Query Parameters are essential in understanding API requests and acquiring specific data from the server as APIs (Application Programming Interfaces) act as connectors that facilitate smooth data exchange and seamless integration of functionalities between various software applications.
When developers interact with APIs, they often encounter the term "query parameters."
In this blog, we will delve into the details of query parameters, understand their syntax, explore common use cases, and learn how to implement them effectively in your web applications.
Let's get started!
Table of Contents
- What are Query Parameters?
- Why Use Query Parameters?
- Parsing Query Parameters
- Validating Query Parameters
- Advanced Query Parameter Techniques
- API Query Params Real-time Monitoring
What are Query Parameters?
Query parameters are key-value pairs appended to the end of an API endpoint's URL to modify the behavior of the request. They provide a straightforward and flexible way to pass additional information to the server, allowing clients to customize the API's response based on their requirements.
In a URL, query parameters are indicated by a question mark (?) followed by one or more key-value pairs, separated by ampersands (&). Here's an example of a URL with query parameters,
https://api.example.com/products?category=electronics&brand=apple&min_price=500
The query parameters in this case are as follows:
category=electronics
: By using this parameter, clients can filter the API response to include only products falling under the "electronics" category.brand=apple
: This parameter allows clients to filter the products further by brand. In this instance, it is set to "apple," indicating that the API response should include products exclusively from the brand "apple."min_price=500
: This parameter sets a minimum price threshold. The API will include only those products whose price is greater than or equal to 500 in the response. Thus, clients can narrow down the results based on the minimum price they are interested in.
Query parameters are connected using the ampersand &
character and follow the base URL. By utilizing query parameters, clients can easily understand the behavior of the API to suit their specific needs, making the API more versatile and user-friendly.
Why Use Query Parameters?
Query parameters add significant value to APIs due to their versatility. They enable clients to filter, sort, paginate, and customize data without requiring changes to the API's core structure. Decoupling the endpoint from customization details simplifies the API design and promotes code reusability.
For instance, imagine a music streaming API where clients can query for songs of a specific genre, filter by release date, and sort by popularity. Instead of creating separate endpoints for each combination, query parameters allow clients to send customized requests.
Let us see an example to understand it better. First, make sure you have Python and Flask installed. If not, you can install Flask.
# app.py
from flask import Flask, request, jsonify
app = Flask(__name__)
# Mock data for demonstration purposes
songs = [
{"id": 1, "title": "Song A", "genre": "Rock", "release_date": "2023-01-15", "popularity": 8},
{"id": 2, "title": "Song B", "genre": "Pop", "release_date": "2022-11-30", "popularity": 6},
{"id": 3, "title": "Song C", "genre": "Rock", "release_date": "2023-02-10", "popularity": 9},
# Add more songs here
]
@app.route('/songs', methods=['GET'])
def get_songs():
genre = request.args.get('genre')
min_popularity = int(request.args.get('min_popularity', 0))
sort_by_popularity = request.args.get('sort_by_popularity', '').lower() == 'true'
filtered_songs = songs
if genre:
filtered_songs = [song for song in filtered_songs if song['genre'].lower() == genre.lower()]
filtered_songs = [song for song in filtered_songs if song['popularity'] >= min_popularity]
if sort_by_popularity:
filtered_songs.sort(key=lambda song: song['popularity'], reverse=True)
return jsonify(filtered_songs)
if __name__ == '__main__':
app.run()
In this example, we have a list of songs stored in the songs
list. The /songs
endpoint accepts the following query parameters,
genre
: Allows clients to query for songs of a specific genre.min_popularity
: Sets the minimum popularity threshold for songs.sort_by_popularity
: If set to 'true', the API will sort the songs by the popularity in descending order.
Here are some examples of how clients can use the API,
To fetch all Rock songs
GET http://localhost:5000/songs?genre=Rock
To fetch Pop songs with minimum popularity of 7, sorted by popularity
GET http://localhost:5000/songs?genre=Pop&min_popularity=7&sort_by_popularity=true
To fetch all songs with minimum popularity of 5
GET http://localhost:5000/songs?min_popularity=5
Parsing Query Parameters
Parsing query parameters refers to the process of extracting and interpreting the key-value pairs present in the URL's query string. When clients make HTTP requests, they can include additional information in the form of query parameters to customize the request and influence the server's response.
Parsing query parameters in different programming languages and frameworks may vary, but the underlying concept remains the same. Let's look at how to parse query parameters in different programming languages.
1. Python
You can access query parameters using the request.args
object.
from flask import Flask, request, jsonify
app = Flask(__name__)
@app.route('/endpoint', methods=['GET'])
def example_endpoint():
# Accessing query parameters
parameter1 = request.args.get('parameter1')
parameter2 = request.args.get('parameter2')
# Your code here...
return jsonify(result)
if __name__ == '__main__':
app.run()
2. Node.js with Express
In Node.js with Express, you can access query parameters through the request.query
object.
const express = require('express');
const app = express();
app.get('/endpoint', (req, res) => {
// Accessing query parameters
const parameter1 = req.query.parameter1;
const parameter2 = req.query.parameter2;
// Your code here...
res.json(result);
});
app.listen(3000, () => {
console.log('Server is running on http://localhost:3000');
});
3. Ruby
Query parameters are available in the params
hash.
class ApiController < ApplicationController
def endpoint
# Accessing query parameters
parameter1 = params[:parameter1]
parameter2 = params[:parameter2]
# Your code here...
render json: result
end
end
In all three programming languages and frameworks, accessing query parameters is a fundamental step in processing client requests to an API. Query parameters are extracted from the URL and available to the server application in different formats.
Once the query parameters are accessed, developers can use them in their code logic to customize the API response or perform specific actions based on the provided parameters. This allows for more dynamic and versatile APIs, as clients can customize their requests without needing separate endpoints for every possible combination of parameters.
Validating Query Parameters
Validating query parameters means checking the information provided in the URL when someone requests your website or application. It's like ensuring the data you give is correct and safe. Let's use a simple example to understand this better.
To understand this we can take the same example as discussed earlier. Imagine you have a website displaying products, and users can search for products by providing details in the website's address bar (URL). They might search for https://yourwebsite.com/products? category=electronics&price=500
. Here, the query parameters are category=electronics
and price=500
.
- Checking if Parameters are Present:
You want to ensure that users always provide a "category" and a "price" when searching for products. If any of these parameters are missing, the website won't know what the user wants, and the search won't work correctly. - Validating Data Types:
You also want to ensure that the "price" parameter is a number, not just any random text. This way, you can avoid mistakes and prevent users from entering incorrect information. - Checking Parameter Values:
You might want to check that the "price" provided is not negative (less than 0) or extremely high (more than $10,000, for example). This prevents unreasonable requests and keeps the search results relevant. - Sanitizing Parameters:
Lastly, you may want to remove any unwanted characters from the parameters. This is like cleaning the input to avoid any potential issues with the website.
Advanced Query Parameter Techniques
Using advanced query parameter techniques can greatly improve the flexibility and functionality of your API. These techniques offer more sophisticated options for users to customize their requests and access specific data, making the API more versatile and user-friendly.
i) Combining Multiple Parameters: Allow clients to use multiple query parameters simultaneously to fine-tune their requests. Example - Online Bookstore API.
GET /books?genre=fiction&author=J.K.%20Rowling&published_year=2005
ii) Default and Optional Parameters: Setting default values for query parameters in an API helps prevent errors or unexpected behavior when clients forget to include certain parameters or leave them empty. By providing default values, the API can gracefully handle such situations, ensuring smooth functionality and delivering meaningful results for a better user experience. Example - Weather Forecast API.
GET /weather?city=London&units=metric
iii) Filtering and Sorting Data: Clients can filter results based on specific criteria and sort the data in ascending or descending order based on chosen attributes.
GET /movies?genre=action&rating=8.0&sort_by=release_date
iv) Pagination: Clients can request a specific page or limit the number of results per page, making it easier to handle large datasets.
GET /blogs?page=2&limit=10
v) Conditional Fetching: Clients can request only data that has been modified or created after a certain date. Example - Notification API.
GET /notifications?unread=true&modified_after=2023-01-01
API Query Params Real-time Monitoring
To address the potential issues arising from users changing query parameters and its impact on application stability and performance, we can implement a comprehensive and robust solution.
Firstly, on the server-side, strict validation for query parameters should be implemented, ensuring only allowed and valid parameters are accepted, and rejecting any invalid or unauthorized inputs to prevent malicious activities.
To continuously monitor APIs, Atatus offers API analytics platform that allows you to gain insights into how your APIs are being used. It provides monitoring and analytics capabilities for API traffic, including tracking query parameters.
With Atatus, you can drill down into specific API endpoints to see detailed information about the query parameters used with each endpoint. This analysis can help you identify popular query parameters, understand how they affect the API's performance, and detect potential issues.
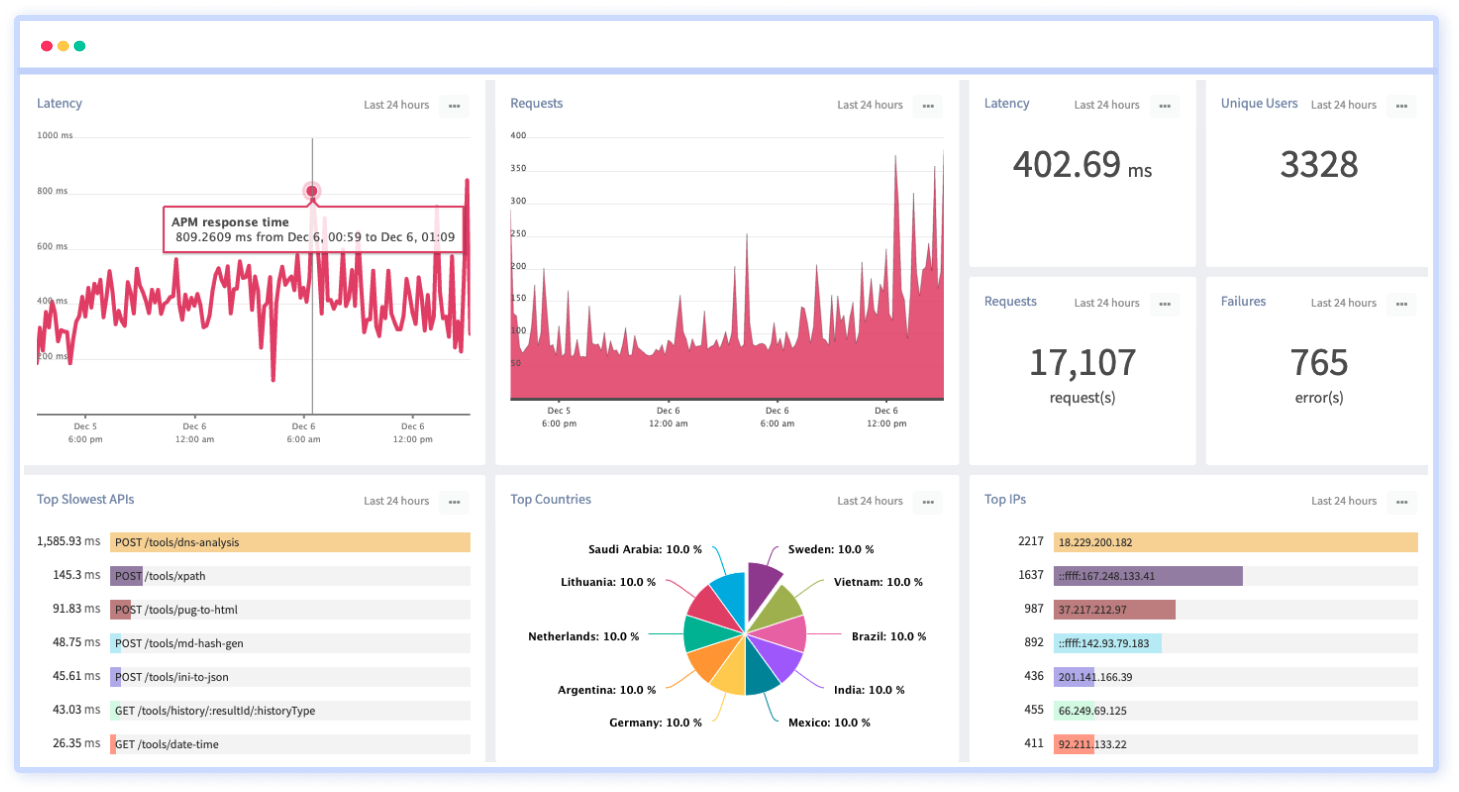
Monitor query parameters in Atatus:
- Analyze API usage: By tracking query parameters, you can understand how clients are using your API and which parameters are commonly used. This helps you identify popular features or functionalities that you should focus on or optimize.
- Identify trends and patterns: Atatus provides insights into trends and patterns in query parameter usage, helping you discover potential opportunities or issues in your API design.
- Detect anomalies and errors: Monitoring query parameters can also reveal if clients are sending incorrect or invalid values, leading to errors in your API responses. Identifying these issues helps you improve the user experience and prevent unnecessary errors.
- Optimize performance: By understanding how clients are using your API and which parameters are commonly utilized, you can optimize your API to handle the most common queries efficiently and make data-driven decisions to improve overall performance.
Conclusion:
Throughout this blog, we explored the significance of query parameters, their syntax, and their ability to enhance the flexibility and functionality of APIs. By leveraging query parameters effectively, developers can create more versatile and user-friendly APIs that cater to a wide range of client needs.
One essential aspect of working with query parameters is validating them to ensure their correctness and security. Developers can prevent injection attacks and handle errors by properly validating query parameters.
Query parameters are crucial in modern API development, allowing developers to build precise, efficient, and secure APIs that cater to client's needs. Utilizing query parameters and advanced techniques results in strong, user-focused APIs that form the foundation for successful applications and services.
ReplayBird - Watch & Track User Query Parameter Usage in your Web app
ReplayBird is a digital experience analytics platform where you can observe and analyze how users utilize query parameters when interacting with your APIs. By closely monitoring this aspect of user behavior, gain valuable insights into how they customize their requests, what specific data they are seeking, and how they interact with the API endpoints.
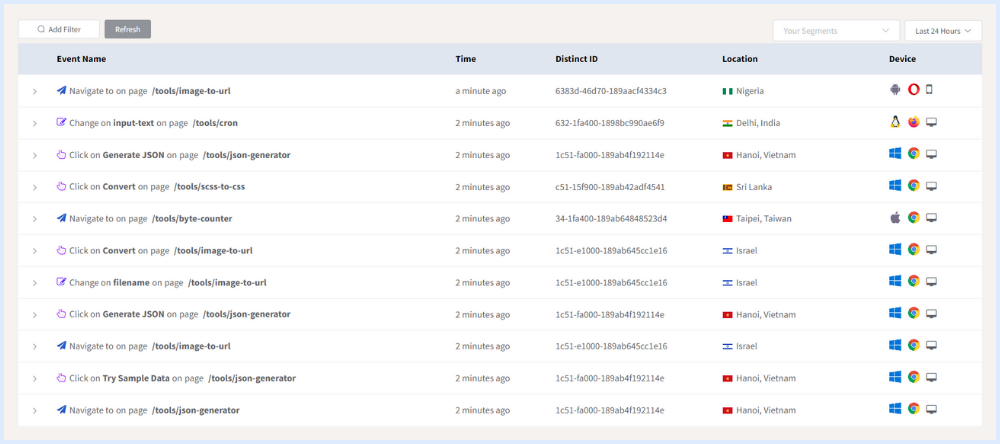
Define and monitor specific events or actions that users perform within your web application with event tracking. These events can include actions such as button clicks, form submissions, and any other custom journey, including user queries that involve query parameters.
By setting up event tracking for query-related interactions, you can gain insights into how users are using query parameters, what they are searching for, and how frequently specific queries are being performed.
Session replay is another valuable feature provided by analytics platforms like ReplayBird. It allows you to visually replay individual user sessions, showing their interactions and behavior during a specific visit to your web application. This feature enables you to observe how users interact with query parameters in real-time.
Try ReplayBird 14-days free trial
Keep Reading
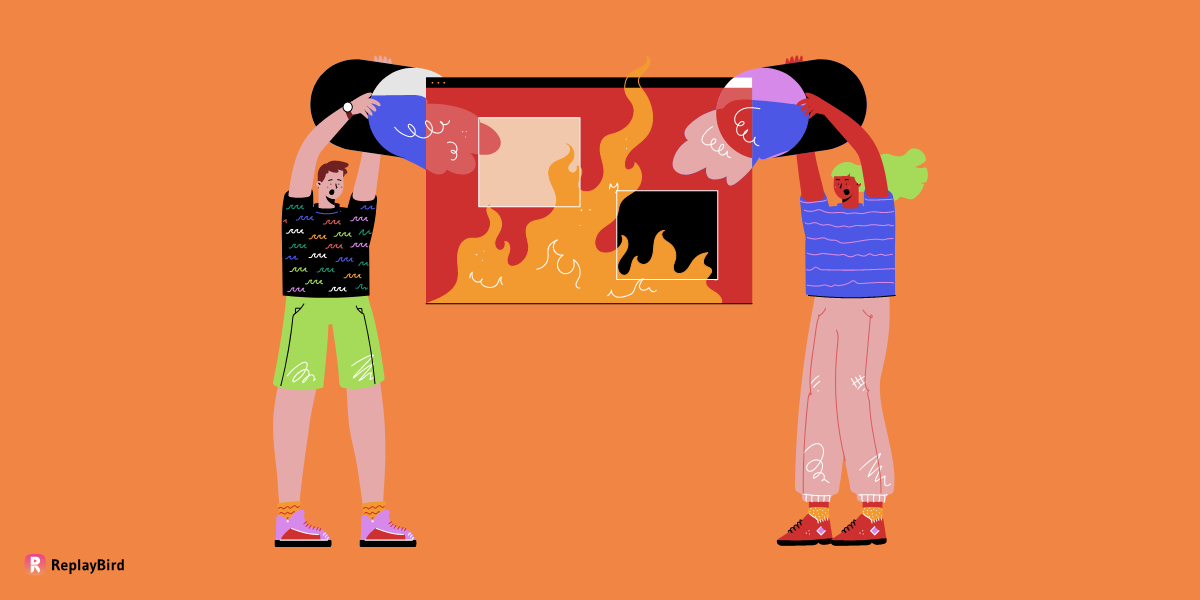