Before getting into REST API Error Handling, let us first learn a little about REST API. REST stands for representational state transfer.
REST API was introduced by Roy Fielding, a computer scientist. API stands for "Application Programming Interface," and REST API is a set of protocols for developing application software.
Here are the topics that we will cover in this blog:
- What is REST API error handling?
- Why is REST API error handling important?
- Call/request status
- Categories of API error codes
- Best practices for handling errors
What is REST API error handling?
In simple terms, APIs help you communicate with your system. When you want your system to perform a particular action, API assists you in communicating what you want to the system, which in turn fulfils your request.
However, REST is not a set of protocols or standards; rather, it is a set of architectural constraints. APIs implement REST in a variety of ways.
For instance, when a client requests something via a RESTful API, it delivers the state of the resource to the client.
This information is delivered via formats such as HTTP, HTML, plain text, PHP, etc.
Errors are an unavoidable factor at these interfaces. The right and meaningful delivery of error messages prove useful to clients for responding to such errors.
Efficient handling of errors makes debugging easier and less complicated.
Moreover, consistency across applications is ensured through efficient error handling. In this article, let us see in detail REST API Error Handling.
Call/request status of API error codes
To connect to clients/users, RESTful APIs based on HTTP protocol are used by the application developer. The response to clients must be made for their request in order to proceed to the next step of the process. The response must take place in both of the following cases:
#1 The success of API calls
In this case, the clients at the endpoint can move to the next step of their action. It is important to note that the success call must also be communicated in order to let the clients know that they can move forward. Examples are:
- Code 200: It indicates that the request was successful.
- Code 201: It refers to the "created" API error code wherein the newly added information is successfully posted.
- Code 202: It refers to the "accepted" API error code, wherein it indicates that the request was received successfully and the processing is being done.
- Code 204: It refers to no content API error code. It indicates that the request was successful, but there will be no information returned in the response.
#2 Failure of API call
This call indicates an error with regard to the request made. We saw earlier about the API error codes through which errors are communicated to the user. In this case, the user is required to modify their actions in order to make their request successful.
Or else, the directions or information from the error message must be followed to resolve the call.
Why is REST API error handling important?
When software developers build application software, they add API error codes to deal with errors as well. Errors are inevitable when dealing with software development. To make it an uncomplicated process, efficient error handling is important.
Furthermore, error handling makes API error code resilient to errors and acts as a recovery strategy. It does not eliminate errors itself; rather, it helps make the process of debugging easier.
When things are off track, error handling makes the API error code work smarter, thereby helping the stakeholders involved in the process.
Error handling is an important aspect of building a REST API because it allows the API to gracefully handle and respond to errors that may occur during the processing of requests.
Without proper error handling, an API may return unexpected results or behave in unpredictable ways, which can lead to a poor user experience and potentially cause issues for clients that are consuming the API.
There are several benefits to implementing effective error handling in a REST API:
-
Improved reliability: Proper error handling can help to ensure that an API is reliable and performs consistently, even when unexpected errors occur.
-
Enhanced user experience: By returning clear and informative error messages to clients, an API can help users to understand and resolve issues that may arise while using the API.
-
Easier debugging: By providing detailed error messages, an API can make it easier for developers to debug issues that may arise while using the API.
-
Increased security: By handling errors in a consistent and predictable manner, an API can help to prevent sensitive information from being disclosed to clients or other unintended parties.
-
Improved performance: Proper error handling can help to prevent the API from wasting resources on processing invalid or malicious requests.
-
Enhanced scalability: By returning appropriate error messages to clients, an API can help to prevent clients from sending unnecessary or invalid requests, which can help to improve the scalability of the API.
-
Greater flexibility: By providing clear and descriptive error messages, an API can help clients to understand the requirements for using the API and make it easier for them to integrate with the API.
Categories of API error codes
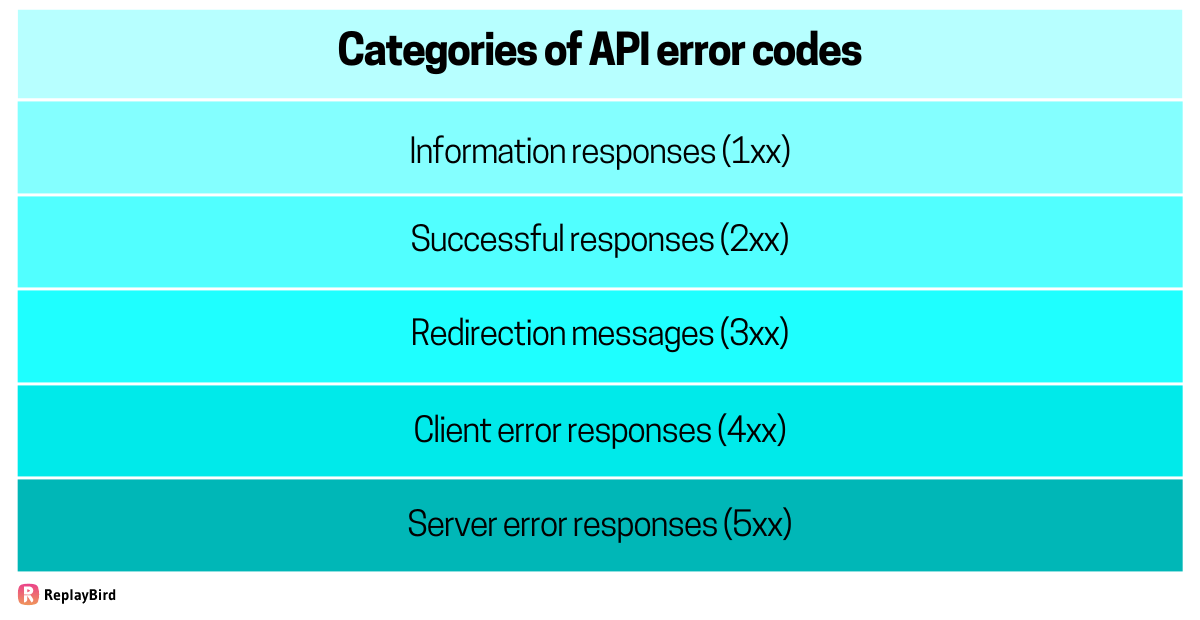
In order to deliver the results of the client's request, HTTP offers over 40 standard codes that can be used by developers for the same purpose. Let us look at the categories of API error code it defines:
#1 Information responses (1xx)
These API error codes communicate information to the clients based on their requests. Let us look at a few API error codes to understand this better.
- Code 100: It is a "continue" code. This indicates that the client should continue with their request or ignore the response if the request is complete.
- Code 101: It is a switching protocol. When an upgrade request header is received from the client, this API error code is sent, indicating the protocol that the server is switching to.
- Code 102: It refers to processing requests. This indicates that the server is processing the request but that no response is yet available.
#2 Successful responses (2xx)
These API error codes indicate that the request from the client was accepted successfully. We have discussed earlier the API error codes for the success of API calls.
#3 Redirection messages (3xx)
These API error codes indicate that the client is required to take additional actions in order to complete their request successfully. Let us look at a few API error codes of redirection:
- Code 300: It refers to multiple choices. This indicates that the request from the client has more than one response. In this case, users are recommended multiple HTML links to the possibilities to choose from.
- Code 301: It refers to "moved permanently." This indicates that the requested page has been changed permanently, and a new URL has been given in response to the request.
#4 Client error responses (4xx)
These API error codes indicate that the error is on the client side. We have seen earlier some of the basic client API error codes. Let us look at a few more now:
-
Code 405: It refers to "method not allowed." This indicates that the requested method is not supported by the target resource but is known to the server.
-
Code 400 - It refers to a bad request and indicates that the server cannot process the request due to an error from the client side. It could be a wrong syntax, an invalid request, etc.
-
Code 401 - It refers to unauthorized access and indicates that the request from the client lacks valid authentication credentials to fulfil the requested process.
-
Code 403 - It indicates an access permission issue. It denotes that access to the requested page is not allowed or banned.
-
Code 404 - It is a not found response and indicates that the server cannot find the requested source. It could be thought of as a broken link, and the requested resource is missing.
-
Code 406: It refers to "not acceptable." This indicates that the server does not find any content that confirms the criteria requested by the user.
-
Code 407: It refers to "proxy authentication required." This indicates that the authentication credential is required from the proxy and is similar to the code 401 that we discussed earlier.
-
Code 408: It refers to "request timeout." This API error code is sent even without any previous request sent by the client on idle connections. This states that the server would shut down in an unused connection.
-
Code 409: It refers to "conflict." This indicates that the request sent by the client is in conflict with the current state of the server.
-
Code 410 - It refers to permanently not found wherein the requested page is permanently deleted and can never be accessed. But for the case of API error status code 404, the page might be updated and made accessible again and the not found response is temporary in nature unlike the API error status code 410.
#5 Server error responses (5xx)
These codes indicate that the error is from the server side, which takes responsibility for them. We have already seen some of the basic server API error codes. Let us look at a few more now:
- Code 500 - It refers to server error and indicates that the server is temporarily inaccessible. It indicates that there is a problem in the server and resolving the error from the server end will enable access to the requested page.
- Code 501: It refers to "not implemented." This indicates that the requested method is not supported by the server. GET and HEAD are the only methods that the servers are required to support.
- Code 502: It refers to a "bad gateway." This indicates that while the server was working as a gateway to get a response, it received an invalid response in return.
- Code 503 - This API error code stands for "server unavailable." It indicates that the server might have overload or is undergoing maintenance due to which it is currently unavailable. Moreover, cyber attacks also cause this type of situation.
- Code 504: It refers to "gateway timeout." This indicates that while the server is working as a gateway, it did not receive a response in time.
- Code 505: It refers to "HTTP version not supported." This indicates that the HTTP version used by the client in the request is not supported by the server.
- Code 507: It refers to "insufficient storage." This indicates that the method could not be processed since the server was unable to store the representation required to successfully complete the request.
- Code 508: It refers to "loop detected." This indicates that the server found an infinite loop while trying to process the request.
- Code 511: It refers to "network authentication required." This indicates that authentication is required from the client side in order to gain network access.
Best practices for handling errors
Here are some best practices for handling errors and returning appropriate HTTP status codes in your web application:
-
Use the proper HTTP status code: Use the relevant HTTP status code for the sort of issue you're dealing with. Use a 404 status code for sites that cannot be found and a 400 status code for incorrect or malformed requests.
-
Return an informative error message: In the response body, include a comprehensive error message to assist developers in understanding and debugging the problem.
-
Use the effective content type: Set the response's Material-Type header to the proper value for the type of content you're returning. Use application/json for JSON replies, and text/html for HTML responses, for example.
-
Avoid revealing important information: Avoid including sensitive information in error messages, as this may expose vulnerabilities in your application.
-
Log errors: Always log errors so that you can monitor and diagnose problems in your application.
-
Test your error handling code: confirm that it is functioning properly and provide the necessary HTTP status codes and error messages.
-
Use HTTP headers to offer more detail: In addition to the response body, HTTP headers may be used to provide further context about the problem. You may, for example, use the Repeat-After header to tell the client when it should retry the request.
-
Consider utilising HTTP error pages: HTTP error pages may be used to give a user-friendly interface for problems in your application. Depending on the HTTP status code of the issue, these pages can show a personalised message or a predetermined one.
-
Utilise separate error pages for different contexts: (e.g. development, staging, production). For example, in the development environment, you could want to provide a more descriptive error message to aid debugging, but in production, you might want to show a more general error message to avoid revealing sensitive information.
-
Monitor errors: Keep track of any errors that occur in your programme so that you may detect and resolve problems as they arise. This can help improve the reliability and stability of your application.
-
Manage exceptions with try/catch blocks: In many programming languages, you may use try/catch blocks to handle exceptions raised by your code. This can help you handle unexpected failures gracefully and keep your application from crashing.
-
To identify the sort of problem, use HTTP status codes: HTTP status codes are three-digit numbers that indicate what sort of issue occurred. A 4xx status code, for example, indicates a client fault, whereas a 5xx status code indicates a server issue. Use the relevant status code for the sort of issue you're dealing with.
-
Set the Content-Type header as follows: The Content-Kind header informs the client about the type of content contained in the response body. Make sure to set this header to the appropriate value for the type of content you are returning. For example, use application/json for JSON responses and text/html for HTML responses.
-
To determine when to retry the request, use the Retry-After header: If an error occurs as a result of the server being overloaded or the client making too many requests, you may use the Repeat-After header to tell the client when it should retry the request.
-
Log errors for debugging and tracking: Make a habit of logging failures in your application so that you can monitor and diagnose any problems that develop. This can help you detect and resolve issues more quickly.
How to monitor REST APIs?
To monitor REST APIs using Atatus API Monitoring, you can follow these steps:
Set up Atatus: If you haven't already used a API monitoring tool, Create an Atatus account by signing up to atatus and get the free trial.
Install APM Atatus: Install the Atatus agent. The Atatus agent is a small library that you need to include in your application in order to start sending performance data to the Atatus platform. You can find installation instructions for the agent on the Atatus website. The instruction will be different for different languages and framework you are using.
Configure the check as follows: Set the check interval and timeout (how frequently Atatus will check the API) (how long Atatus will wait for a response from the API before considering it a failure). You may also define the expected HTTP status code and, if necessary, add custom headers to the request.
Create alerts: You may create alerts to get notifications when the API check fails or specific thresholds are exceeded. For example, you may configure an alert to be triggered if the API response time exceeds a given threshold.
Keep track on the results: The results of the API checks will be shown in the Atatus web interface, allowing you to track the status and performance of your APIs over time. You may use this information to discover and troubleshoot API problems.
Benefits of Monitoring REST APIs using Atatus API Monitoring
There are several benefits to using Atatus API Monitoring to monitor your REST APIs:
Real-time monitoring: Atatus API Monitoring monitors your APIs in real-time, allowing you to discover and resolve issues as they emerge.
Customizable tests: You may tailor the checks to your API's unique requirements, such as altering the check interval, timeout, and anticipated HTTP status code.
Notifying: To keep updated about the status of your APIs, you may set up alerts to tell you when the API check fails or when specified thresholds are surpassed.
Performance metrics: Atatus API Monitoring delivers performance indicators such as response time and uptime to help you detect and manage API performance issues.
Integration: Atatus API Monitoring connects with other Atatus capabilities such as logs and tracing, giving you a more comprehensive picture of your application's performance and helping you to spot issues faster.
Overall, utilising Atatus API Monitoring to monitor your REST APIs may assist you in ensuring the availability and performance of your APIs, as well as rapidly identifying and resolving issues as they happen.
Conclusion
Handling errors while developing REST APIs is an inherent part of software development. Careful consideration of the errors comes in handy while working with the software.
Useful mechanisms in the API error code for error handling and provision of information to the client for the same prevent malfunctioning or blockage of application due to error.
Overall, efficient handling of errors is something that the developer must not miss in order to make things easy and efficient for both the developer and the client.
ReplayBird - Driving Revenue and Growth through Actionable Product Insights
ReplayBird is a digital experience analytics platform that offers a comprehensive real-time insights which goes beyond the limitations of traditional web analytics with features such as product analytics, session replay, error analysis, funnel, and path analysis.
With Replaybird, you can capture a complete picture of user behavior, understand their pain points, and improve the overall end-user experience. Session replay feature allows you to watch user sessions in real-time, so you can understand their actions, identify issues and quickly take corrective actions. Error analysis feature helps you identify and resolve javascript errors as they occur, minimizing the negative impact on user experience.
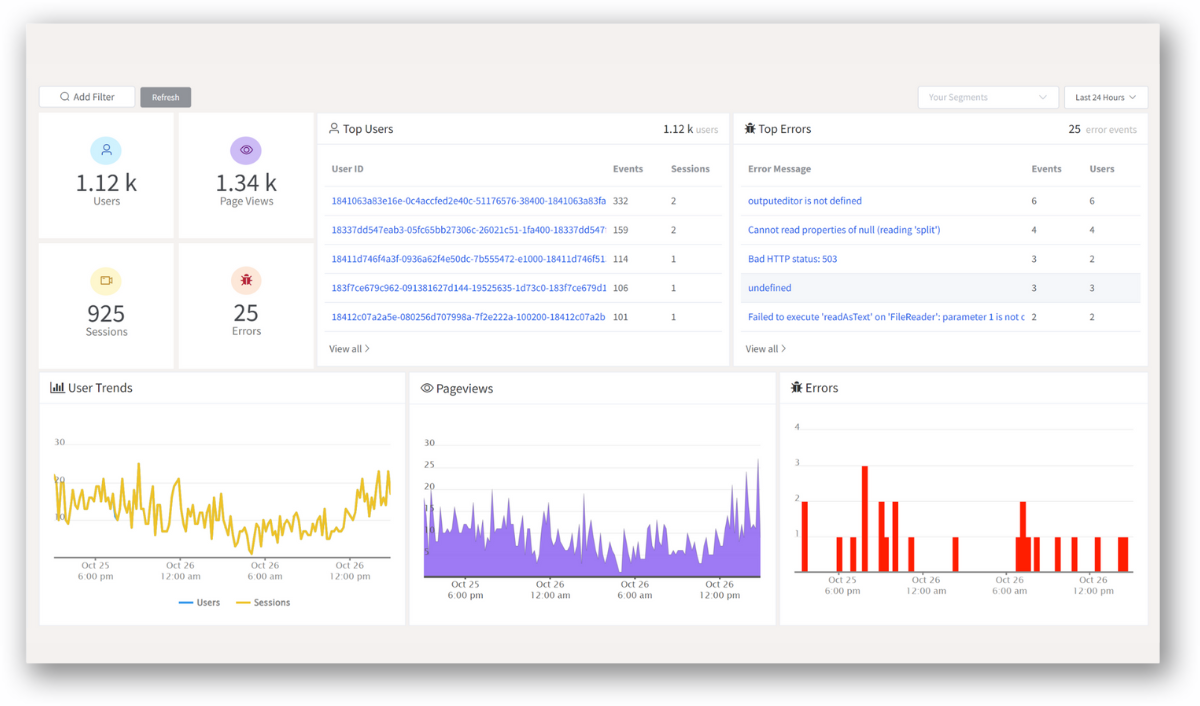
With product analytics feature, you can get deeper insights into how users are interacting with your product and identify opportunities to improve. Drive understanding, action, and trust, leading to improved customer experiences and driving business revenue growth.
Try ReplayBird 14-days free trial
Further Readings:
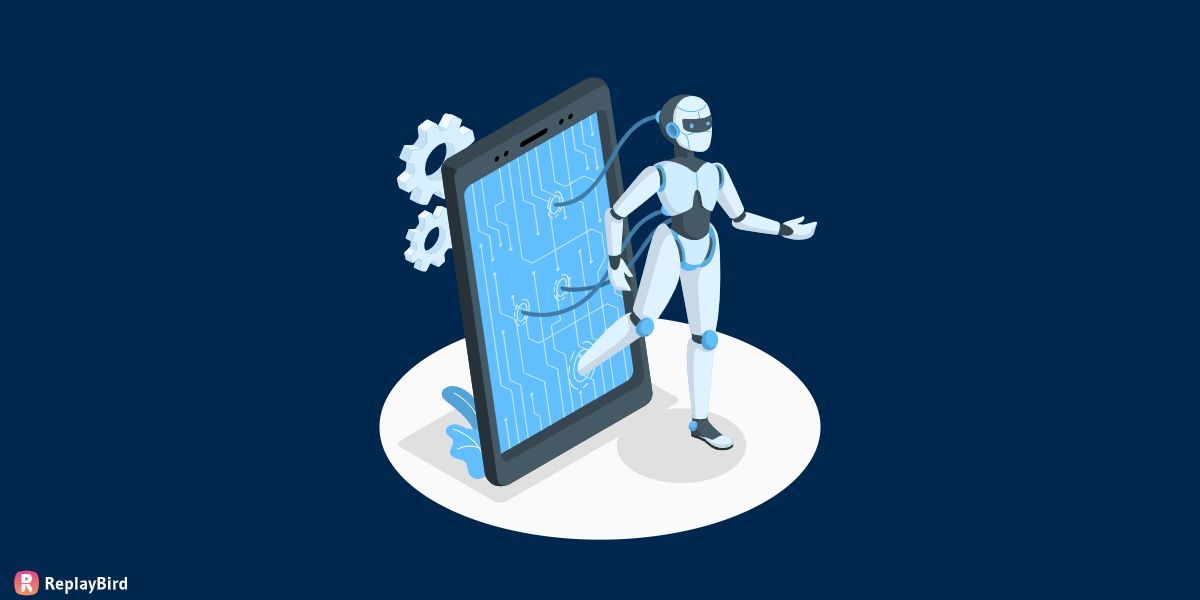
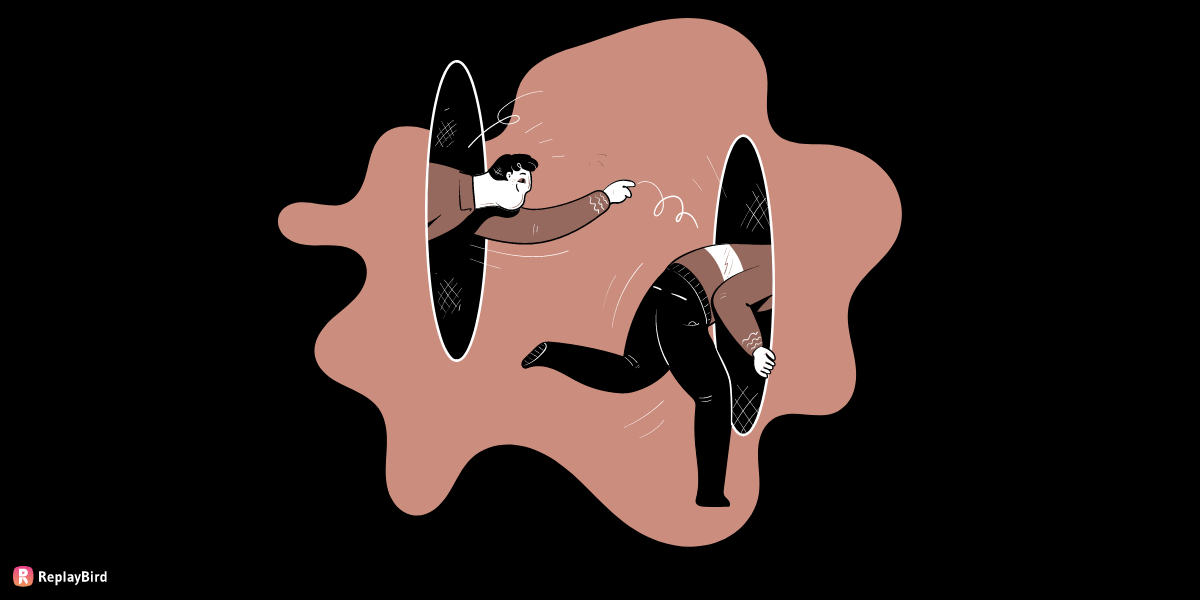
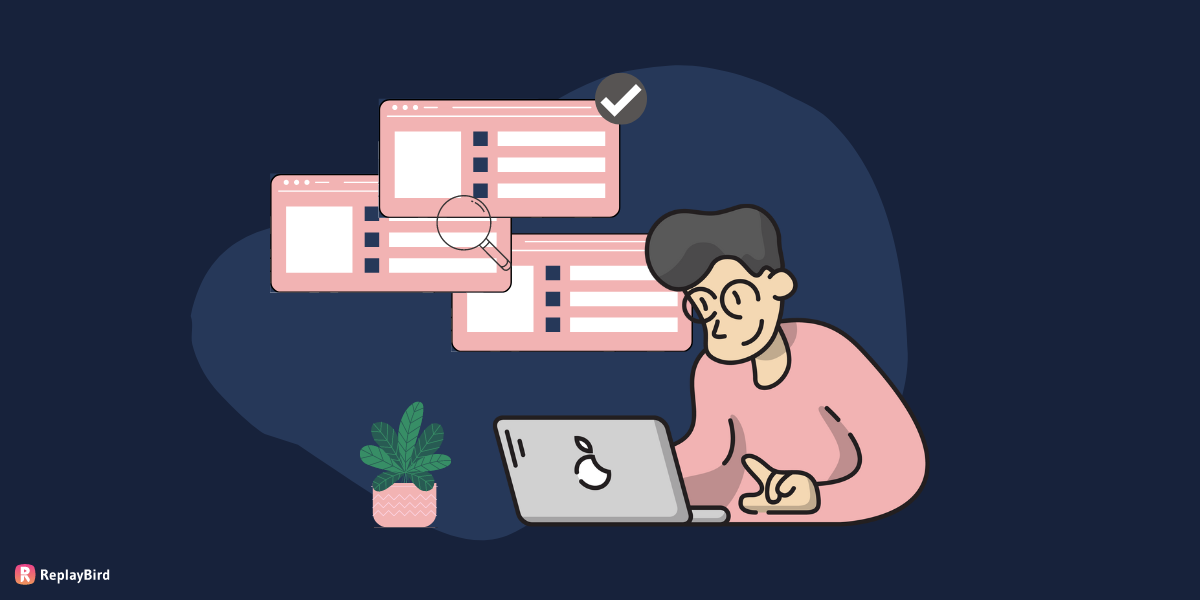