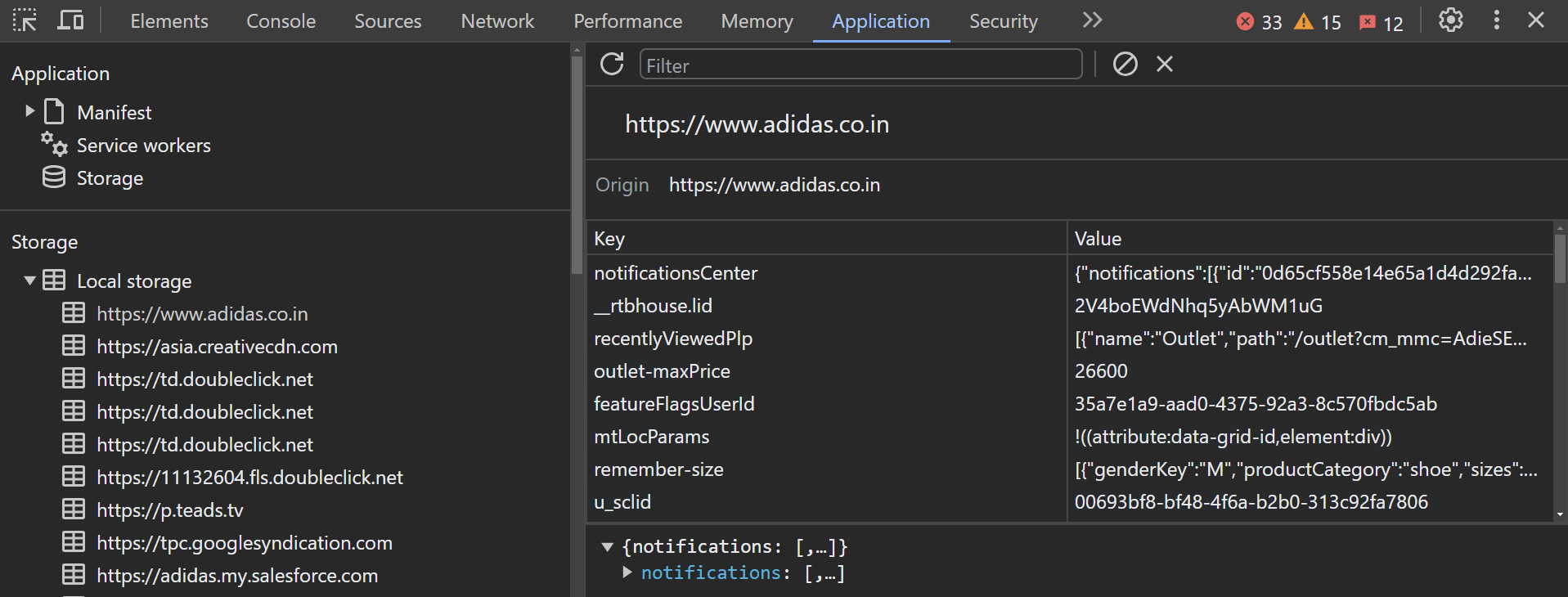
- Step 1: Open Developer Tools
- Step 2: Click on the "Application" or "Storage" tab
- Step 3: Click on "Local Storage" to expand and view the list of websites that have stored data.
- Step 4: Select the Website
- Step 5: Click on it to view the key-value pairs (data) stored by that website in localStorage.
You should see the items you added to the cart displayed in key-value pairs. The keys represent the names of the items, and the values are their corresponding details.
This localStorage
is specific to your browser and your device. So, if you save something on your computer using Chrome, it won't be in the same spot if you switch to Firefox. Each browser has its own localStorage
.
While it's a safe and convenient spot, it's not infinite. There's a limit to how much it can hold, so it's best for storing smaller bits of information, like preferences or settings.
How to Store JavaScript API Data in Localstorage?
To store JavaScript API data in the browser's localStorage
, you need to follow these general steps:
- Fetch the data from the JavaScript API.
- Convert the data to a string (usually JSON format) because
localStorage
can only store strings. - Store the stringified data in
localStorage
. - Optionally, you may want to parse the stored data back to its original format when retrieving it from
localStorage
.
// Fetch data from API
fetch('https://api.example.com/data')
.then(response => response.json()) // Assuming JSON response, parse it
.then(data => {
// Convert data to string (stringify)
const jsonData = JSON.stringify(data);
// Store data in localStorage
localStorage.setItem('apiData', jsonData);
})
.catch(error => {
console.error('Error fetching data:', error);
});
Later, when you want to retrieve the data:
// Retrieve data from localStorage
const storedData = localStorage.getItem('apiData');
if (storedData) {
// Parse the stored data back to its original format
const parsedData = JSON.parse(storedData);
// Now you can use the parsed data
console.log(parsedData);
} else {
console.log('No data found in localStorage.');
}
- As JavaScript localstorage api can only store strings. So, you need to serialize (e.g., using JSON.stringify) your data before storing it and deserialize it (e.g., using JSON.parse) when retrieving it.
- Data stored in
localStorage
is scoped to the origin of the page. Each origin gets its own separate storage. - The data persists even after the browser is closed and reopened, until explicitly cleared by the user or by your script.
Why to use a localStorage?
Here are five reason why you may need to use localStorage
API in JS.
-
Remembering User Preferences:
Use
localStorage
when you want your website to remember user-specific settings, like theme choices or language preferences. This way, when the user returns to your website, their preferences are remembered. -
Storing Shopping Cart Items:
localStorage
is perfect for e-commerce sites as it helps retain items in the shopping cart even if the user navigates away or returns later, enhancing the shopping experience. -
Constant Login Sessions:
localStorage
is also used to remember logged-in states as users won't need to log in every time they visit your site, making for a smoother experience. -
Caching Data for Offline Use:
When building progressive web apps,
localStorage
allows you to store essential data locally to make sure that your app functions even without an internet connection, improving user convenience. -
Reducing Server Requests:
In browser-based games, localStorage can be used to save the player's progress as it optimize performance by using
localStorage
to store frequently accessed data. This minimizes server requests, leading to faster load times and a concise user experience.
localStorage vs sessionStorage vs cookie
We've got three storage tools in our web development toolkit: localStorage
, sessionStorage
, and cookies.
#1 localStorage:
Think of localStorage
as a long-term data storage for your browser. It is a smaller storage unit where you can keep data and they'll be there even after you close your browser and come back later.
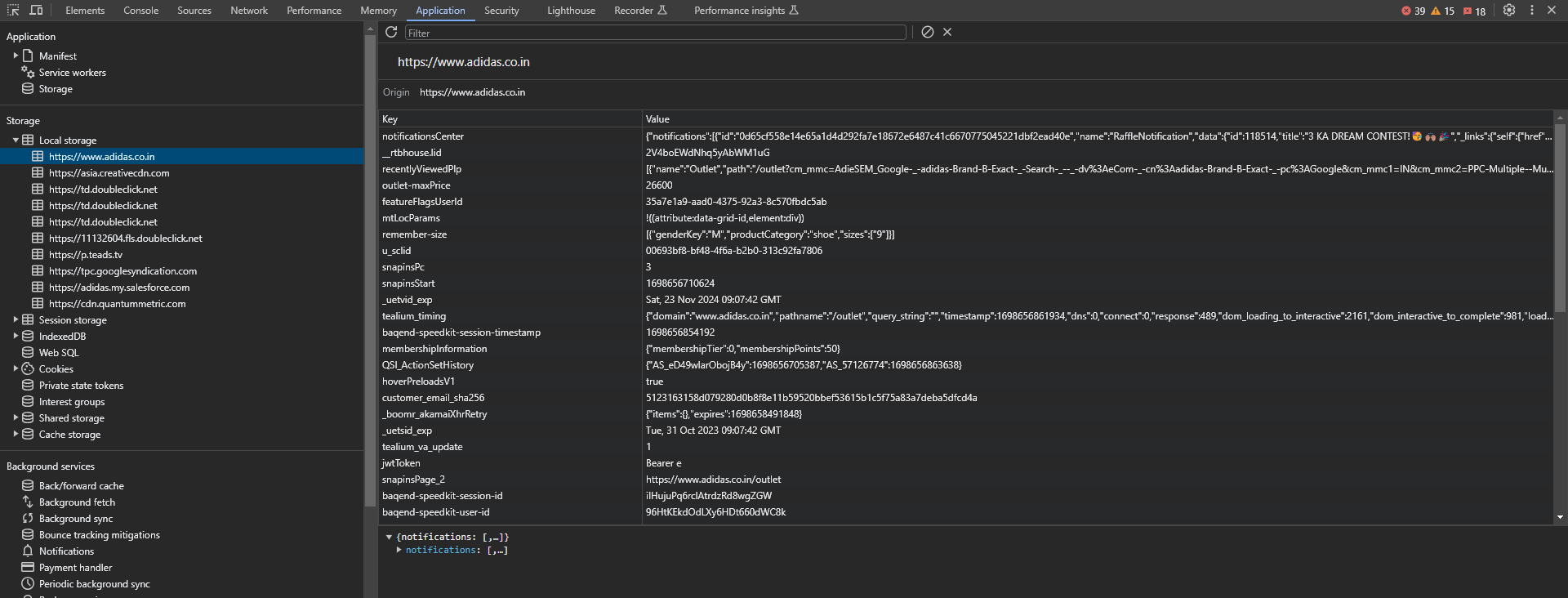
Best for storing information you want to keep for a long time, like user preferences or settings.
#2 sessionStorage:
sessionStorage
a web storage feature in JavaScript that allows you to store key-value pairs on the client side. It's handy for temporary storage that you only need while you're on a specific page. Once you close the browser tab or window, it refreshes.
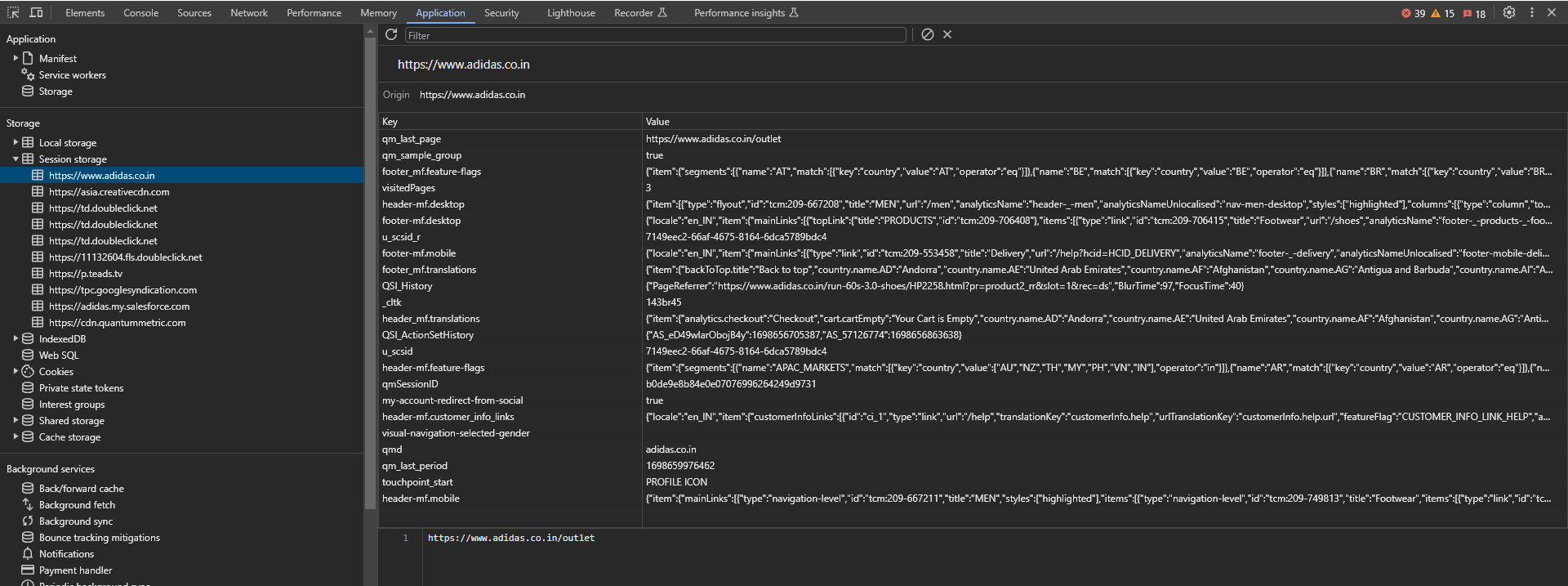
Best for keeping data around for a single browsing session, like form data or temporary preferences.
#3 Cookies:
Cookies are some data that a website leaves on your computer. They're used for various purposes, like remembering login status or tracking user behavior. They can be set to expire after a certain time.
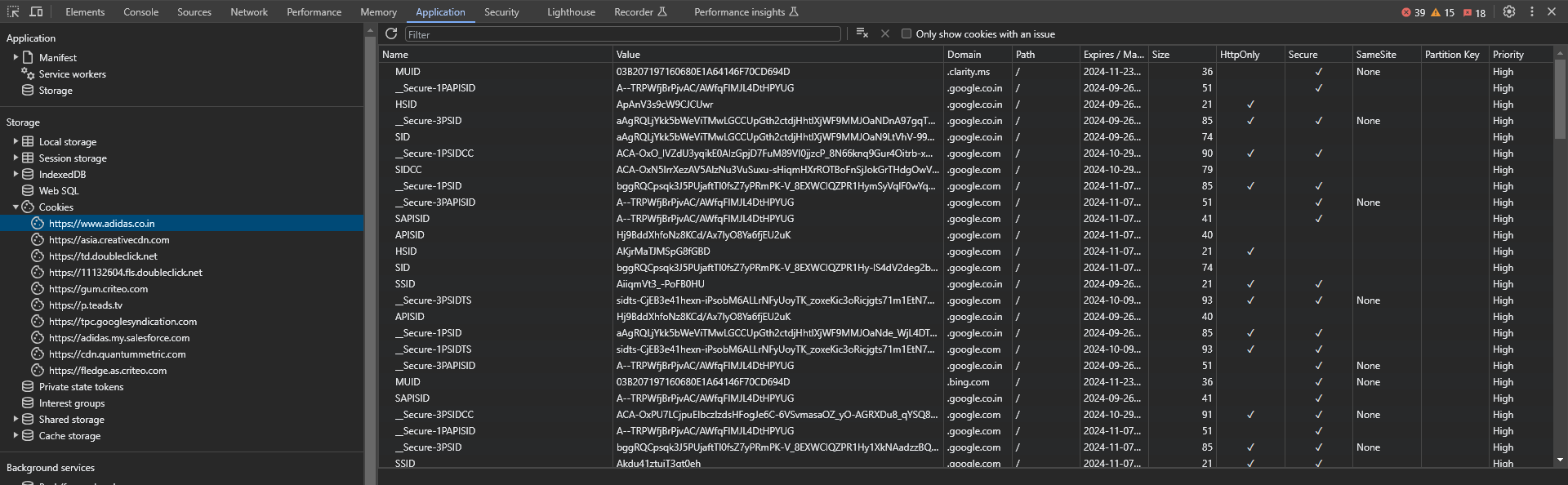
Best for storing small pieces of information that need to be sent back to the server with each request, like authentication tokens.
Here's a detailed comparison of localStorage
, sessionStorage
, and cookies in a tabular format:
localStorage API in JavaScript - Methods
There are five primary methods for interacting with localStorage
. These key()
method is used to recall the name of a key associated with a specific index.
This method is best in web development for managing user-specific preferences, settings, and other data that needs to persist across browser sessions.
1. setItem()
- Storing data
setItem()
is like placing your favorite items into a special box called localStorage
. This method allows you to store data in the browser, so even if you close it or come back later, your items will still be there. It's super handy for remembering user preferences, settings, or any information you want to keep safe and easily accessible.
Syntax:
localStorage.setItem('key', 'value');
In this syntax, 'key'
is like a label for your item, and 'value'
is the actual data you want to store. For example, localStorage.setItem('username', 'JohnDoe');
will save the username "JohnDoe" in localStorage
.
Example:
Consider you're building a robust customer management system. With localStorage
and setItem()
, you can enhance the user experience. For instance, you want to remember how the user prefers to view their customer data.
By using the code:
localStorage.setItem('customers.columns', JSON.stringify({
columns: ['full_name', 'phone_number', 'email', 'title', 'company', 'deal_amount'],
sort_by: 'deal_amount'
}));
Here's what happens:
Column Preferences:
- The code saves the user's chosen columns (
full_name
,phone_number
,email
, etc.) to display in the customer list. So, every time they return, the system remembers their preferred view.
Sorting Criteria:
- It also stores the sorting preference based on the
deal_amount
. This ensures that customer data is initially sorted by deal amount, which might be crucial for business decisions.
This means that even if the user refreshes the page or comes back later, their chosen columns and sorting order are retained. It's like having a personalized dashboard that adapts to their needs! This advanced use of setItem()
greatly enhances the user's efficiency and satisfaction.
2. getItem()
- Retrieving data
getItem()
method allows you to retrieve information, like user preferences or settings, from your browser.
Syntax:
let language = localStorage.getItem('key');
You use 'key'
to tell the browser exactly which data you want to retrieve.
Example:
Let's say you have a language learning app. You want to remember which language the user chose for their lessons. getItem()
is just to help you fetch that language choice from localStorage
so the app can start in the right language every time.
First you need to set item as you need to store the memory of the language.
localStorage.setItem('language', 'english')
let columnStr = localStorage.getItem('language');
let columns = JSON.parse(columnStr)
And then we're telling the browser to fetch the value associated with the key language
. This way, the app knows which language to get and use for the lessons.
3. removeItem()
- Deleting data
removeItem()
as your way of tidying up that localStorage
to clear out this specific data stored. This method simple when you want to remove a specific piece of information you no longer need stored.
Syntax:
localStorage.removeItem('key');
You just replace 'key'
with the name of the data you want to remove.
Example:
Imagine you have a to-do list app. When you complete a task, you want to remove it from the list. removeItem()
helps you clear out that specific task from localStorage
, keeping your list organized.
localStorage.removeItem('completedTask1');
In this example, we're letting the browser to remove the data associated with the key 'completedTask1'
.
4. clear()
- Delete all items
localStorage.clear()
is not like removeItem()
removing only specific data, but it clean-up whole local storage in your browser and starts with fresh storage as it deletes everything stored in it localStorage
, giving you a clean and tidy local storage.
Syntax:
localStorage.clear();
Example:
Imagine you have a game with multiple levels. Once a player completes all the levels, you want to reset the game for a new player. localStorage.clear()
wipes out all the level progress, so the next player can start fresh.
localStorage.clear();
In this example, we're telling the browser to clear out everything in localStorage
.
5. key: key()
- Find data name
localStorage.key()
helps you find out the names of all the data stored inside. This method makes it simple for you to know what's in your storage without actually taking everything out.
Syntax:
let keyName = localStorage.key(index);
You provide an index
to this method, and it gives you back the name of the data at that index.
Example:
Let's say you have a note-taking app, and you want to show users a list of all the notes they've saved. localStorage.key()
provides you with the names of the saved notes, so you can display them in a neat list.
let firstKeyName = localStorage.key(0);
In this example, we're asking the browser to give us the name of the first data stored in localStorage
.
LocalStorage API in JavaScript - Practical Example
Let's create a simple note-taking app that allows users to add, edit, and delete notes, and uses localStorage
to persist the notes even after the page is refreshed or closed.
<body>
<h1>Enter Your Note Here</h1>
<input type="text" id="newNote" placeholder="Enter a new note">
<button onclick="addNote()">Add Note</button>
<ul id="noteList"></ul>
<script>
// Function to add a note
function addNote() {
var noteInput = document.getElementById('newNote');
var noteText = noteInput.value;
if (noteText.trim() !== '') {
var noteList = document.getElementById('noteList');
var listItem = document.createElement('li');
listItem.textContent = noteText;
noteList.appendChild(listItem);
// Save the notes to localStorage
var notes = JSON.parse(localStorage.getItem('notes')) || [];
notes.push(noteText);
localStorage.setItem('notes', JSON.stringify(notes));
// Clear the input field
noteInput.value = '';
}
}
// Function to load notes from localStorage
function loadNotes() {
var noteList = document.getElementById('noteList');
var notes = JSON.parse(localStorage.getItem('notes')) || [];
notes.forEach(function(noteText) {
var listItem = document.createElement('li');
listItem.textContent = noteText;
noteList.appendChild(listItem);
});
}
// Load notes when the page loads
window.onload = loadNotes;
</script>
</body>
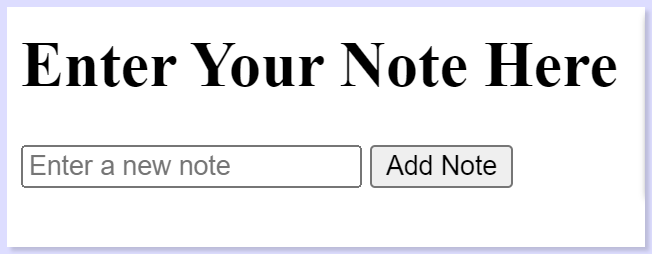
In this example, we have a simple HTML page with an input field to add notes, a button to add notes, and an unordered list to display the notes. The JS code handles adding notes to the list and saving them to localStorage
.
Here's how it works:
- When you enter a note and click "Add Note," the
addNote
function is called. - It gets the note text from the input field, creates a new list item, and appends it to the unordered list.
- It then retrieves the current list of notes from
localStorage
(if any) or creates an empty array if there are no notes yet. It then pushes the new note to this array and saves it back tolocalStorage
.
Additionally, when the page loads, the loadNotes
function is called. This function retrieves the notes from localStorage
and populates the list with them.
This example demonstrates a basic use of localStorage
in a simple note-taking application. It allows notes to be added and stored locally, even after the page is refreshed or closed.
Advantages of using localStorage API in JavaScript
The localStorage
API in JavaScript provides a stronger client-side storage solution which boost the functionality, performance, and user experience of your web applications.
- Speedy Storage: Data retrieval and storage are faster compared to server-based alternatives as
localStorage
API in JavaScript imporves user experience with swift data access and updates. - Steady Data: Information remains even after browser or device is closed or restarted as it allows for continuity and personalized experiences across browsing sessions.
- Client-Side: Operates entirely on the client's device, reducing server load and latency as
localStorage
API in JavaScript frees up server resources, leading to smoother web applications. - Ample Capacity: Provides more storage space compared to cookies for larger datasets as it accommodates complex applications without compromising performance.
Limitations of using localStorage API in JavaScript
While localStorage
is a valuable tool for client-side storage, it does come with its set of limitations. These factors can influence the effectiveness of using localStorage in certain scenarios.
- Storage Capacity: Limited to about 5-10 MB per domain, potentially insufficient for large-scale applications which may lead to data truncation or loss if storage limit is reached.
- Lack of Server Interaction:
localStorage
cannot communicate directly with servers, limiting dynamic data updates. Real-time updates may require additional server-side synchronization efforts. - No Expiry Control: Data in
localStorage
remain indefinitely, potentially leading to outdated information which requires manual clearing or refreshing strategies to manage data expiration. - Single Origin Policy:
localStorage
API in JavaScript is limited to one origin (protocol, domain, and port). Cross-origin sharing oflocalStorage
data is not allowed for security reasons. - Limited Data Format: Only strings can be stored, requiring serialization and deserialization for complex data which adds complexity when working with non-string data types in
localStorage
API in JavaScript.
Security limitations of localStorage
API in JavaScript
localStorage
is accessible by any script from the same origin (meaning, same protocol, domain, and port). This means if there's a security vulnerability in a website, it's possible for malicious scripts to access or modify localStorage
data.
So, while it's great for storing non-sensitive preferences and settings, it's not the place for highly confidential information like passwords or sensitive user data. For that, server-side storage with proper encryption and security measures will be preferable.
Browser Support
The JavaScriptlocalStorage API is widely supported across modern browsers, making it a reliable choice for client-side storage in web development. Here's a list of browsers and their versions that support localStorage:
- Google Chrome: Supported in all versions.✅
- Mozilla Firefox: Supported in all versions.✅
- Apple Safari: Supported in all versions.✅
- Microsoft Edge: Supported in all versions.✅
- Opera: Supported in all versions.✅
- Internet Explorer: Supported in IE8 and later versions.⛔
You can verify if the browser supports localStorage
by using the following code snippet:
if (typeof(Storage) !== "undefined") {
console.log('this browser has localstorage support');
} else {
console.log('localstorage not supported for this browser');
}
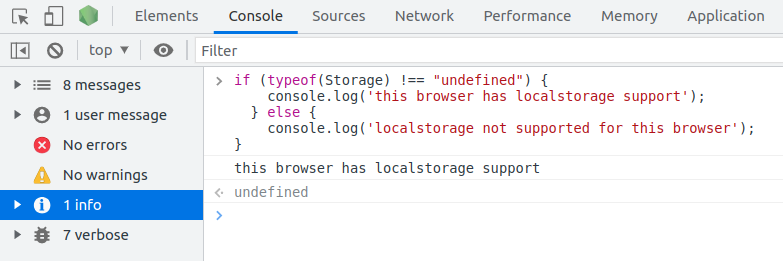
Conclusion:
At its core, localStorage is a key-value storage mechanism provided by modern web browsers as it allows developers to store data on the client-side withstand across browser sessions and even computer restarts.
In JavaScript, this functionality is accessed through the Window.localStorage
property, providing a straightforward interface to work with.
Through this article, we have not only learnt how to use localStorage effectively, but also delved into the fundamental concepts of web storage in JavaScript.
By the end, you'll have a comprehensive understanding of how to increase this tool to boost user experiences and prevent data loss.
Understand Exactly How Your Users Interact with Your app
ReplayBird, a digital user experience analytics platform designed specifically for developers with advanced insights to optimize your applications like a pro!
Unleash the power of behavioral insights with ReplayBird's intuitive heatmaps, session replays, and clickstream analysis allows you to visualize user behavior, identify popular elements, and detect pain points that might hinder user satisfaction.
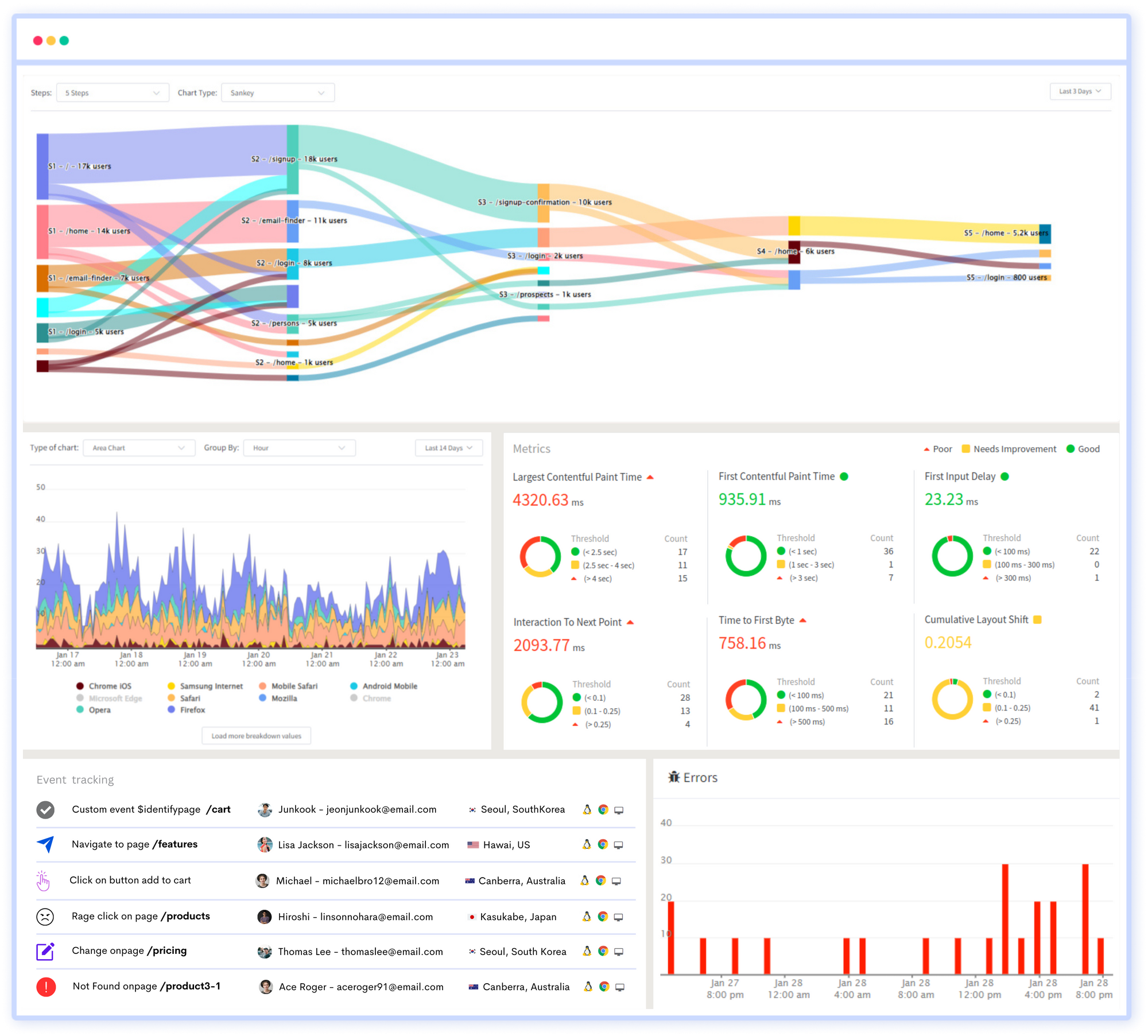
Customer journey analysis and conversion funnels of ReplayBird to analyze deeper into user journeys, identify where drop-offs occur, and uncover conversion blockers.
Troubleshooting is now simpler with ReplayBird's robust debugging features. Detect and diagnose UX issues quickly, ensuring a seamless user journey from start to finish.
With ReplayBird, you have the ultimate toolkit to elevate your projects to the next level. The platform empowers you to create high-performing, user-centric applications that leave a lasting impression.
Try ReplayBird 14-days free trial
Keep reading more about JavaScript:
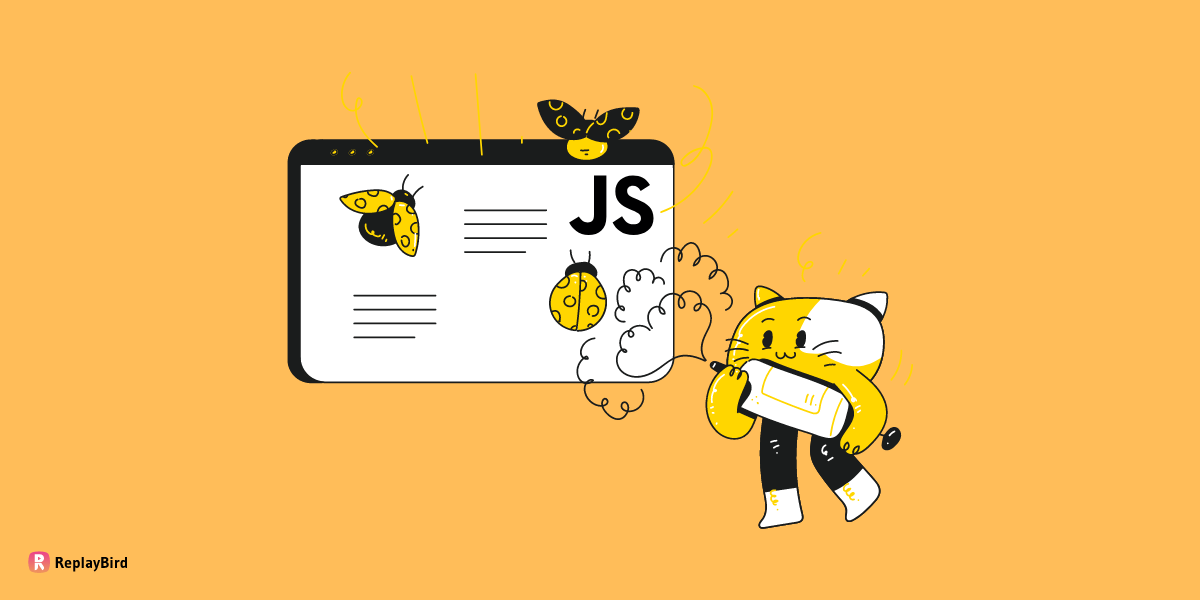
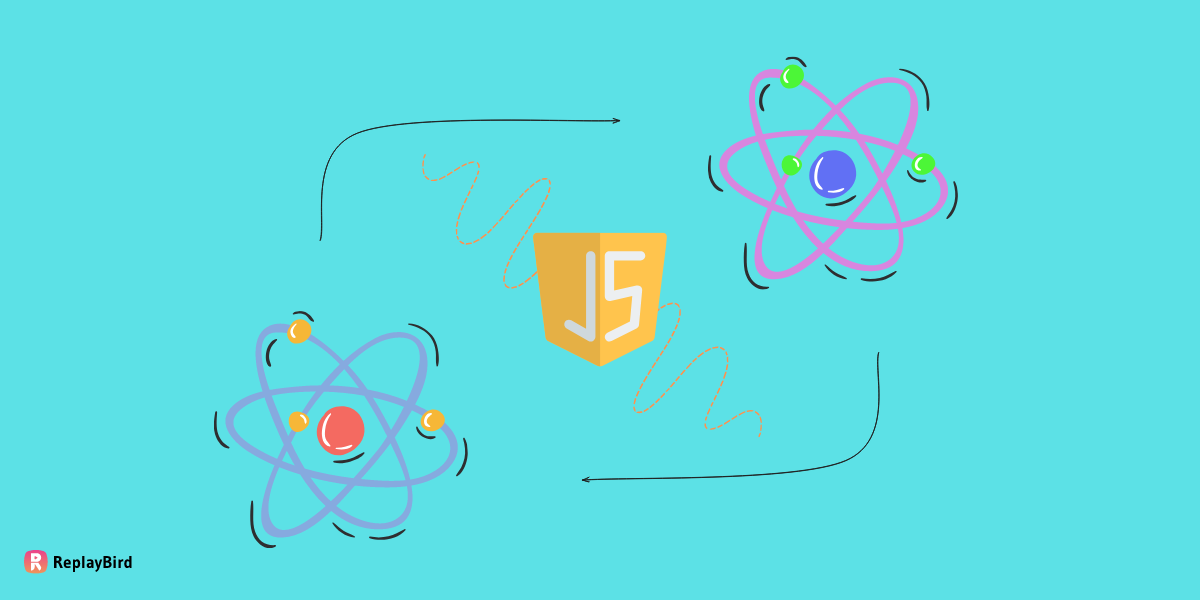
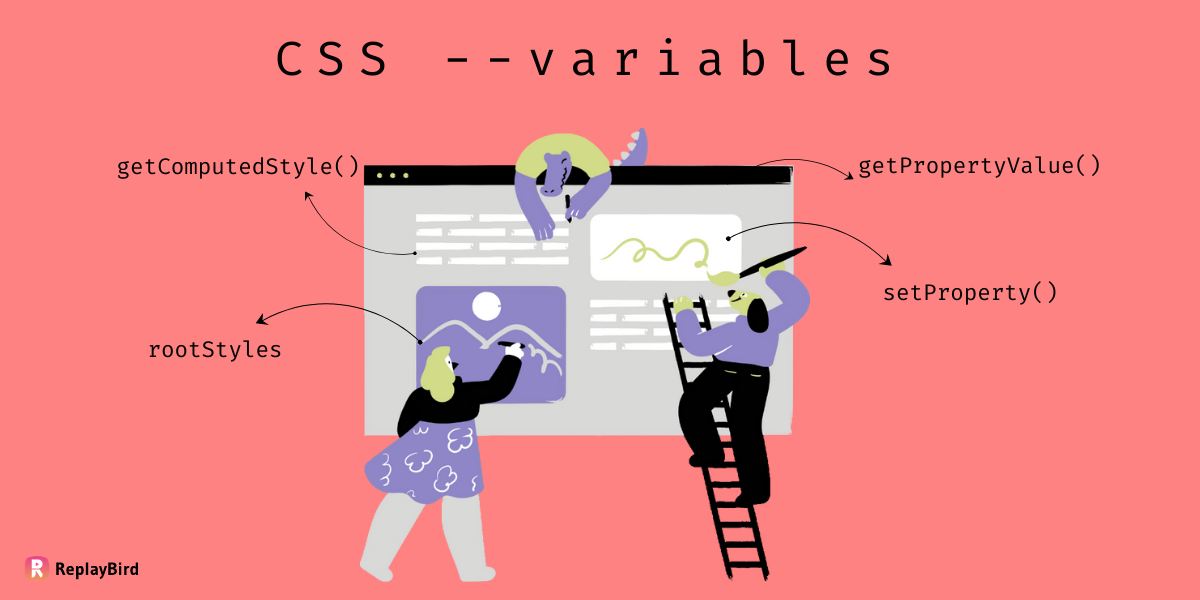