All the HTML elements on your webpage have to be loaded and rendered in order to be visible to your users. They can be prioritization of images, text, or elements with bulk CSS and JavaScript. One of the major keys to making your webpage user-friendly is to make it load faster.
But now you don't know what to do with your webpage because you don't know how to handle its loading. The fetchpriority
attribute helps you handle the loading of your webpage.
Table of Content
- What are Fetch Priority?
- Fetch Priority Attribute
- How to Use Fetchpriority?
- When to Use Fetch Priority?
- Browser Compatibility of Fetchpriority
As per Google's Largest Contentful Paint (LCP) metric, your website has a certain time frame to load. The faster your webpage content loads, the better the loading experience it gives your user, which is the prior goal of measuring the LCP metric of your webpage.
But Google is smarter, so it does not mind if the last part of your webpage is loading slowly compared to the top part of your webpage, as users face the top part of your webpage first. Now let's say you enter a webpage and you see the header keeps loading, and you have to wait until it gets loaded. You get frustrated and exit the webpage, which leads to a bad user experience, resulting in a lower conversion rate.
Now, let's give it a try again. You enter a webpage, you see the header has loaded, all the texts are clearly in the format, and at the perfect layout, you keep scrolling and scrolling. You see a page taking some time to load, say 0.8 seconds.
Okay, you waited and kept scrolling; you did not mind. Though some still mind such minor issues, what all you had in mind was that the page loaded faster just because the header prioritization image loaded faster, which did not let you have frustration or keep you waiting.
That is exactly what Google wants from you. You need to prioritize what the major content or HTML user focus is and what you want to highlight.
What are Fetch Priority?
fetchpriority
Also known as crawl priority or crawl budget, is an attribute that website developers can use to tell web browsers which parts of a webpage are more important and should be loaded first. These parts could be things like images, fonts, styles (CSS), programming code (scripts), or embedded content (iframes).
When a browser starts to build a webpage, it first collects the HTML document because that's the starting point of the webpage. But after that, the browser needs to decide what to load next.
However, website creators might know their projects better than the browser does. They might have specific parts of their webpage that are more important than what the browser assumes.
By using thisfetchpriority
, website developers can give the browser hints about what's most crucial to load next. Even though browsers try to make good guesses about what to load after the HTML, website owners can provide extra guidance so that the most important parts load quickly and in the right order for their specific project.
For example, if a webpage relies heavily on data fetched from a REST API, SOAP API, or partner API web developers can set fetchpriority to ensure that these API calls are prioritized over other resources, optimizing the loading sequence and enhancing user experience.
How Web Browsers Load a Webpage?
Browser follows a step-by-step process in an organized sequence as it collects all the necessary information from the HTML, styles, scripts, and priority images, and then presents the complete webpage to you.
- HTML Document
- Referenced Resources - CSS, JS, Images
- Critical Render Path
- Render Tree - using DOM and CSSOM
- Render-Blocking Resources - CSS, font files, and scripts
- Rendering the Page
Fetch Priority Attribute
The fetchpriority
attribute can be used within the <link>
, <img>
, and <script>
Tags.
Fetch Priority Attribute has 3 Priority Levels:
- High: Indicates that the resource is of high importance and should be prioritized by the browser, given that the browser's default behavior or rules allow it.
fetchpriority="high"
- Low: Suggests that the resource is of low importance and can be deprioritized by the browser if its heuristics or rules permit it to lower priority.
fetchpriority="low"
- Auto: This is the default value. It signifies that there's no specific preference set by the developer to decide the fetchpriority based on its own rules and heuristics.
How to Use Fetchpriority?
Let's imagine you have a list of resources (such as images) that you want to load with different priorities. You can use a similar behavior to fetchpriority
by controlling the order of fetching based on priority
using setTimeout
:
// Simulated list of resources with different priorities
const resources = [
{ url: 'img/resource1.jpg', priority: 'high' },
{ url: 'img/resource2.jpg', priority: 'low' },
{ url: 'img/resource3.jpg', priority: 'high' },
// Add more resources with their respective priorities
];
// Function to fetch a resource with a specified delay
function fetchResource(resource) {
return new Promise((resolve, reject) => {
// Simulating a delay for fetching the resource
setTimeout(() => {
console.log(`Fetching ${resource.url} with priority: ${resource.priority}`);
// Simulating success by resolving the promise after the delay
resolve(`${resource.url} fetched`);
}, resource.priority === 'high' ? 1000 : 3000); // Adjust delay based on priority
});
}
// Fetch resources based on their priorities
function fetchResourcesWithPriority() {
const fetchPromises = resources.map(resource => fetchResource(resource));
return Promise.all(fetchPromises);
}
// Fetch resources with priority and handle the results
fetchResourcesWithPriority()
.then(results => {
console.log('All resources fetched:', results);
// Handle the fetched resources
})
.catch(error => {
console.error('Error fetching resources:', error);
// Handle errors if any occurred during fetching
});
You can also use (JavaScript) JS Fetch API:
This fetchpriority
Attribute extends beyond HTML; it can also be used within JS fetch to give priority to specific REST API calls over others.
The (JavaScript) JS Fetch API is used to make network API requests (such as fetching resources or data) asynchronously.
// API endpoint you want to fetch data from
const apiUrl = 'https://api.example.com/data';
// Fetch data using the JS Fetch API
fetch(apiUrl)
.then(response => {
// Check if the response status is OK (200)
if (!response.ok) {
throw new Error(`Network response was not OK: ${response.status}`);
}
// Parse the JSON response
return response.json();
})
.then(data => {
// Handle the data retrieved from the API
console.log('Data received:', data);
// Perform operations with the received data
})
.catch(error => {
// Handle any errors that occurred during the JS fetch
console.error('There was a problem with the fetch operation:', error);
});
The JS Fetch API can be used for various HTTP methods (GET, POST, PUT, DELETE, etc.) and supports more advanced configurations for headers, request bodies, and handling different types of responses.
When to Use Fetch Priority?
Website developers can modify the loading priorities of different resources for an efficient web page loading experience individually to meet the specific needs of the project and user interaction patterns.
- Prioritize Hero Images: Hero images especially one with large size and prominent visuals in the viewport might start with a lower priority by default. Setting a "High"
fetchpriority
for these priority images in the markup can speed up their loading. - Preloading Images with fetchpriority: Use the
preload
attribute along withfetchpriority
for prioritization images referenced in CSS backgrounds or other elements. Without specifying thefetchpriority
, these preloaded images might start with a default "Low" or "Medium" priority.
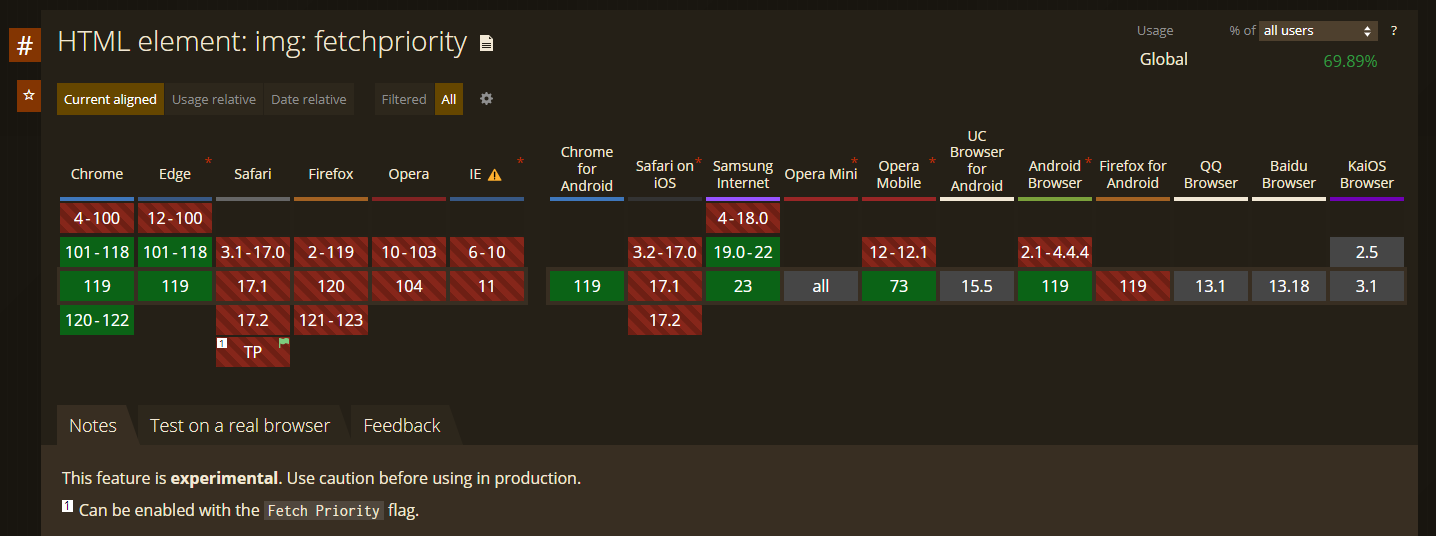
- Script Loading with fetchpriority: Scripts set as async or defer usually have a default "Low" priority. If some scripts are important as per Google for the user experience but load asynchronously, you can focus on their priority using
fetchpriority
without blocking page rendering.
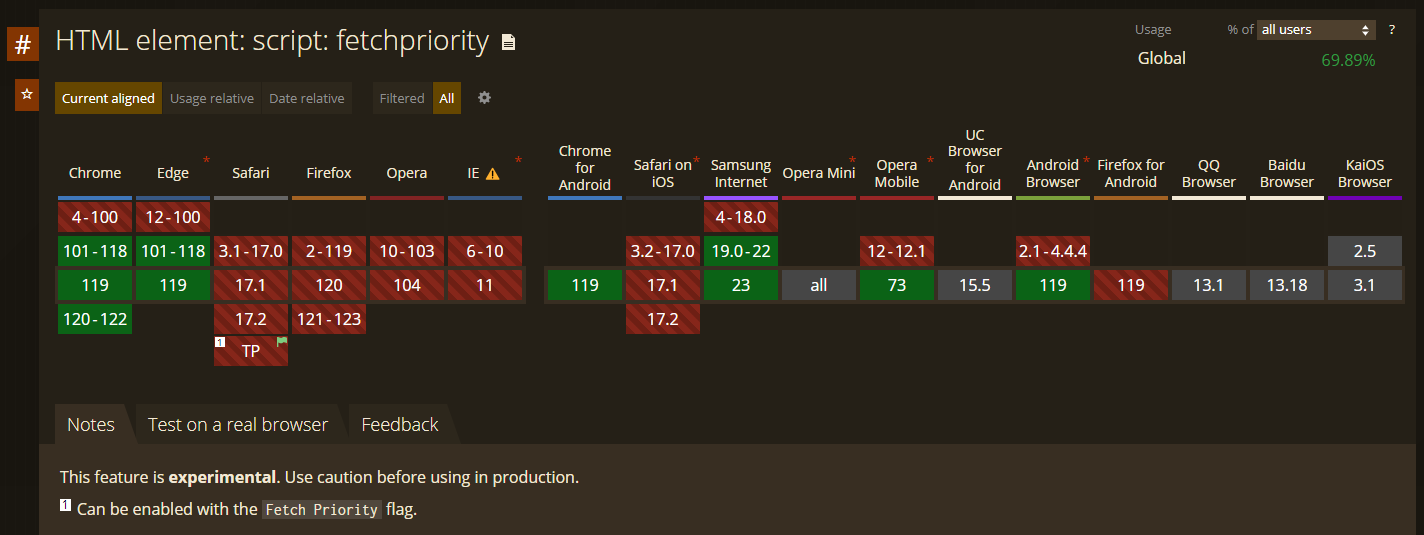
- Adjusting fetchpriority for API Calls: By default, JavaScript's
fetch()
for API calls uses a "High" priority. To manage different types of API calls (background tasks vs. interactive tasks), you can use varyingfetchpriority
levels. For example, tag background API calls as "Low" priority and interactive ones as "High" priority based on how importance they are and their timings.
- Conditional fetchpriority for Images: Images above-the-fold initially visible without scrolling whereas in carousels or sliders they might have different levels of importance which developer can use higher priority to the first visible priority image as it's immediately seen by users. For subsequent images, can also lower priority as they aren't immediately visible.
- Customizing CSS and Fonts with fetchpriority: While browsers assign a default "High" priority to CSS and fonts, not all of these resources will be equally important for the Largest Contentful Paint (LCP).
Browser Compatibility of Fetchpriority
Fetch priority attributes might not be universally supported across all browsers. The effectiveness of using these attributes could be constrained by browser compatibility issues. Some older browsers or specific versions might not recognize or prioritize fetchpriority
attributes correctly.
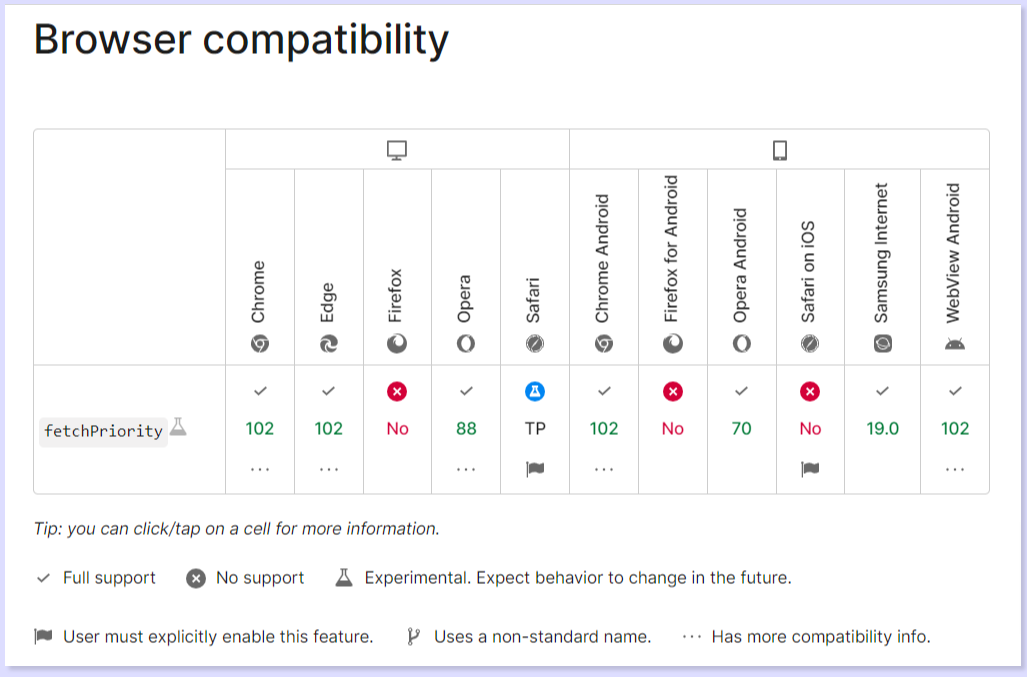
Understand Exactly How Speed of Your Website Affecting Users
ReplayBird, a digital user experience analytics platform designed specifically for website developers with advanced insights to optimize your website speed like a pro!
Unleash the power of behavioral insights with ReplayBird's intuitive heatmaps, session replays, and clickstream analysis, which allows you to visualize user behavior, identify popular elements, and detect pain points that might hinder user satisfaction.
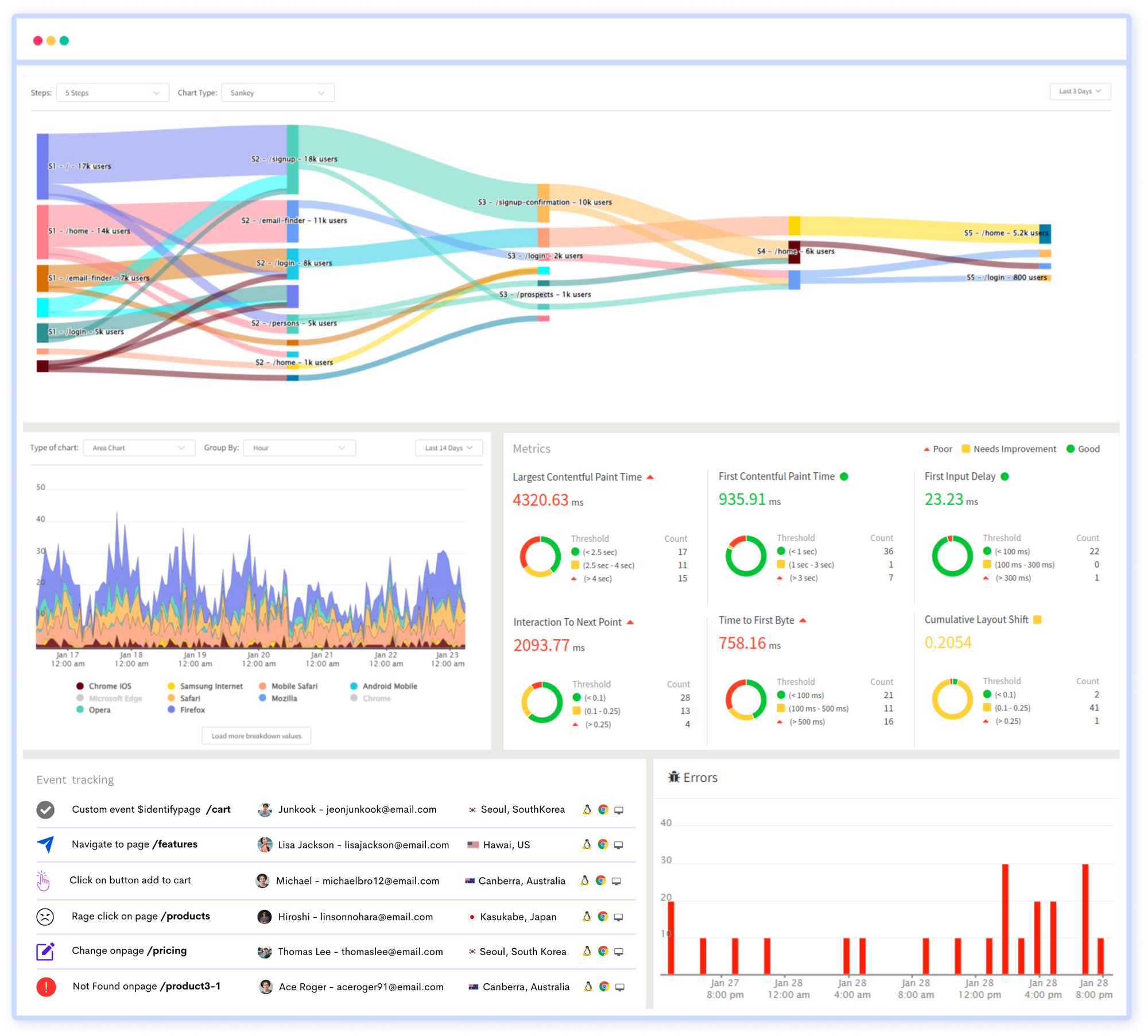
Analyze your website's speed with real user insights. ReplayBird. Prevent load time issues affecting SEO rankings.
Troubleshooting is now simpler with ReplayBird's robust debugging features. Detect and diagnose UX issues quickly, ensuring a seamless user journey from start to finish.
With ReplayBird, you have the ultimate toolkit to elevate your websites to the next level. The platform empowers you to create high-performing, user-centric applications that leave a lasting impression.
Try ReplayBird 14-days free trial
Keep Reading More:
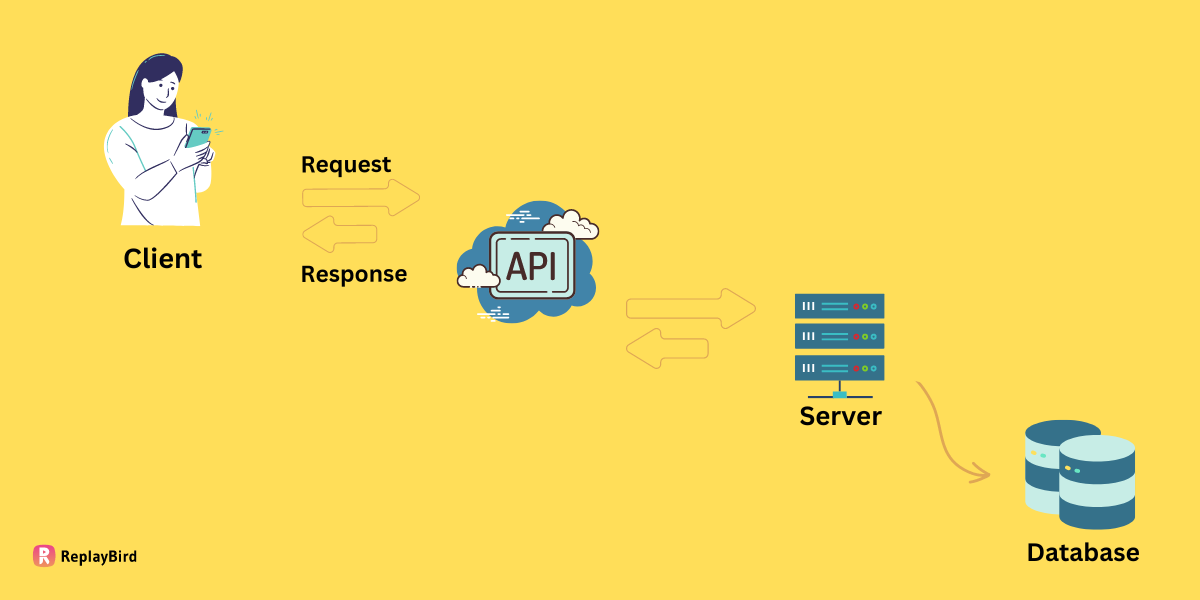
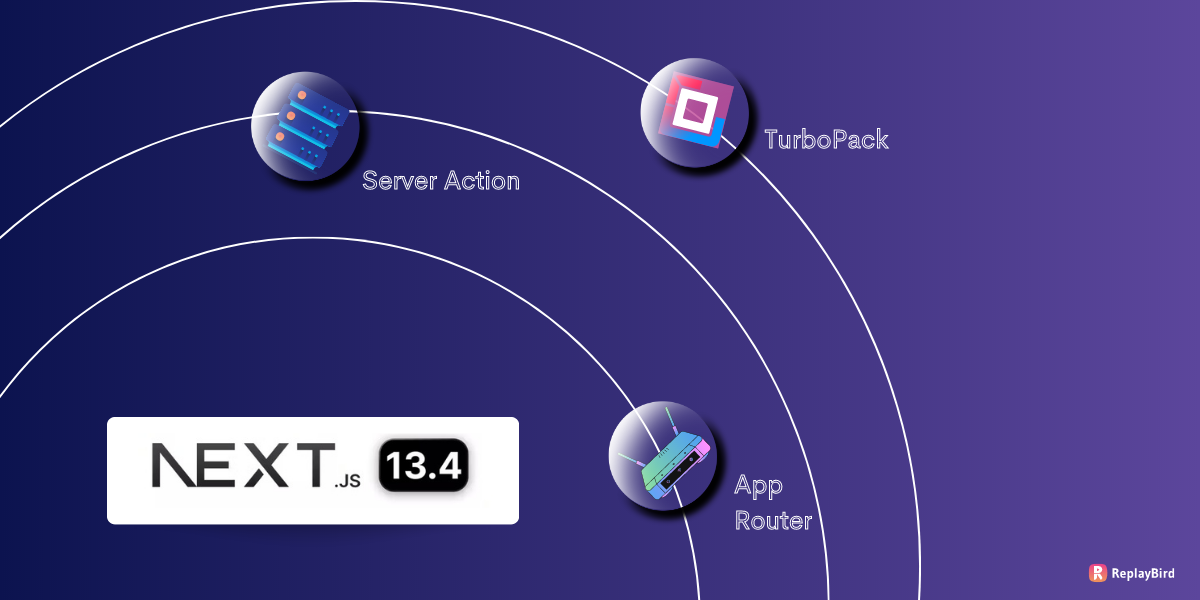
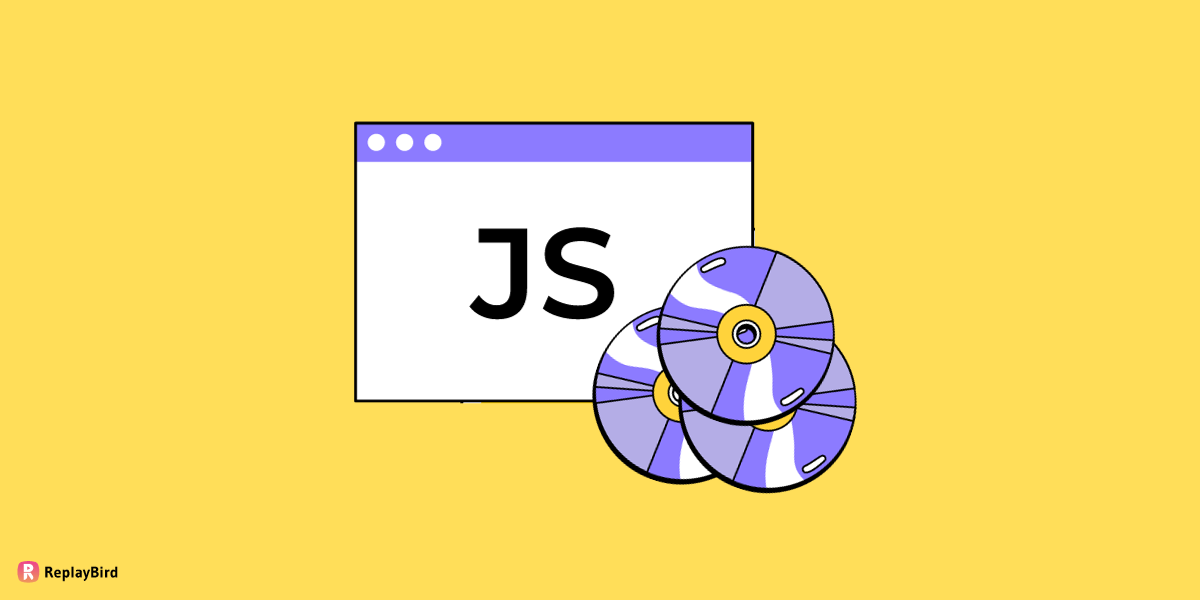