Python Dictionaries are a significant data structure in Python that permit storing and retrieving data through key-value pairs. Other programming languages may refer to them as associative arrays, maps, or hash tables.
It's importance in Python programming is due to their efficiency in storing, modifying, and retrieving data with a unique identifier (key).
Dictionaries are flexible and can represent complex data structures like graphs and trees, and they are useful in various domains like data science, web development, and machine learning.
In Python, dictionaries are mutable, so you can modify them anytime by adding, updating, or removing key-value pairs they also support various built-in methods that make it easy to manipulate and query the data they contain.
Now Let's look into the topics that we will cover in this Python dictionaries blog:
- Creating and Initializing Python Dictionaries
- Accessing Values in Python Dictionaries
- Updating Python Dictionaries
- Adding and Removing Key-Value Pairs
- Iterating Over Python Dictionaries
- Python Dictionary Methods
- Python Dictionary Comprehension
Creating and Initializing Python Dictionaries
Let us first look at how to create an empty dictionary and add key-value pairs to it.
To create an empty dictionary in Python, we will now use curly braces and the dict()
function.
python_dict = {}
# or
python_dict = dict()
Both of these methods will create an empty dictionary in your Python.
To add key-value pairs to your Python dictionary, you can use assignment.
python_dict['key1'] = 'value1'
python_dict['key2'] = 'value2'
python_dict['key3'] = 'value3'
This adds three key-value pairs to the python_dict
dictionary, whereas you can add as many key-value pairs as you need in the same way.
Alternatively, you can also create a Python dictionary with some initial key-value pairs using curly braces.
python_dict = {'key1': 'value1', 'key2': 'value2', 'key3': 'value3'}
This creates a dictionary with three key-value pairs.
Remember that keys in the Python dictionary must be unique and immutable (they cannot be changed). Values can be any type, including other dictionaries or objects. Let's give keys as employees and their value as their rankings as an employee.
#1 Using curly braces:
python_dict = {'Liam': 3, 'Harry': 6, 'Niall': 4}
print(python_dict)
Output:
{'Liam': 3, 'Harry': 6, 'Niall': 4}
#2 Using the dict()
function: key-value pairs
python_dict = dict(Liam=3, Harry=6, Niall=4)
print(python_dict)
Output:
{'Liam': 3, 'Harry': 6, 'Niall': 4}
Note that when using the dict()
function, the keys are specified as keyword arguments without quotes around them. This is a shorthand syntax that can be more convenient for some use cases.
You can also mix and match the two syntaxes to create a Python dictionary.
python_dict = {'Liam': 3, 'Harry': 6}
python_dict.update(dict(Niall=4, select=2))
print(python_dict)
Output:
{'Liam': 3, 'Harry': 6, 'Niall': 4, 'select': 2}
Here, we first create a dictionary with two key-value pairs using curly braces. Then, we use the update()
method to add two more key-value pairs using the dict()
function.
Accessing Values in Python Dictionaries
In Python dictionaries, you can access values using keys. To do this, you can use square brackets []
and specify the key inside the brackets.
python_dict = {'Liam': 3, 'Harry': 6, 'Niall': 4}
#Access the value associated with the 'Harry' key
print(python_dict['Harry'])
Output:
6
# Access the value associated with the 'Niall' key
print(python_dict['Niall'])
Output:
4
In this example, we create a dictionary python_dict
that contains three key-value pairs. To access the value associated with a particular key, we use square brackets and specify the key inside them.
If the key you specify is not present in the dictionary, a KeyError exception will be raised. To avoid this, you can use the get()
method, which returns None if the key is not present, or a default value that you specify:
# Access the value associated with the 'Louis' key (not present)
print(python_dict.get('Louis'))
Output:
None
# Access the value associated with the 'Louis' key, with a default value of 0
print(python_dict.get('Louis', 0))
Output:
0
We try to access the value associated with the 'Louis'
key, which is not present in the dictionary. Using get()
with no default value specified returns None. We can specify a default value of 0
by passing it as the second argument to get()
.
Both methods achieve the same result of retrieving a value associated with a key, but there are some differences between them:
- Error handling: When using square brackets to access a non-existent key, a
KeyError
exception is raised. However, theget()
method can return a default value when a key is not found instead of raising an error. - Default value: The square bracket method doesn't have a way to specify a default value to be returned if the key is not found. The
get()
method, on the other hand, takes an optional second argument that specifies the default value to be returned if the key is not found.
Shall we look at the differences between using square brackets []
and the get()
method:
python_dict = {'Liam': 3, 'Harry': 6, 'Niall': 4}
# Using square brackets to access a key that doesn't exist raises a KeyError
print(python_dict['Louis'])
# Using get() with a non-existent key returns None by default
print(python_dict.get('Louis'))
# Using get() with a non-existent key returns a default value if specified
print(python_dict.get('Louis', 0))
# Using square brackets to access an existing key
print(python_dict['Liam'])
# Using get() to access an existing key
print(python_dict.get('Liam'))
Output:
KeyError: 'Louis'
None
0
3
3
We have created a dictionary python_dict
that contains three key-value pairs. We have tried to access a non-existent key 'Louis'
using square brackets []
, which raises a KeyError
. However, using the get()
method with the same key returns None
. When a default value of 0
is specified with get()
, it returns 0
instead of None
. When accessing an existing key, both methods return the same value.
Updating Python Dictionaries
Python dictionaries are mutable, which means that you can update them by adding, modifying, or removing key-value pairs.
To update an existing dictionary, you can assign a new value to a key or use the update()
method to add, modify or remove key-value pairs from the dictionary.
python_dict = {'Liam': 3, 'Harry': 6, 'Louis': 4}
# Updating an existing key
python_dict['Liam'] = 5
# Adding a new key-value pair
python_dict['Zayn'] = 2
# Removing a key-value pair
del python_dict['Louis']
# Using the update() method to add or modify key-value pairs
python_dict.update({'Liam': 10, 'Niall': 8})
print(python_dict)
Output:
{'Liam': 10, 'Harry': 6, 'Zayn': 2, 'Niall': 8}
We have created a dictionary python_dict
that contains three key-value pairs. We have updated the value associated with the key 'Liam' by assigning a new value to it. We have added a new key-value pair 'switch': 2 to the dictionary using the square bracket notation. We also have removed the key-value pair 'swipe': 4 from the dictionary using the del
statement. Finally, we use the update()
method to add a new key-value pair 'Niall': 8
and modify the value associated with the key 'Liam' to 10
.
Note that when you add a key-value pair using
update()
, it will overwrite the value of an existing key if the key already exists in the dictionary. If the key doesn't exist, it will be added as a new key-value pair.
Adding and Removing Key-Value Pairs
#1 Adding new key-value pairs using assignment:
We can add new key-value pairs to the Python dictionary using assignment by assigning a value to a new key that does not already exist in the dictionary.
python_dict = {'Liam': 3, 'Harry': 6, 'Zayn': 4}
# Adding a new key-value pair
python_dict['Niall'] = 2
print(python_dict)
Output:
{'Liam': 3, 'Harry': 6, 'Zayn': 4, 'Niall': 2}
We have first created a python dictionary python_dict
that contains three key-value pairs, and then we have added a new key-value pair 'Niall': 2
to the dictionary by assigning the value 2
to a new key 'Niall'
. When we printed the dictionary, we could see that the new key-value pair had been added to the dictionary.
If you try to add a new key-value pair using an existing key, the existing value for that key will be overwritten with the new value.
python_dict = {'Liam': 3, 'Harry': 6, 'Zayn': 4}
# Overwrite an existing key-value pair
python_dict['Liam'] = 5
print(python_dict)
Output:
{'Liam': 5, 'Harry': 6, 'Zayn': 4}
Now we have overwritten the value associated with the existing key 'Liam'
by assigning the value 5 to it. When we have printed the dictionary, we can see that the value associated with the key 'Liam'
has been updated to 5
.
If you try to add a new key-value pair using a non-existent key that is not present in the Python dictionary, the Python dictionary will create a new key with the assigned value.
python_dict = {'Liam': 3, 'Harry': 6, 'Niall': 4}
# Add a new key-value pair using a non-existent key
python_dict['Zayn'] = 2
print(python_dict)
# Output: {'Liam': 3, 'Harry': 6, 'Niall': 4, 'Zayn': 2}
# Access the value of a non-existent key
print(python_dict['Justin'])
Output:
KeyError: 'Justin'
In this example, we have now tried to access the value of a non-existent key 'Justin'
and received a KeyError
because the key does not exist in the Python dictionary. If you want to add a key-value pair using a non-existent key and provide a default value if the key doesn't exist, you can use the get()
method with a default value.
python_dict = {'Liam': 3, 'Harry': 6, 'Niall': 4}
# Add a new key-value pair using a non-existent key and a default value
python_dict.setdefault('Justin', 2)
# Add a new key-value pair using an existing key and a default value
python_dict.setdefault('Liam', 5)
print(python_dict)
Output:
{'Liam': 3, 'Harry': 6, 'Niall': 4, 'Justin': 2}
In this last example, we have used the setdefault()
method to add a new key-value pair 'Justin': 2
to the dictionary using a non-existent key 'Justin'
and a default value of 2
. We also have tried to add a new key-value pair 'Liam': 5
using an existing key'
#2 Removing key-value pairs using the del
statement.
In a Python dictionary, the del
statement can be used to remove key-value pairs from a dictionary. It takes the key of the item that needs to be removed as its argument.
Here is an example of how to use del
to remove an item from a dictionary using an existing key:
python_dict = {'Liam': 1, 'Harry': 2, 'Louis': 3}
del python_dict['Liam']
print(python_dict)
Output:
{'Harry': 2, 'Louis': 3}
We have used the del
statement to remove the key-value pair with the key 'Liam'
from the dictionary python_dict
.
If the key is not present in the dictionary, a KeyError
will be raised.
python_dict = {'Liam': 1, 'Harry': 2, 'Louis': 3}
del python_dict['Niall']
Output:
Raises KeyError: 'Niall'
The del
statement has tried to remove a key-value pair with the key 'Niall'
which is not present in the dictionary, so it raises a KeyError
.
Iterating Over Python Dictionaries
In Python, iteration means repeating a block of code for every item in a collection, such as a list, tuple, or dictionary. When you iterate over a collection, you can perform different operations on each item, such as modifying it, printing it, or using it to calculate a result.
When you iterate over a dictionary in Python, you are essentially looping through all the keys, values, or key-value pairs in the dictionary. Since dictionaries are unordered collections, you cannot rely on the order of the keys or key-value pairs while iterating. However, iterating over a dictionary can still be useful if you need to perform an operation on every key, value, or key-value pair, regardless of their order.
Iterating over dictionaries is beneficial in various programming situations. For instance, if you have data stored in a dictionary, you may need to loop through each key or value and perform some action on it. Similarly, if you are looking for a particular value in a dictionary, you can iterate over the keys or key-value pairs until you locate the value you need.
In Python, we can use loops to iterate over dictionaries. There are three primary methods for iterating over dictionaries: keys()
, values()
, and items()
.
#1 Iterating Over Python Dictionaries Using keys()
python_dict = {'Liam': 1, 'Harry': 2, 'Niall': 3}
# Iterate over keys
for key in python_dict.keys():
print(key)
Output:
Liam
Harry
Niall
#2 Iterating Over Python Dictionaries Using values()
python_dict = {'Liam': 1, 'Harry': 2, 'Niall': 3}
# Iterate over values
for value in python_dict.values():
print(value)
Output:
1
2
3
#3 Iterating Over Python Dictionaries Using items()
python_dict = {'Liam': 1, 'Harry': 2, 'Niall': 3}
# Iterate over key-value pairs
for key, value in python_dict.items():
print(key, value)
Output:
Liam 1
Harry 2
Niall 3
The keys()
method returns a list of all the keys in the dictionary, which can be used to iterate over the keys. The values()
method returns a list of all the values in the dictionary, which can be used to iterate over the values. The items()
method returns a list of tuples containing the key-value pairs in the dictionary, which can be used to iterate over both the keys and the values at the same time.
When using the items()
method, the items are returned as tuples, with the first element is the key and the second element being the value. This allows you to access both the key and the value in the same loop.
In general, the items()
method is the most versatile because it allows you to access both the keys and the values at the same time. However, if you only need to iterate over the keys or the values, using the keys()
or values()
method can be more efficient.
Python Dictionary Methods
#1 keys()
This method returns a view object containing all the keys in the dictionary.
python_dict = {'Liam': 1, 'Harry': 2, 'Niall': 3}
print(python_dict.keys())
Output:
dict_keys(['Liam', 'Harry', 'Niall'])
#2 values()
This method returns a view object containing all the values in the dictionary.
python_dict = {'Liam': 1, 'Harry': 2, 'Niall': 3}
print(python_dict.values())
Output:
dict_values([1, 2, 3])
#3 items()
This method returns a view object containing all the key-value pairs in the dictionary as tuples.
python_dict = {'Liam': 1, 'Harry': 2, 'Niall': 3}
print(python_dict.items())
Output:
dict_items([('Liam', 1), ('Harry', 2), ('Niall', 3)])
#4 get()
This method returns the value associated with the given key in the dictionary. If the key is not found, it returns a default value (None if not specified).
python_dict = {'Liam': 1, 'Harry': 2, 'Niall': 3}
print(python_dict.get('Liam')
print(python_dict.get('Louis', 4))
Output:
1
4 (default value)
#5 pop()
This method removes and returns the value associated with the given key from the dictionary. If the key is not found, it returns a default value (Raises KeyError if not specified).
python_dict = {'Liam': 1, 'Harry': 2, 'Niall': 3}
value = python_dict.pop('harry')
print(value)
print(python_dict)
Output:
2
{'Liam': 1, 'Niall': 3}
#6 clear()
This method removes all the key-value pairs from the dictionary.
python_dict = {'Liam': 1, 'Harry': 2, 'Niall': 3}
python_dict.clear()
print(python_dict)
Output:
{}
#7 copy()
This method creates a shallow copy of the dictionary.
python_dict = {'Liam': 1, 'Harry': 2, 'Niall': 3}
new_dict = python_dict.copy()
print(python_dict)
Output:
{'Liam': 1, 'Harry': 2, 'Niall': 3}
Python Dictionary Comprehension
Dictionary comprehension is a concise and efficient way of creating a dictionary in Python by mapping an iterable object to key-value pairs which are similar to comprehension but use curly braces {}
instead of square brackets []
.
Example of creating a dictionary using dictionary comprehension to store the squares of numbers from 1 to 5:
keys = ['a', 'b', 'c', 'd', 'e']
values = [1, 2, 3, 4, 5]
dictionary = {k:v for k, v in zip(keys, values)}
print(dictionary)
Output:
{'a': 1, 'b': 2, 'c': 3, 'd': 4, 'e': 5}
We have created a dictionary from two lists using the built-in zip()
function to combine corresponding elements from the two lists; then, you can use dictionary comprehension to create a new dictionary where the elements from the first list are the keys and the elements from the second list are the values.
Conclusion:
In summary, Python dictionaries are widely used in Python programming due to their versatility and strength as a data structure. They offer a flexible means of storing and retrieving data by using key-value pairs.
Proficiency in creating, manipulating, and iterating dictionaries is vital for any Python programmer. Furthermore, with the inclusion of dictionary comprehension, programmers have access to a powerful tool to create dictionaries concisely and legibly.
Python Dictionaries are efficient in storing and retrieving data with their built-in methods and tools. A thorough understanding of how to use Python dictionaries effectively can enhance programming skills and lead to the development of more efficient and effective programs.
ReplayBird - Driving Revenue and Growth through Actionable Product Insights
ReplayBird is a digital experience analytics platform that offers a comprehensive real-time insights which goes beyond the limitations of traditional web analytics with features such as product analytics, session replay, error analysis, funnel, and path analysis.
With Replaybird, you can capture a complete picture of user behavior, understand their pain points, and improve the overall end-user experience. Session replay feature allows you to watch user sessions in real-time, so you can understand their actions, identify issues and quickly take corrective actions. Error analysis feature helps you identify and resolve javascript errors as they occur, minimizing the negative impact on user experience.
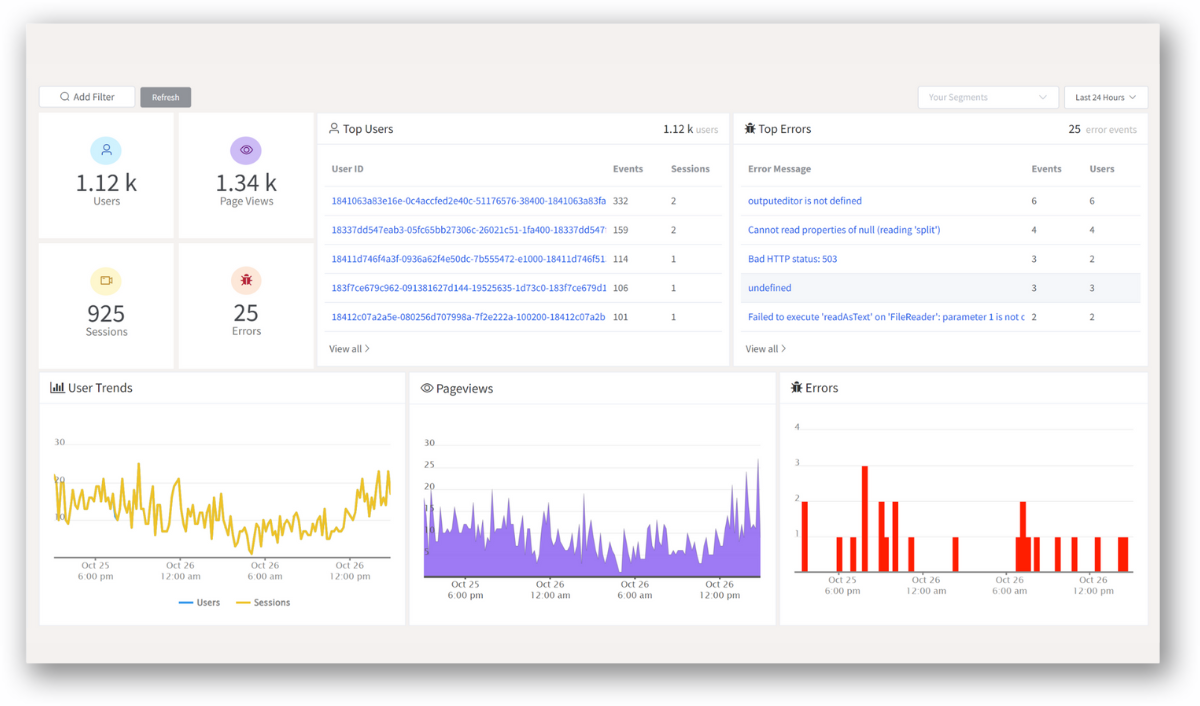
With product analytics feature, you can get deeper insights into how users are interacting with your product and identify opportunities to improve. Drive understanding, action, and trust, leading to improved customer experiences and driving business revenue growth.
Try ReplayBird 14-days free trial
Further Readings:
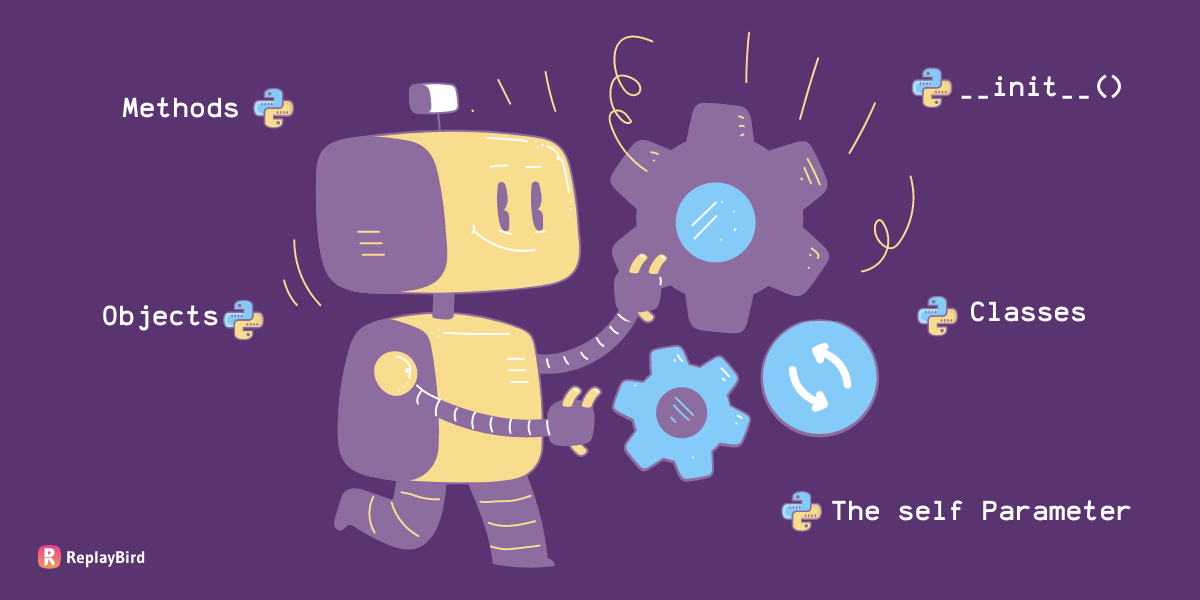
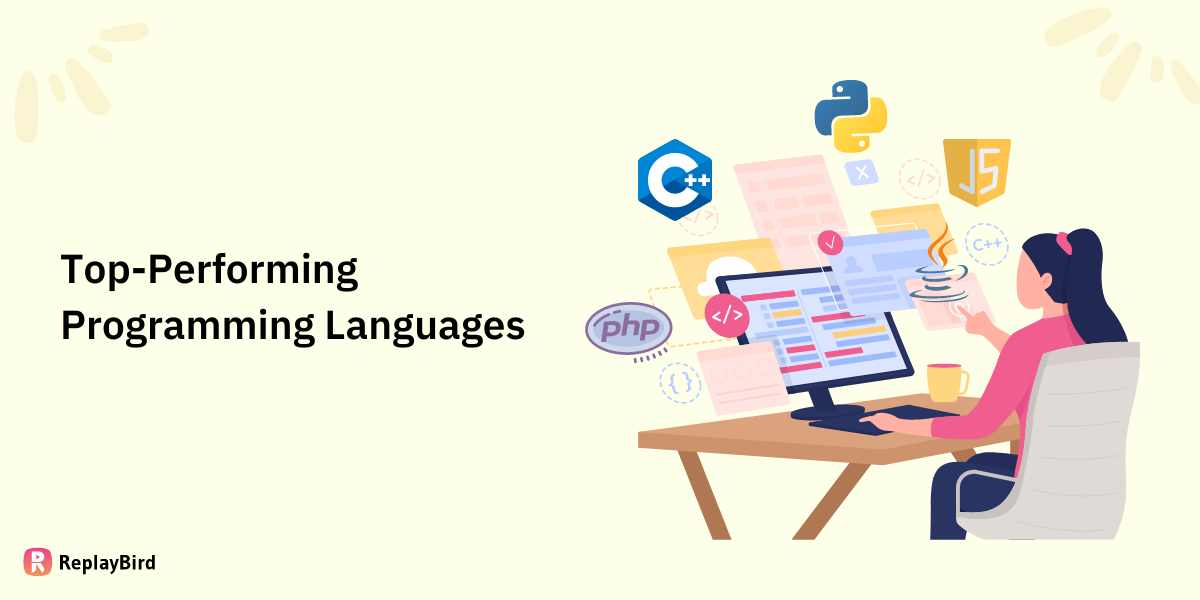
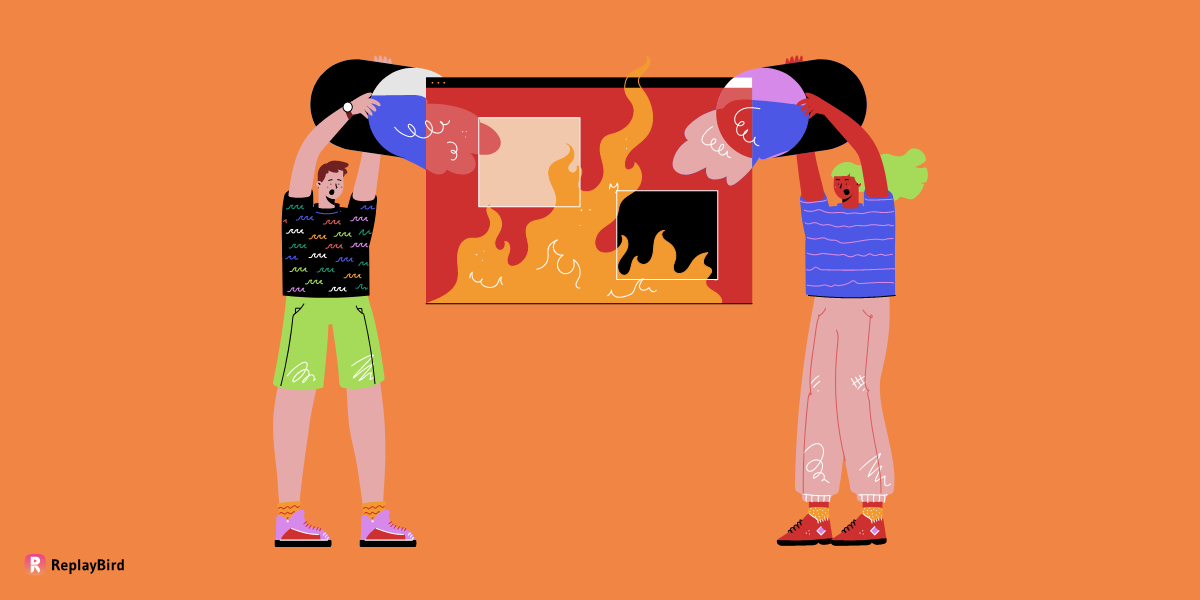