The world of coding and programming can be daunting and a bit confusing, particularly when it comes to understanding the nuance between statements and expressions. It’s an area that can be difficult to grasp and often leads to confusion, especially for beginner coders. However, it is a necessary concept to understand in order to ensure your coding is accurate and efficient.
In this blog post, we will explore the fundamental differences between statements and expressions and unpack the intricacies of each. We will discuss the various uses of statements and expressions, the implications of each and provide examples to help you better understand the concept.
The goal is to provide you with a comprehensive guide to this often complicated topic, so you can be well-armed with the knowledge to confidently navigate the coding world.
- What are Statements and Expressions?
- What's the difference between Statements and Expressions?
- Expressions
- Statements
- Expression including a function call
- Use cases for expressions in programming
- Can expressions be used as statements in programming?
- Can an expression be a single value in programming?
What are Statements and Expressions?
Before getting into the details of the difference between statements and expressions, let us first understand their meanings.
Firstly, the term "Statement" in a programming language refers to a piece of code that communicates instructions to the computer. It is a statement given by the programmer to the computer that defines the action to be carried out. The statements are in accordance with the programming language being used, and they carrier internal components such as expressions. It could be a single statement or a sequence of statements.
Secondly, the term "Expression" refers to a value that constitutes variables, functions, etc. An expression evaluates an output, and the common example could be a mathematical operation.
For instance, 5-2
is arithmetic as well as a programming expression which could yield an output of 3
. Here, the variables are 5
and 2
, and the operator used is the subtraction symbol -
.
What's the difference between Statements and Expressions?
So, let us see the difference between statement and expression below:
Expressions | Statements |
---|---|
Expressions can be assigned. | Statements are executed. |
Values are assigned in an expression. | Statements carry instructions that are to be executed. |
Expression creates value. | Statements take the chance of execution i.e., the statements could be true or false, and it might get executed with side effects. |
Expressions are a part of it, and a group of expressions forms a statement. | Statements comprise a defined structure . |
Expressions have a specific meaning attached to them which gives a definite value. | Statements could be executed or not based on their relativity and precision in coding. |
Expressions
An expression in Java is a combination of one or more literals, variables, operators, and method calls that can be assessed to a value. Expressions are used to produce values that can be assigned to variables, passed as arguments to methods, or utilized in control structures.
Expression can be a:
- Variable
- Keyword
- Operators (+,-,*,/)
- Assignment operator (=)
- Operands
- Numbers
- Characters
Here's an example of a simple expression in Java that adds two numbers:
int x = 5;
int y = 10;
int result = x + y;
In this example, x and y are literals, and + is an operator. The expression x + y is evaluated to 15, which is then assigned to the variable result.
An expression might return any kind of value, such as integers, strings, boolean, etc.
Variable Expressions Examples
A variable expression is an expression in programming that evaluates to a value and can be used to manipulate data and store results of computations.
x = 3;
y = x + 3;
In this example, x
is a variable expression that evaluates to the value 8
, and y
is another variable that stores the result of the expression x + 3
, which is 12
.
Keyword Expressions Examples
Keyword expressions are expressions in programming that contain keywords and are used to control the flow of execution and specify the structure of the program.
X = 7;
if (x > 2) {
System.out.println("X is greater than 2");
}
In this example, the keyword expression if
is used to test the value of x
and execute the print statement only if x
is greater than 3
.
Operator Expressions Example
Operator expressions are expressions in programming that use operators to perform operations on values.
x = 8;
y = 8;
if (x = y) {
System.out.println(x is equal to y);
}
In this example, the expression x = y
evaluates to True
, which means that the condition in the if
statement is satisfied, and the print
statement is executed.
Assignment Operator Expression Example
The assignment operator expression is a type of expression in programming that assigns a value to a variable.
x = 4;
y = 7;
z = x + y;
In this example, the expression x + y
evaluates to 11
, and the assignment operator expression z = x + y
assigns the value 11
to the variable z
.
Operands Expressions Examples
The operands are the objects that the operations are performed on.
8 * (4 + 4)
In this expression, 8
and (4 + 4)
are the operands and * is the operator. The expression inside the parentheses, (4 + 4)
, is evaluated first, and then the result, 8
, is multiplied by it. So, the expression evaluates to 8 * 8
, which is equal to 64
.
Numerical Expressions Examples
A numerical expression refers to an expression that evaluates to a numerical value, such as an integer or a floating-point number.
6 + 4 * 7
In this expression, the operands are 6
, 4
, and 7
, and the operators are +
and *
. According to the rules of operator precedence, the multiplication 4 * 7
is performed first, yielding 28
, and then the addition 6 + 28
is performed, yielding 168
. So, the expression evaluates to 168
, which is a numerical value.
Character Expressions Examples
The character expression evaluates to a character value, represented as a single 16-bit Unicode character. In Java, character expressions are written by enclosing a single character in single quotes, as shown below:
char a = 'a';
char b = 'b';
You may also use the (int) typecast operator to utilise a character's integer value in a character expression. As an example:
char c = (char) 65; // c will have the value 'A'
Using parentheses in expressions
If the operators used in the arithmetic expressions are independent of each other, the order of expression need not be focused on. This is because the output would be the same in spite of the order of expressions. Let us understand this with an example:
2x5x10
Here, the order of precedence is not important since multiplication is the only operator being used and the output would remain the same even if you change the order.
However, the use of parentheses comes in to play when multiple operators are being used and the order of precedence needs to be determined.
Parentheses are an essential element in a compound expression. The output might change according to the use of parentheses. We'll walk through an example to understand this better.
2+2/2
This expression is indicated without parentheses and the output for it would be 3. Since division comes first in the order of precedence, 2 would get divided by 2 first and it would result to 1. Then it would be added again to 2 producing the final output as 3. Whereas in case parentheses is used in the same statement above, it could make a difference with the way you're using it.
For instance,
2+(2/2)
would produce the same output of 3.
However, if parentheses is used in a different way, let's say, (2+2)/2 the output would be 2.
This is because the values in parentheses get processed first. And that is why it is important to specify parentheses in arithmetic expressions in order to avoid errors in output. If you do not indicate the operator precedence using parentheses, the standard order of precedence is followed:
-
Postfix operators:
- expr++
- expr--
-
Unary operators:
- ++expr
- --expr
- +expr
- -expr
- ~
- !
-
Multiplicative:
- /
- %
-
Additive:
- +
- -
-
Shift:
- <<
- >>
- >>>
-
Relational:
- <
- >
- <=
- >=
- instanceof
-
Equality:
- ==
- !=
-
Bitwise:
- AND - &
-
Bitwise exclusive:
- OR - ^
-
Bitwise inclusive:
- OR - |
-
Logical:
- AND - &&
- OR - ||
-
Conditional:
- ?
- :
-
Assignment:
- =
- +=
- -=
- *=
- /=
- %=
- &=
- ^=
- |=
- <<=
- >>=
- >>>=
Statements
Now that we know a group of expressions forms a statement let us look more at it in detail. Moreover, statements either perform the action or do not perform it. Let us look at an example of a statement.
const mrp = 300;
This is a statement that comprises values, and it performs the action of assigning 300 to the variable of 'mrp'.
There are many types of statements in programming languages. They are as follows:-
a) Declaration statements
It is a standalone statement wherein inline means ' in a line', and the statement is aligned. In simple words, the Statement is in line with a sequence.
For instance,
int x = 10;
int y = 20;
prints(10,20);
b) Conditional statements
These statements are double-sided, wherein they might get executed or not based on relevance or conditions attached to them. Let's see an example.
if (score <= 80) {
grade = 'A';
}
Here, this statement checks a condition to return a value. As mentioned earlier, only if the condition is satisfied will the statement be executed. Otherwise, the Statement would not be executed.
The statements do not always execute to a value; rather, they might just get executed or not like in the case of loop statements. Loop statements get iterated several times. The three common types of loop statements are:
- for
- while
- do while
#1 for loop:
Here, part of the program gets iterated based on the number of iterations fixed. You need to initialize a variable, check the condition, and increment or decrement the value. The four parts of For loop are:
- Initialization: Here, the variables are initialized. This is the first condition that gets executed.
- Condition: Here, the condition of the loop is set wherein it gets executed repeatedly until the condition becomes false.
- Increment/Decrement: Here, the variable gets incremented or decremented and is an optional condition.
- Statement: It refers to the statement or code to be executed until the condition becomes false.
Syntax in Java programming language is:
for (initialization; condition; increment / decrement) {
//Statement to be executed
}
Example:
for (int i = 1; i & lt; = 10; i++) {
System.out.println(i);
}
The above code will print integer values from 1 to 10.
#2 while loop:
It is a repetitive if statement wherein if the number of iterations is not fixed, you can use the whole loop. To print integer values from 1 to 10, just like we did in for loop, the following code is used in Java:
int i = 1;
while (i <= 10) {
System.out.println(i);
i++;
}
Here, the initialization and increment/decrement of variables are done separately, unlike the for a loop. Hence, the while loop contains the following parts:
- Condition: Here, the condition is tested, and the loop statement is executed until the condition remains true. We exit the while loop when the condition becomes false.
- Update expression: Here, the variable gets incremented or decremented every time the loop statement is executed.
#3 do while loop:
Here, part of the program gets iterated repeatedly until the condition is true. It is otherwise called an exit control loop, and the condition is checked after the loop body is processed. The two parts of do while loop are:
- Condition: It is an expression that is tested, and if it is true, the loop body is executed. Thereafter, it goes for updated expression.
- Update expression: After the condition is tested, the variable is incremented or decremented based on the specifications.
Syntax in Java:
do {
//code to be executed / loop body
//update Statement
} while (condition);
Example:
To print integer values from 1 to 10, just like we did in the previous loop, the following code is used:
int i = 1;
do {
System.out.println(i);
i++;
} while (i <= 10);
Here, the variable is initialized separately, and the update expression is specified separately failing which the loop will get executed infinitely.
Expression including a function call
In programming, an expression that includes a function call is a type of expression that evaluates to a value by calling a function. The function call is part of the expression and its value is returned as the result of evaluating the expression. The syntax for a function call as an expression varies depending on the programming language, but it generally involves the name of the function, followed by a set of parentheses enclosing any arguments that the function requires.
For example, in java, the following expression is a function call that evaluates to the value returned by the function max:
max(1, 2, 3)
In this example, the max function is called with three arguments, and the expression evaluates to the maximum value of the three arguments, which is 3.
Function calls can be used in a variety of ways within expressions, such as to perform calculations, manipulate data, or control program flow. The value returned by a function can be used as part of a larger expression, or it can be assigned to a variable for use later in the program.
Use cases for expressions in programming
Expressions are a fundamental concept in programming and are used in many different ways. Some common use cases for expressions include:
- Assigning values to variables: Expressions can be used to compute a value and assign it to a variable. For example,
x = 2 + 3
is an expression that evaluates to 5 and assigns the value 5 to the variable x. - Performing calculations: Expressions can be used to perform arithmetic operations, such as addition, subtraction, multiplication, and division. For example,
2 + 3 * 4
is an expression that evaluates to 14. - Evaluating conditions: Expressions can be used to evaluate conditions and make decisions in a program. For example,
x > 0
is an expression that evaluates to either true or false, depending on the value of x. - Initializing data structures: Expressions can be used to initialize arrays, lists, dictionaries, and other data structures. For example,
[1, 2, 3]
is an expression that creates an array with three elements. - Calling functions: Expressions can include function calls, which allow you to reuse code and perform complex operations. For example,
max(2, 3)
is an expression that calls the max function with two arguments and returns the maximum value. - Formatting strings: Expressions can be used to format strings by embedding values within a string literal. For example, "The answer is" +
str(2 + 3)
is an expression that evaluates to the string "The answer is 5".
These are just a few examples of the many ways that expressions are used in programming. Expressions provide a flexible and powerful way to manipulate data, make decisions, and perform operations in a program.
Can expressions be used as statements in programming?
Yes, expressions can be used as statements in programming. In many programming languages, an expression can be used as a statement if it has a side effect, such as assigning a value to a variable, performing a calculation, or calling a function. The result of the expression is not used, but the side effect is executed when the statement is executed.
Can an expression be a single value in programming?
Yes, an expression can be a single value in programming. An expression is any valid combination of symbols that can be evaluated to produce a value. This value can be a constant, a variable, or a combination of both. Expressions can be simple or complex, and the result of evaluating an expression is a single value, which can be a scalar value (such as an integer or floating-point number) or a compound value (such as an array or object).
Conclusion:
We looked at the differences between expression and Statement in this article. Moreover, the concept of expressions and statements were discussed in brief.
Understanding these differences contributes to a better understanding of the programming languages. In addition, this key knowledge adds value to the know-how of practical coding in various languages.
A statement is a standalone unit of code that performs an action. Statements don't return a value, but they can have side effects, such as modifying a variable or performing an I/O operation. Examples of statements include assignments, control structures (such as if statements and loops), and function calls.
An expression, on the other hand, is a piece of code that evaluates to a value. Expressions can be simple, like a constant or a variable, or complex, like a combination of operators and function calls. Expressions can be used as part of larger expressions, or they can be assigned to variables.
In conclusion, a good insight into these basic concepts of programming language helps prevent errors and enhances your coding ability.
ReplayBird - Driving Revenue and Growth through Actionable Product Insights
ReplayBird is a digital experience analytics platform that offers a comprehensive real-time insights which goes beyond the limitations of traditional web analytics with features such as product analytics, session replay, error analysis, funnel, and path analysis.
With Replaybird, you can capture a complete picture of user behavior, understand their pain points, and improve the overall end-user experience. Session replay feature allows you to watch user sessions in real-time, so you can understand their actions, identify issues and quickly take corrective actions. Error analysis feature helps you identify and resolve javascript errors as they occur, minimizing the negative impact on user experience.
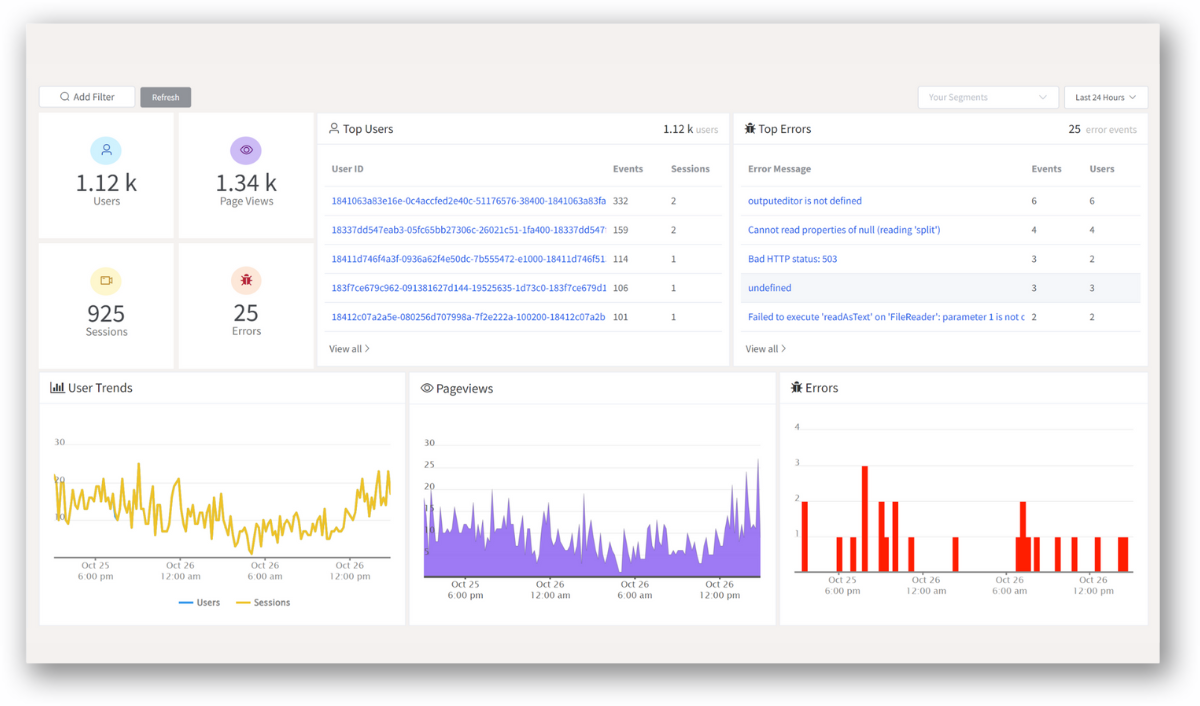
With product analytics feature, you can get deeper insights into how users are interacting with your product and identify opportunities to improve. Drive understanding, action, and trust, leading to improved customer experiences and driving business revenue growth.
Try ReplayBird 14-days free trial
Further Readings
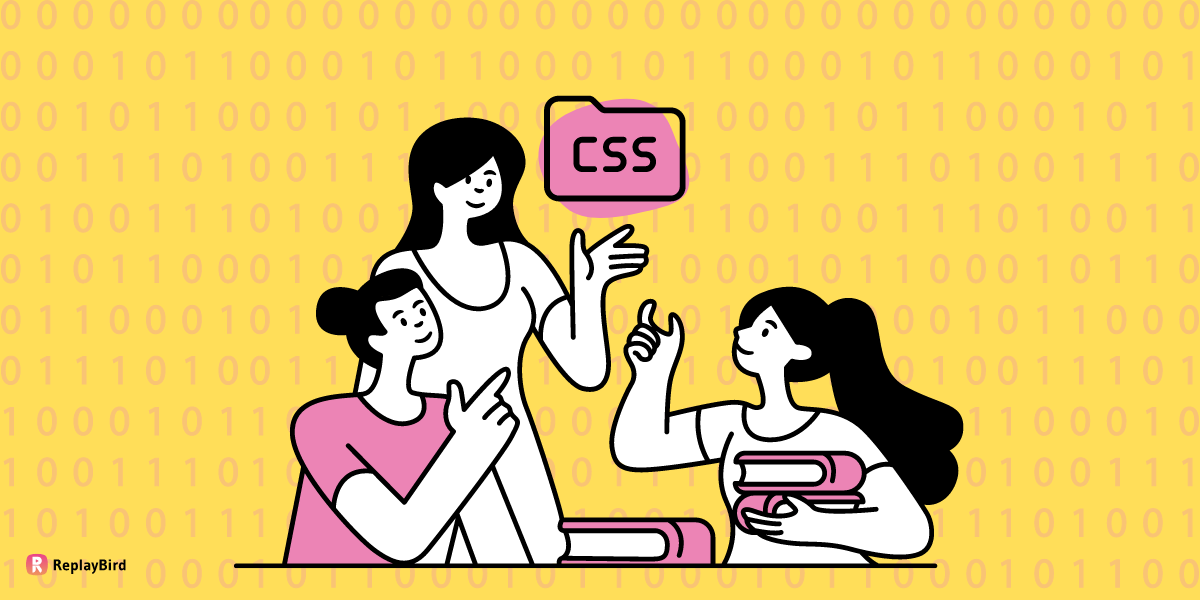
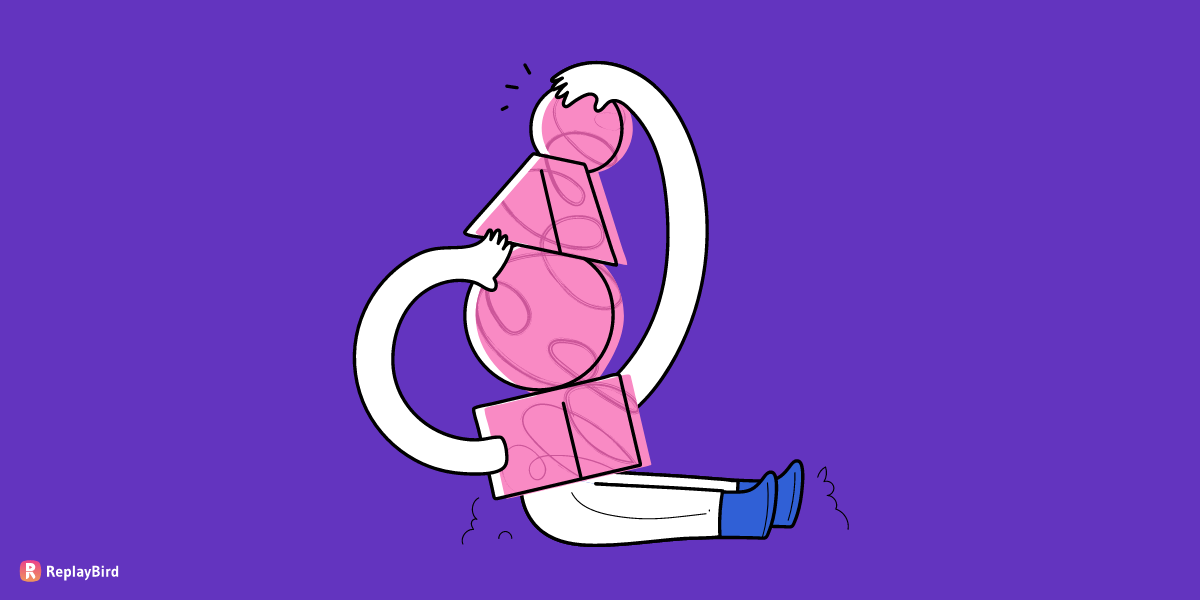
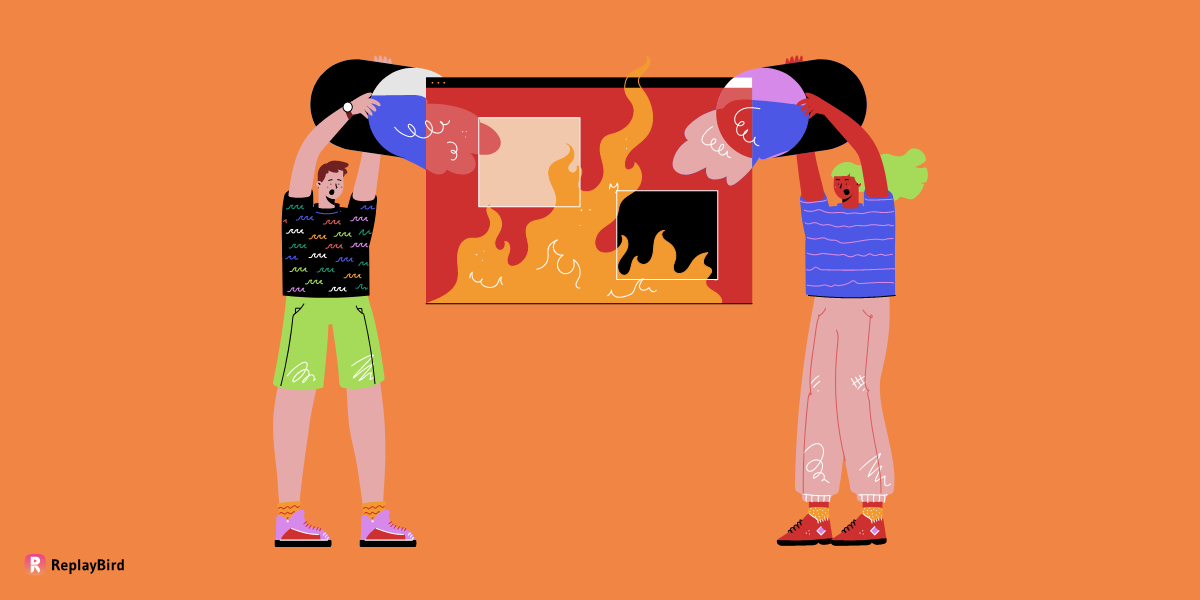