Next.js have released it's new update Next.js 13.4 on Thursday, May 4th 2023. Let us go deeper into all the new features updated in the Next.js 13.4 as Next.js is a popular and powerful framework for building modern web applications with React. It's packed with features and tools that make web development developer friendly whether you're a beginner or an experienced developer.
One of the standout features of Next.js is its built-in server-side rendering (SSR) capability. This means that your web pages can be rendered on the server and sent to the client as fully rendered HTML, improving performance and search engine optimization (SEO).
Next.js also supports static site generation (SSG), which allows you to pre-render pages at build time, resulting in lightning-fast loading times for your users. Plus, you can combine SSR and SSG for a dynamic and efficient website.
With Next.js, you get a robust routing system, automatic code splitting, and seamless integration with React. It also offers a great developer experience with features like hot module replacement (HMR), fast refresh, and extensive documentation.
But that's not all! Next.js is constantly evolving and introducing new features to make your development process smoother and more enjoyable. From server components to nested routes and layouts, Next.js keeps pushing the boundaries of what's possible in web development.
So, whether you're building a small personal project or a large-scale enterprise application, Next.js has got your back.
Let's dive in and see what Next.js 13.4 has in for us!
App Router
Next.js introduces the stable version of the App Router, which is built on React Server Components and Suspense. This new router architecture offers enhanced performance and flexibility in building web applications.
In the past, we used different APIs like getInitialProps
, getServerSideProps
, and getStaticProps
for data fetching. But now, with the App Router, we can use React Server Components to fetch data directly on the server side using familiar async/await syntax with Next.js 13.4.
Let's see an example of a Server Component in action:
// pages/posts/[id].js
import React from 'react';
export async function serverFetchData(id) {
const res = await fetch(`https://api.example.com/posts/${id}`);
const data = await res.json();
return data;
}
export default function Post({ post }) {
return (
<div>
<h1>{post.title}</h1>
<p>{post.content}</p>
</div>
);
}
export async function getServerData({ params }) {
const { id } = params;
const post = await serverFetchData(id);
return { props: { post } };
}
The getServerData
function is a Server Component that fetches data from the server and returns it as props to the component. This ensures that the data is fetched on the server side during server rendering, leading to better performance with Next.js 13.4.
1. React Server Components
The Server Components do the data-fetching right on the server, saving you from making extra requests from the client-side with Next.js 13.4. This is perfect for components that need data from external APIs or databases. It's like having a direct line to the server for your data needs.
From the Server Component Example earlier, it shows how easy it is to use Server Components to handle data mutations directly on the server with Next.js 13.4. With this approach, you can access the freshest and most up-to-date data at your fingertips without any struggle.
So, if you want to boost your app's performance and simplify data fetching, Server Components of Next.js 13.4 can make it easier.
2. Nested Routes & Layouts
With the App Router, you can create nested routes and layouts without any difficulty. This means you can build more flexible and complex interfaces for your web app, making it user-friendly.
All you need to do is organize your components in folders that match the route hierarchy you want.
For example, let's say we have a nested route for a blog:
posts/
├── article/
│ ├── index.js // Parent route - All article posts list
│ └── [slug].js // Child route - Individual article post
The index.js
and [slug].js
files will automatically form a nested route, where index.js
is the parent route displaying a list of all articles posts, and [slug].js
is the child route displaying an individual article post.
Additionally, "layouts" in with Next.js 13.4 are like templates that you can use to build consistent and instant user interfaces!
With layouts, you can create common components that you want to use on multiple pages. The best part is, you can even nest and combine layouts to make your UI look uniform across your whole app.
Here's a simple example to create consistent UIs with Next.js 13.4.
// layouts/MainLayout.js
import React from 'react';
const MainLayout = ({ children }) => (
<div>
<header>Header</header>
<main>{children}</main>
<footer>Footer</footer>
</div>
);
export default MainLayout;
Now, you can use this layout in any page to wrap its content:
// pages/about.js
import React from 'react';
import MainLayout from '../layouts/MainLayout';
const About = () => (
<MainLayout>
<h1>About us</h1>
<p>Some content about the company</p>
</MainLayout>
);
export default About;
This way, the MainLayout
will be applied to the About
page and any other page you wrap with this layout.
3. Simplified Data Fetching
The App Router in with Next.js 13.4 simplifies data fetching by providing a unified and easy-to-use approach to fetch data on the server side. As shown in the earlier example, you can define Server Components that fetch data during server rendering.
This makes it straightforward to fetch data for each specific page or component without having to use different APIs like getInitialProps
, getServerSideProps
, or getStaticProps
, which were used in older versions of Next.js.
4. Streaming & Suspense:
Next.js 13.4 integrates React Suspense, letting progressive streaming of content from the server. This means that parts of the page can be sent to the client as they are ready, improving perceived loading performance for a smoother user experience.
Suspense allows you to define fallback content that is shown while data is loading. Once the data is ready, the actual content is shown, resulting in a more fluid and responsive user interface with Next.js 13.4.
In the Server Components example provided earlier, the data fetched from the server can be used to show fallback content until the data is available:
// pages/posts/[id].js
import React from 'react';
import { serverFetchData } from './api';
export default function Post({ post }) {
if (!post) {
return <p>Loading...</p>;
}
return (
<div>
<h1>{post.title}</h1>
<p>{post.content}</p>
</div>
);
}
export async function getServerData({ params }) {
const { id } = params;
const post = await serverFetchData(id);
return { props: { post } };
}
In this example, if the post
data is not available yet (while fetching), the component displays the fallback content "Loading...". Once the data is fetched, the actual content is shown.
5. Built-in SEO Support
Next.js 13.4 provides built-in SEO support, making it easier to optimize your web application for search engines. This includes features like automatic generation of <head>
tags, meta information, and canonical URLs.
For example, you can customize the <head>
section of each page to include specific metadata, such as page titles and descriptions:
// pages/about.js
import React from 'react';
import Head from 'next/head';
const About = () => (
<div>
<Head>
<title>About Us - My Company</title>
<meta name="description" content="Learn about our company and our mission." />
</Head>
<h1>About us</h1>
<p>Some content about the company</p>
</div>
);
export default About;
This make sure that search engines can better understand and index your web pages, leading to improved discoverability and visibility on search engine results pages (SERPs) with Next.js 13.4.
Turbopack (Beta)
The new bundler Turbopack, that's currently being tested and fine-tuned in Next.js 13.4, it's all about speeding up development!
Turbopack really have enhanced on Next.js applications locally. With a simple next dev --turbo
command, the iterations are faster! And that's not all – it's also faster in production builds with next build --turbo
.
{
"scripts": {
"dev": "next dev --turbo",
"build": "next build",
"start": "next start",
"lint": "next lint"
}
}
Since its alpha release in Next.js 13, the team has also tested it out on vercel.com and with big Next.js 13.4 websites to make sure it's stability.
Now, it's reached the beta phase, which means it's getting closer to being stable and ready for prime time. While it may not have every single feature of webpack and Next.js 13.4 just yet, it already covers the majority of use cases. The team is dedicated to pressing out any remaining bugs and making it super reliable.
Turbopack not only speeds up local development but also boosts production builds. That means faster loading times for our users and an all-around better experience.
There is more in the next version of with Next.js 13.4, where we'll have access to the next build --turbo
command and get to experience those instant builds!
Server Actions
Imagine you're building a web app with React using Next.js 13.4, and you need to handle data changes like submitting forms or updating information. Traditionally, this would involve sending requests to the server through an API, which can be a bit of a complicated.
Well, Server Actions allows you to perform data mutations directly on the server, without dealing with an extra API layer. This means less complexity and faster performance.
With Server Actions, you can call server-side functions right from your React components. So when a user interacts with your app, the data changes happen directly on the server. That way, you get the latest data without any complicated client-side state management.
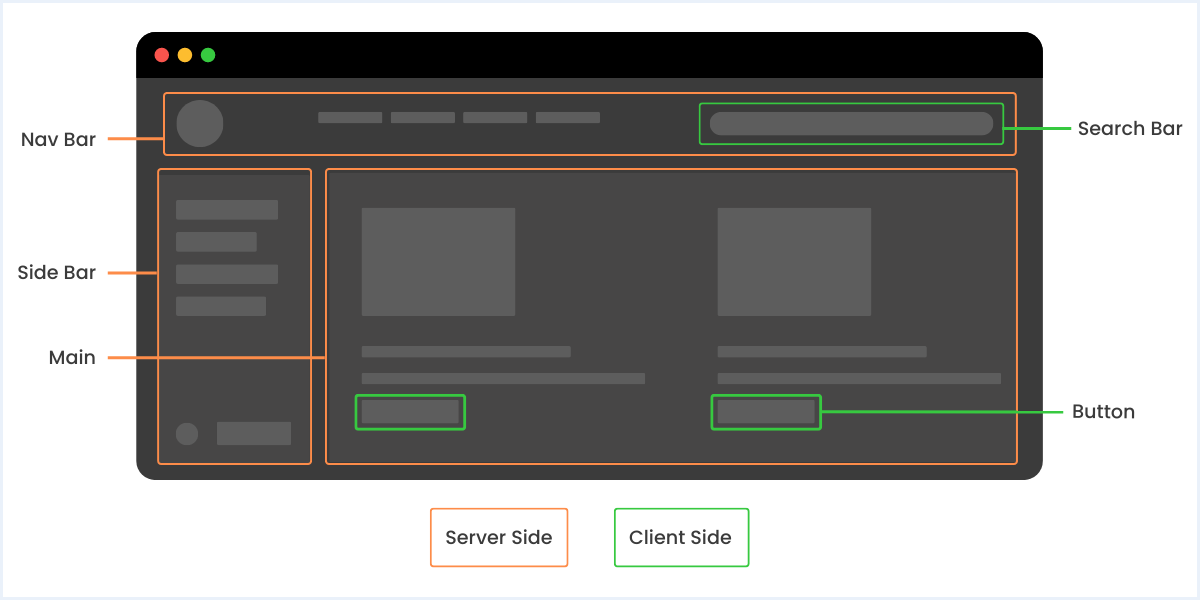
Here are some key highlights of Server Actions:
- Server-First Data Mutations: Your data changes get processed on the server first, ensuring you always have the freshest data.
- Simplified Forms and Data Management: You can easily handle form submissions and data operations directly within your React components. It's intuitive and efficient.
- Progressive Enhancement: Server Actions work great with JavaScript enabled, but even if it's disabled, your app still functions smoothly.
- Deep Integration with Data Lifecycle: Server Actions play nicely with other data-related features in Next.js, like caching and data regeneration, making your app even more efficient.
- Composability and Reusability: You can create and reuse Server Actions across different parts of your app, keeping your code organized and easy to maintain.
It's essential to note that Server Actions are introduced as an experimental feature in Next.js 13.4 and might undergo changes and improvements based on user feedback and further development. As such, developers should be cautious when using Server Actions in production applications and consider testing them thoroughly with Next.js 13.4.
To enable Server Actions in with Next.js 13.4, developers can configure their with Next.js 13.4 project with the appropriate settings in the next.config.js
file, as shown in the provided code snippet.
Conclusion:
Next.js 13.4 has brought some exciting improvements and features making web development developer friendly.
From The App Router, Nested Routes & Layouts, Simplified Data Fetching, Streaming & Suspense, Built-in SEO Support to Turbopack (Beta), and Server Actions all are great initialtion to improve the performance, flexibility, and productivity.
As more into Next.js 13.4, the introduction of Server Components, Suspense, and other improvements to make your development journey smoother and more enjoyable.
Understand Exactly How Your Users Interact with Your Next.js app
ReplayBird, a digital user experience analytics platform designed specifically for Next.js developers with advanced insights to optimize your Next.js applications like a pro!
Unleash the power of behavioral insights with ReplayBird's intuitive heatmaps, session replays, and clickstream analysis allows you to visualize user behavior, identify popular elements, and detect pain points that might hinder user satisfaction.
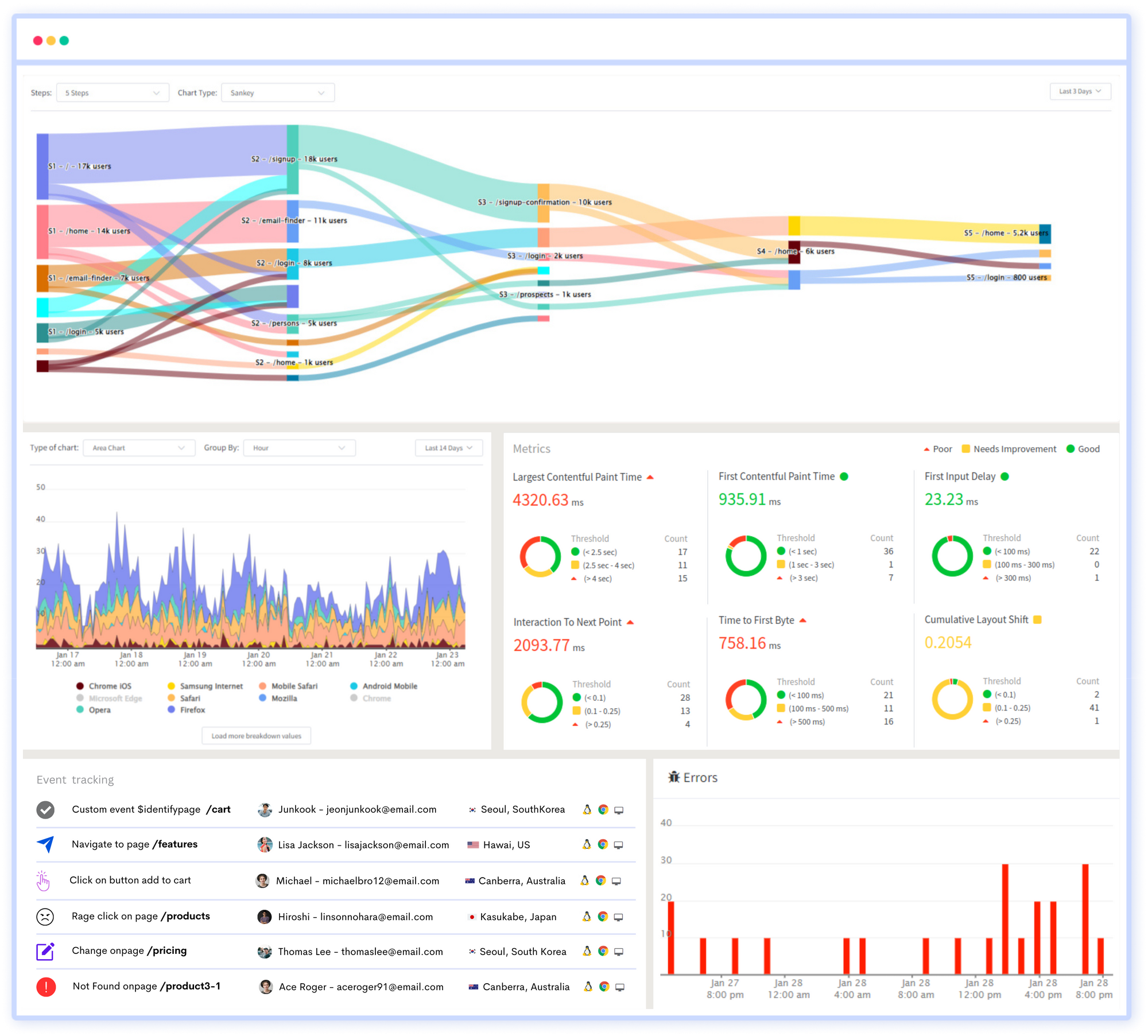
Customer journey analysis and conversion funnels of ReplayBird to analyze deeper into user journeys, identify where drop-offs occur, and uncover conversion blockers.
Troubleshooting is now simpler with ReplayBird's robust debugging features. Detect and diagnose UX issues quickly, ensuring a seamless user journey from start to finish.
With ReplayBird, you have the ultimate toolkit to elevate your Next.js projects to the next level. The platform empowers you to create high-performing, user-centric applications that leave a lasting impression.